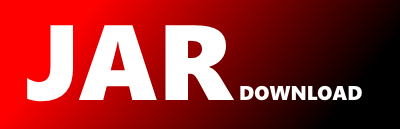
commonMain.aws.sdk.kotlin.services.lookoutmetrics.model.AlertSummary.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lookoutmetrics Show documentation
Show all versions of lookoutmetrics Show documentation
The AWS SDK for Kotlin client for LookoutMetrics
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.lookoutmetrics.model
import aws.smithy.kotlin.runtime.time.Instant
/**
* Provides a summary of an alert's configuration.
*/
public class AlertSummary private constructor(builder: Builder) {
/**
* The ARN of the alert.
*/
public val alertArn: kotlin.String? = builder.alertArn
/**
* The name of the alert.
*/
public val alertName: kotlin.String? = builder.alertName
/**
* The minimum severity for an anomaly to trigger the alert.
*/
public val alertSensitivityThreshold: kotlin.Int = builder.alertSensitivityThreshold
/**
* The status of the alert.
*/
public val alertStatus: aws.sdk.kotlin.services.lookoutmetrics.model.AlertStatus? = builder.alertStatus
/**
* The type of the alert.
*/
public val alertType: aws.sdk.kotlin.services.lookoutmetrics.model.AlertType? = builder.alertType
/**
* The ARN of the detector to which the alert is attached.
*/
public val anomalyDetectorArn: kotlin.String? = builder.anomalyDetectorArn
/**
* The time at which the alert was created.
*/
public val creationTime: aws.smithy.kotlin.runtime.time.Instant? = builder.creationTime
/**
* The time at which the alert was last modified.
*/
public val lastModificationTime: aws.smithy.kotlin.runtime.time.Instant? = builder.lastModificationTime
/**
* The alert's [tags](https://docs.aws.amazon.com/lookoutmetrics/latest/dev/detectors-tags.html).
*/
public val tags: Map? = builder.tags
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.lookoutmetrics.model.AlertSummary = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("AlertSummary(")
append("alertArn=$alertArn,")
append("alertName=$alertName,")
append("alertSensitivityThreshold=$alertSensitivityThreshold,")
append("alertStatus=$alertStatus,")
append("alertType=$alertType,")
append("anomalyDetectorArn=$anomalyDetectorArn,")
append("creationTime=$creationTime,")
append("lastModificationTime=$lastModificationTime,")
append("tags=$tags")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = alertArn?.hashCode() ?: 0
result = 31 * result + (alertName?.hashCode() ?: 0)
result = 31 * result + (alertSensitivityThreshold)
result = 31 * result + (alertStatus?.hashCode() ?: 0)
result = 31 * result + (alertType?.hashCode() ?: 0)
result = 31 * result + (anomalyDetectorArn?.hashCode() ?: 0)
result = 31 * result + (creationTime?.hashCode() ?: 0)
result = 31 * result + (lastModificationTime?.hashCode() ?: 0)
result = 31 * result + (tags?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as AlertSummary
if (alertArn != other.alertArn) return false
if (alertName != other.alertName) return false
if (alertSensitivityThreshold != other.alertSensitivityThreshold) return false
if (alertStatus != other.alertStatus) return false
if (alertType != other.alertType) return false
if (anomalyDetectorArn != other.anomalyDetectorArn) return false
if (creationTime != other.creationTime) return false
if (lastModificationTime != other.lastModificationTime) return false
if (tags != other.tags) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.lookoutmetrics.model.AlertSummary = Builder(this).apply(block).build()
public class Builder {
/**
* The ARN of the alert.
*/
public var alertArn: kotlin.String? = null
/**
* The name of the alert.
*/
public var alertName: kotlin.String? = null
/**
* The minimum severity for an anomaly to trigger the alert.
*/
public var alertSensitivityThreshold: kotlin.Int = 0
/**
* The status of the alert.
*/
public var alertStatus: aws.sdk.kotlin.services.lookoutmetrics.model.AlertStatus? = null
/**
* The type of the alert.
*/
public var alertType: aws.sdk.kotlin.services.lookoutmetrics.model.AlertType? = null
/**
* The ARN of the detector to which the alert is attached.
*/
public var anomalyDetectorArn: kotlin.String? = null
/**
* The time at which the alert was created.
*/
public var creationTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The time at which the alert was last modified.
*/
public var lastModificationTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The alert's [tags](https://docs.aws.amazon.com/lookoutmetrics/latest/dev/detectors-tags.html).
*/
public var tags: Map? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.lookoutmetrics.model.AlertSummary) : this() {
this.alertArn = x.alertArn
this.alertName = x.alertName
this.alertSensitivityThreshold = x.alertSensitivityThreshold
this.alertStatus = x.alertStatus
this.alertType = x.alertType
this.anomalyDetectorArn = x.anomalyDetectorArn
this.creationTime = x.creationTime
this.lastModificationTime = x.lastModificationTime
this.tags = x.tags
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.lookoutmetrics.model.AlertSummary = AlertSummary(this)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy