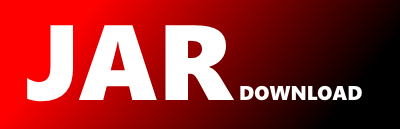
commonMain.aws.sdk.kotlin.services.lookoutmetrics.model.UpdateMetricSetRequest.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lookoutmetrics-jvm Show documentation
Show all versions of lookoutmetrics-jvm Show documentation
The AWS SDK for Kotlin client for LookoutMetrics
The newest version!
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.lookoutmetrics.model
import aws.smithy.kotlin.runtime.SdkDsl
public class UpdateMetricSetRequest private constructor(builder: Builder) {
/**
* Describes a list of filters for choosing specific dimensions and specific values. Each filter consists of the dimension and one of its values that you want to include. When multiple dimensions or values are specified, the dimensions are joined with an AND operation and the values are joined with an OR operation.
*/
public val dimensionFilterList: List? = builder.dimensionFilterList
/**
* The dimension list.
*/
public val dimensionList: List? = builder.dimensionList
/**
* The metric list.
*/
public val metricList: List? = builder.metricList
/**
* The ARN of the dataset to update.
*/
public val metricSetArn: kotlin.String? = builder.metricSetArn
/**
* The dataset's description.
*/
public val metricSetDescription: kotlin.String? = builder.metricSetDescription
/**
* The dataset's interval.
*/
public val metricSetFrequency: aws.sdk.kotlin.services.lookoutmetrics.model.Frequency? = builder.metricSetFrequency
/**
* Contains information about source data used to generate metrics.
*/
public val metricSource: aws.sdk.kotlin.services.lookoutmetrics.model.MetricSource? = builder.metricSource
/**
* After an interval ends, the amount of seconds that the detector waits before importing data. Offset is only supported for S3, Redshift, Athena and datasources.
*/
public val offset: kotlin.Int? = builder.offset
/**
* The timestamp column.
*/
public val timestampColumn: aws.sdk.kotlin.services.lookoutmetrics.model.TimestampColumn? = builder.timestampColumn
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.lookoutmetrics.model.UpdateMetricSetRequest = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("UpdateMetricSetRequest(")
append("dimensionFilterList=$dimensionFilterList,")
append("dimensionList=$dimensionList,")
append("metricList=$metricList,")
append("metricSetArn=$metricSetArn,")
append("metricSetDescription=$metricSetDescription,")
append("metricSetFrequency=$metricSetFrequency,")
append("metricSource=$metricSource,")
append("offset=$offset,")
append("timestampColumn=$timestampColumn")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = dimensionFilterList?.hashCode() ?: 0
result = 31 * result + (dimensionList?.hashCode() ?: 0)
result = 31 * result + (metricList?.hashCode() ?: 0)
result = 31 * result + (metricSetArn?.hashCode() ?: 0)
result = 31 * result + (metricSetDescription?.hashCode() ?: 0)
result = 31 * result + (metricSetFrequency?.hashCode() ?: 0)
result = 31 * result + (metricSource?.hashCode() ?: 0)
result = 31 * result + (offset ?: 0)
result = 31 * result + (timestampColumn?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as UpdateMetricSetRequest
if (dimensionFilterList != other.dimensionFilterList) return false
if (dimensionList != other.dimensionList) return false
if (metricList != other.metricList) return false
if (metricSetArn != other.metricSetArn) return false
if (metricSetDescription != other.metricSetDescription) return false
if (metricSetFrequency != other.metricSetFrequency) return false
if (metricSource != other.metricSource) return false
if (offset != other.offset) return false
if (timestampColumn != other.timestampColumn) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.lookoutmetrics.model.UpdateMetricSetRequest = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* Describes a list of filters for choosing specific dimensions and specific values. Each filter consists of the dimension and one of its values that you want to include. When multiple dimensions or values are specified, the dimensions are joined with an AND operation and the values are joined with an OR operation.
*/
public var dimensionFilterList: List? = null
/**
* The dimension list.
*/
public var dimensionList: List? = null
/**
* The metric list.
*/
public var metricList: List? = null
/**
* The ARN of the dataset to update.
*/
public var metricSetArn: kotlin.String? = null
/**
* The dataset's description.
*/
public var metricSetDescription: kotlin.String? = null
/**
* The dataset's interval.
*/
public var metricSetFrequency: aws.sdk.kotlin.services.lookoutmetrics.model.Frequency? = null
/**
* Contains information about source data used to generate metrics.
*/
public var metricSource: aws.sdk.kotlin.services.lookoutmetrics.model.MetricSource? = null
/**
* After an interval ends, the amount of seconds that the detector waits before importing data. Offset is only supported for S3, Redshift, Athena and datasources.
*/
public var offset: kotlin.Int? = null
/**
* The timestamp column.
*/
public var timestampColumn: aws.sdk.kotlin.services.lookoutmetrics.model.TimestampColumn? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.lookoutmetrics.model.UpdateMetricSetRequest) : this() {
this.dimensionFilterList = x.dimensionFilterList
this.dimensionList = x.dimensionList
this.metricList = x.metricList
this.metricSetArn = x.metricSetArn
this.metricSetDescription = x.metricSetDescription
this.metricSetFrequency = x.metricSetFrequency
this.metricSource = x.metricSource
this.offset = x.offset
this.timestampColumn = x.timestampColumn
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.lookoutmetrics.model.UpdateMetricSetRequest = UpdateMetricSetRequest(this)
/**
* construct an [aws.sdk.kotlin.services.lookoutmetrics.model.MetricSource] inside the given [block]
*/
public fun metricSource(block: aws.sdk.kotlin.services.lookoutmetrics.model.MetricSource.Builder.() -> kotlin.Unit) {
this.metricSource = aws.sdk.kotlin.services.lookoutmetrics.model.MetricSource.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.lookoutmetrics.model.TimestampColumn] inside the given [block]
*/
public fun timestampColumn(block: aws.sdk.kotlin.services.lookoutmetrics.model.TimestampColumn.Builder.() -> kotlin.Unit) {
this.timestampColumn = aws.sdk.kotlin.services.lookoutmetrics.model.TimestampColumn.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy