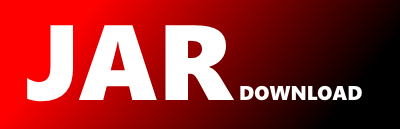
commonMain.aws.sdk.kotlin.services.mediapackage.model.DashPackage.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mediapackage-jvm Show documentation
Show all versions of mediapackage-jvm Show documentation
The AWS SDK for Kotlin client for MediaPackage
The newest version!
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.mediapackage.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* A Dynamic Adaptive Streaming over HTTP (DASH) packaging configuration.
*/
public class DashPackage private constructor(builder: Builder) {
/**
* A list of SCTE-35 message types that are treated as ad markers in the output. If empty, no ad markers are output. Specify multiple items to create ad markers for all of the included message types.
*/
public val adTriggers: List? = builder.adTriggers
/**
* This setting allows the delivery restriction flags on SCTE-35 segmentation descriptors to determine whether a message signals an ad. Choosing "NONE" means no SCTE-35 messages become ads. Choosing "RESTRICTED" means SCTE-35 messages of the types specified in AdTriggers that contain delivery restrictions will be treated as ads. Choosing "UNRESTRICTED" means SCTE-35 messages of the types specified in AdTriggers that do not contain delivery restrictions will be treated as ads. Choosing "BOTH" means all SCTE-35 messages of the types specified in AdTriggers will be treated as ads. Note that Splice Insert messages do not have these flags and are always treated as ads if specified in AdTriggers.
*/
public val adsOnDeliveryRestrictions: aws.sdk.kotlin.services.mediapackage.model.AdsOnDeliveryRestrictions? = builder.adsOnDeliveryRestrictions
/**
* A Dynamic Adaptive Streaming over HTTP (DASH) encryption configuration.
*/
public val encryption: aws.sdk.kotlin.services.mediapackage.model.DashEncryption? = builder.encryption
/**
* When enabled, an I-Frame only stream will be included in the output.
*/
public val includeIframeOnlyStream: kotlin.Boolean? = builder.includeIframeOnlyStream
/**
* Determines the position of some tags in the Media Presentation Description (MPD). When set to FULL, elements like SegmentTemplate and ContentProtection are included in each Representation. When set to COMPACT, duplicate elements are combined and presented at the AdaptationSet level. When set to DRM_TOP_LEVEL_COMPACT, content protection elements are placed the MPD level and referenced at the AdaptationSet level.
*/
public val manifestLayout: aws.sdk.kotlin.services.mediapackage.model.ManifestLayout? = builder.manifestLayout
/**
* Time window (in seconds) contained in each manifest.
*/
public val manifestWindowSeconds: kotlin.Int? = builder.manifestWindowSeconds
/**
* Minimum duration (in seconds) that a player will buffer media before starting the presentation.
*/
public val minBufferTimeSeconds: kotlin.Int? = builder.minBufferTimeSeconds
/**
* Minimum duration (in seconds) between potential changes to the Dynamic Adaptive Streaming over HTTP (DASH) Media Presentation Description (MPD).
*/
public val minUpdatePeriodSeconds: kotlin.Int? = builder.minUpdatePeriodSeconds
/**
* A list of triggers that controls when the outgoing Dynamic Adaptive Streaming over HTTP (DASH) Media Presentation Description (MPD) will be partitioned into multiple periods. If empty, the content will not be partitioned into more than one period. If the list contains "ADS", new periods will be created where the Channel source contains SCTE-35 ad markers.
*/
public val periodTriggers: List? = builder.periodTriggers
/**
* The Dynamic Adaptive Streaming over HTTP (DASH) profile type. When set to "HBBTV_1_5", HbbTV 1.5 compliant output is enabled. When set to "DVB-DASH_2014", DVB-DASH 2014 compliant output is enabled.
*/
public val profile: aws.sdk.kotlin.services.mediapackage.model.Profile? = builder.profile
/**
* Duration (in seconds) of each segment. Actual segments will be rounded to the nearest multiple of the source segment duration.
*/
public val segmentDurationSeconds: kotlin.Int? = builder.segmentDurationSeconds
/**
* Determines the type of SegmentTemplate included in the Media Presentation Description (MPD). When set to NUMBER_WITH_TIMELINE, a full timeline is presented in each SegmentTemplate, with $Number$ media URLs. When set to TIME_WITH_TIMELINE, a full timeline is presented in each SegmentTemplate, with $Time$ media URLs. When set to NUMBER_WITH_DURATION, only a duration is included in each SegmentTemplate, with $Number$ media URLs.
*/
public val segmentTemplateFormat: aws.sdk.kotlin.services.mediapackage.model.SegmentTemplateFormat? = builder.segmentTemplateFormat
/**
* A StreamSelection configuration.
*/
public val streamSelection: aws.sdk.kotlin.services.mediapackage.model.StreamSelection? = builder.streamSelection
/**
* Duration (in seconds) to delay live content before presentation.
*/
public val suggestedPresentationDelaySeconds: kotlin.Int? = builder.suggestedPresentationDelaySeconds
/**
* Determines the type of UTCTiming included in the Media Presentation Description (MPD)
*/
public val utcTiming: aws.sdk.kotlin.services.mediapackage.model.UtcTiming? = builder.utcTiming
/**
* Specifies the value attribute of the UTCTiming field when utcTiming is set to HTTP-ISO, HTTP-HEAD or HTTP-XSDATE
*/
public val utcTimingUri: kotlin.String? = builder.utcTimingUri
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.mediapackage.model.DashPackage = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("DashPackage(")
append("adTriggers=$adTriggers,")
append("adsOnDeliveryRestrictions=$adsOnDeliveryRestrictions,")
append("encryption=$encryption,")
append("includeIframeOnlyStream=$includeIframeOnlyStream,")
append("manifestLayout=$manifestLayout,")
append("manifestWindowSeconds=$manifestWindowSeconds,")
append("minBufferTimeSeconds=$minBufferTimeSeconds,")
append("minUpdatePeriodSeconds=$minUpdatePeriodSeconds,")
append("periodTriggers=$periodTriggers,")
append("profile=$profile,")
append("segmentDurationSeconds=$segmentDurationSeconds,")
append("segmentTemplateFormat=$segmentTemplateFormat,")
append("streamSelection=$streamSelection,")
append("suggestedPresentationDelaySeconds=$suggestedPresentationDelaySeconds,")
append("utcTiming=$utcTiming,")
append("utcTimingUri=$utcTimingUri")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = adTriggers?.hashCode() ?: 0
result = 31 * result + (adsOnDeliveryRestrictions?.hashCode() ?: 0)
result = 31 * result + (encryption?.hashCode() ?: 0)
result = 31 * result + (includeIframeOnlyStream?.hashCode() ?: 0)
result = 31 * result + (manifestLayout?.hashCode() ?: 0)
result = 31 * result + (manifestWindowSeconds ?: 0)
result = 31 * result + (minBufferTimeSeconds ?: 0)
result = 31 * result + (minUpdatePeriodSeconds ?: 0)
result = 31 * result + (periodTriggers?.hashCode() ?: 0)
result = 31 * result + (profile?.hashCode() ?: 0)
result = 31 * result + (segmentDurationSeconds ?: 0)
result = 31 * result + (segmentTemplateFormat?.hashCode() ?: 0)
result = 31 * result + (streamSelection?.hashCode() ?: 0)
result = 31 * result + (suggestedPresentationDelaySeconds ?: 0)
result = 31 * result + (utcTiming?.hashCode() ?: 0)
result = 31 * result + (utcTimingUri?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as DashPackage
if (adTriggers != other.adTriggers) return false
if (adsOnDeliveryRestrictions != other.adsOnDeliveryRestrictions) return false
if (encryption != other.encryption) return false
if (includeIframeOnlyStream != other.includeIframeOnlyStream) return false
if (manifestLayout != other.manifestLayout) return false
if (manifestWindowSeconds != other.manifestWindowSeconds) return false
if (minBufferTimeSeconds != other.minBufferTimeSeconds) return false
if (minUpdatePeriodSeconds != other.minUpdatePeriodSeconds) return false
if (periodTriggers != other.periodTriggers) return false
if (profile != other.profile) return false
if (segmentDurationSeconds != other.segmentDurationSeconds) return false
if (segmentTemplateFormat != other.segmentTemplateFormat) return false
if (streamSelection != other.streamSelection) return false
if (suggestedPresentationDelaySeconds != other.suggestedPresentationDelaySeconds) return false
if (utcTiming != other.utcTiming) return false
if (utcTimingUri != other.utcTimingUri) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.mediapackage.model.DashPackage = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* A list of SCTE-35 message types that are treated as ad markers in the output. If empty, no ad markers are output. Specify multiple items to create ad markers for all of the included message types.
*/
public var adTriggers: List? = null
/**
* This setting allows the delivery restriction flags on SCTE-35 segmentation descriptors to determine whether a message signals an ad. Choosing "NONE" means no SCTE-35 messages become ads. Choosing "RESTRICTED" means SCTE-35 messages of the types specified in AdTriggers that contain delivery restrictions will be treated as ads. Choosing "UNRESTRICTED" means SCTE-35 messages of the types specified in AdTriggers that do not contain delivery restrictions will be treated as ads. Choosing "BOTH" means all SCTE-35 messages of the types specified in AdTriggers will be treated as ads. Note that Splice Insert messages do not have these flags and are always treated as ads if specified in AdTriggers.
*/
public var adsOnDeliveryRestrictions: aws.sdk.kotlin.services.mediapackage.model.AdsOnDeliveryRestrictions? = null
/**
* A Dynamic Adaptive Streaming over HTTP (DASH) encryption configuration.
*/
public var encryption: aws.sdk.kotlin.services.mediapackage.model.DashEncryption? = null
/**
* When enabled, an I-Frame only stream will be included in the output.
*/
public var includeIframeOnlyStream: kotlin.Boolean? = null
/**
* Determines the position of some tags in the Media Presentation Description (MPD). When set to FULL, elements like SegmentTemplate and ContentProtection are included in each Representation. When set to COMPACT, duplicate elements are combined and presented at the AdaptationSet level. When set to DRM_TOP_LEVEL_COMPACT, content protection elements are placed the MPD level and referenced at the AdaptationSet level.
*/
public var manifestLayout: aws.sdk.kotlin.services.mediapackage.model.ManifestLayout? = null
/**
* Time window (in seconds) contained in each manifest.
*/
public var manifestWindowSeconds: kotlin.Int? = null
/**
* Minimum duration (in seconds) that a player will buffer media before starting the presentation.
*/
public var minBufferTimeSeconds: kotlin.Int? = null
/**
* Minimum duration (in seconds) between potential changes to the Dynamic Adaptive Streaming over HTTP (DASH) Media Presentation Description (MPD).
*/
public var minUpdatePeriodSeconds: kotlin.Int? = null
/**
* A list of triggers that controls when the outgoing Dynamic Adaptive Streaming over HTTP (DASH) Media Presentation Description (MPD) will be partitioned into multiple periods. If empty, the content will not be partitioned into more than one period. If the list contains "ADS", new periods will be created where the Channel source contains SCTE-35 ad markers.
*/
public var periodTriggers: List? = null
/**
* The Dynamic Adaptive Streaming over HTTP (DASH) profile type. When set to "HBBTV_1_5", HbbTV 1.5 compliant output is enabled. When set to "DVB-DASH_2014", DVB-DASH 2014 compliant output is enabled.
*/
public var profile: aws.sdk.kotlin.services.mediapackage.model.Profile? = null
/**
* Duration (in seconds) of each segment. Actual segments will be rounded to the nearest multiple of the source segment duration.
*/
public var segmentDurationSeconds: kotlin.Int? = null
/**
* Determines the type of SegmentTemplate included in the Media Presentation Description (MPD). When set to NUMBER_WITH_TIMELINE, a full timeline is presented in each SegmentTemplate, with $Number$ media URLs. When set to TIME_WITH_TIMELINE, a full timeline is presented in each SegmentTemplate, with $Time$ media URLs. When set to NUMBER_WITH_DURATION, only a duration is included in each SegmentTemplate, with $Number$ media URLs.
*/
public var segmentTemplateFormat: aws.sdk.kotlin.services.mediapackage.model.SegmentTemplateFormat? = null
/**
* A StreamSelection configuration.
*/
public var streamSelection: aws.sdk.kotlin.services.mediapackage.model.StreamSelection? = null
/**
* Duration (in seconds) to delay live content before presentation.
*/
public var suggestedPresentationDelaySeconds: kotlin.Int? = null
/**
* Determines the type of UTCTiming included in the Media Presentation Description (MPD)
*/
public var utcTiming: aws.sdk.kotlin.services.mediapackage.model.UtcTiming? = null
/**
* Specifies the value attribute of the UTCTiming field when utcTiming is set to HTTP-ISO, HTTP-HEAD or HTTP-XSDATE
*/
public var utcTimingUri: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.mediapackage.model.DashPackage) : this() {
this.adTriggers = x.adTriggers
this.adsOnDeliveryRestrictions = x.adsOnDeliveryRestrictions
this.encryption = x.encryption
this.includeIframeOnlyStream = x.includeIframeOnlyStream
this.manifestLayout = x.manifestLayout
this.manifestWindowSeconds = x.manifestWindowSeconds
this.minBufferTimeSeconds = x.minBufferTimeSeconds
this.minUpdatePeriodSeconds = x.minUpdatePeriodSeconds
this.periodTriggers = x.periodTriggers
this.profile = x.profile
this.segmentDurationSeconds = x.segmentDurationSeconds
this.segmentTemplateFormat = x.segmentTemplateFormat
this.streamSelection = x.streamSelection
this.suggestedPresentationDelaySeconds = x.suggestedPresentationDelaySeconds
this.utcTiming = x.utcTiming
this.utcTimingUri = x.utcTimingUri
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.mediapackage.model.DashPackage = DashPackage(this)
/**
* construct an [aws.sdk.kotlin.services.mediapackage.model.DashEncryption] inside the given [block]
*/
public fun encryption(block: aws.sdk.kotlin.services.mediapackage.model.DashEncryption.Builder.() -> kotlin.Unit) {
this.encryption = aws.sdk.kotlin.services.mediapackage.model.DashEncryption.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.mediapackage.model.StreamSelection] inside the given [block]
*/
public fun streamSelection(block: aws.sdk.kotlin.services.mediapackage.model.StreamSelection.Builder.() -> kotlin.Unit) {
this.streamSelection = aws.sdk.kotlin.services.mediapackage.model.StreamSelection.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy