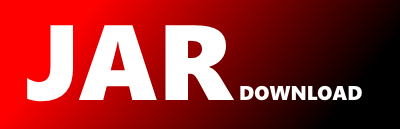
commonMain.aws.sdk.kotlin.services.mediaconvert.model.Mp3Settings.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mediaconvert Show documentation
Show all versions of mediaconvert Show documentation
The AWS SDK for Kotlin client for MediaConvert
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.mediaconvert.model
/**
* Required when you set Codec, under AudioDescriptions>CodecSettings, to the value MP3.
*/
public class Mp3Settings private constructor(builder: Builder) {
/**
* Specify the average bitrate in bits per second.
*/
public val bitrate: kotlin.Int = builder.bitrate
/**
* Specify the number of channels in this output audio track. Choosing Mono on the console gives you 1 output channel; choosing Stereo gives you 2. In the API, valid values are 1 and 2.
*/
public val channels: kotlin.Int = builder.channels
/**
* Specify whether the service encodes this MP3 audio output with a constant bitrate (CBR) or a variable bitrate (VBR).
*/
public val rateControlMode: aws.sdk.kotlin.services.mediaconvert.model.Mp3RateControlMode? = builder.rateControlMode
/**
* Sample rate in hz.
*/
public val sampleRate: kotlin.Int = builder.sampleRate
/**
* Required when you set Bitrate control mode (rateControlMode) to VBR. Specify the audio quality of this MP3 output from 0 (highest quality) to 9 (lowest quality).
*/
public val vbrQuality: kotlin.Int = builder.vbrQuality
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.mediaconvert.model.Mp3Settings = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("Mp3Settings(")
append("bitrate=$bitrate,")
append("channels=$channels,")
append("rateControlMode=$rateControlMode,")
append("sampleRate=$sampleRate,")
append("vbrQuality=$vbrQuality")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = bitrate
result = 31 * result + (channels)
result = 31 * result + (rateControlMode?.hashCode() ?: 0)
result = 31 * result + (sampleRate)
result = 31 * result + (vbrQuality)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as Mp3Settings
if (bitrate != other.bitrate) return false
if (channels != other.channels) return false
if (rateControlMode != other.rateControlMode) return false
if (sampleRate != other.sampleRate) return false
if (vbrQuality != other.vbrQuality) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.mediaconvert.model.Mp3Settings = Builder(this).apply(block).build()
public class Builder {
/**
* Specify the average bitrate in bits per second.
*/
public var bitrate: kotlin.Int = 0
/**
* Specify the number of channels in this output audio track. Choosing Mono on the console gives you 1 output channel; choosing Stereo gives you 2. In the API, valid values are 1 and 2.
*/
public var channels: kotlin.Int = 0
/**
* Specify whether the service encodes this MP3 audio output with a constant bitrate (CBR) or a variable bitrate (VBR).
*/
public var rateControlMode: aws.sdk.kotlin.services.mediaconvert.model.Mp3RateControlMode? = null
/**
* Sample rate in hz.
*/
public var sampleRate: kotlin.Int = 0
/**
* Required when you set Bitrate control mode (rateControlMode) to VBR. Specify the audio quality of this MP3 output from 0 (highest quality) to 9 (lowest quality).
*/
public var vbrQuality: kotlin.Int = 0
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.mediaconvert.model.Mp3Settings) : this() {
this.bitrate = x.bitrate
this.channels = x.channels
this.rateControlMode = x.rateControlMode
this.sampleRate = x.sampleRate
this.vbrQuality = x.vbrQuality
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.mediaconvert.model.Mp3Settings = Mp3Settings(this)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy