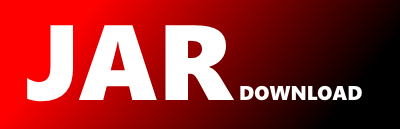
commonMain.aws.sdk.kotlin.services.mediaconvert.model.CreatePresetRequest.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mediaconvert Show documentation
Show all versions of mediaconvert Show documentation
The AWS SDK for Kotlin client for MediaConvert
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.mediaconvert.model
public class CreatePresetRequest private constructor(builder: Builder) {
/**
* Optional. A category for the preset you are creating.
*/
public val category: kotlin.String? = builder.category
/**
* Optional. A description of the preset you are creating.
*/
public val description: kotlin.String? = builder.description
/**
* The name of the preset you are creating.
*/
public val name: kotlin.String? = builder.name
/**
* Settings for preset
*/
public val settings: aws.sdk.kotlin.services.mediaconvert.model.PresetSettings? = builder.settings
/**
* The tags that you want to add to the resource. You can tag resources with a key-value pair or with only a key.
*/
public val tags: Map? = builder.tags
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.mediaconvert.model.CreatePresetRequest = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("CreatePresetRequest(")
append("category=$category,")
append("description=$description,")
append("name=$name,")
append("settings=$settings,")
append("tags=$tags")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = category?.hashCode() ?: 0
result = 31 * result + (description?.hashCode() ?: 0)
result = 31 * result + (name?.hashCode() ?: 0)
result = 31 * result + (settings?.hashCode() ?: 0)
result = 31 * result + (tags?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as CreatePresetRequest
if (category != other.category) return false
if (description != other.description) return false
if (name != other.name) return false
if (settings != other.settings) return false
if (tags != other.tags) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.mediaconvert.model.CreatePresetRequest = Builder(this).apply(block).build()
public class Builder {
/**
* Optional. A category for the preset you are creating.
*/
public var category: kotlin.String? = null
/**
* Optional. A description of the preset you are creating.
*/
public var description: kotlin.String? = null
/**
* The name of the preset you are creating.
*/
public var name: kotlin.String? = null
/**
* Settings for preset
*/
public var settings: aws.sdk.kotlin.services.mediaconvert.model.PresetSettings? = null
/**
* The tags that you want to add to the resource. You can tag resources with a key-value pair or with only a key.
*/
public var tags: Map? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.mediaconvert.model.CreatePresetRequest) : this() {
this.category = x.category
this.description = x.description
this.name = x.name
this.settings = x.settings
this.tags = x.tags
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.mediaconvert.model.CreatePresetRequest = CreatePresetRequest(this)
/**
* construct an [aws.sdk.kotlin.services.mediaconvert.model.PresetSettings] inside the given [block]
*/
public fun settings(block: aws.sdk.kotlin.services.mediaconvert.model.PresetSettings.Builder.() -> kotlin.Unit) {
this.settings = aws.sdk.kotlin.services.mediaconvert.model.PresetSettings.invoke(block)
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy