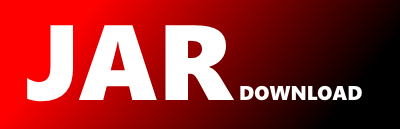
commonMain.aws.sdk.kotlin.services.mediapackage.model.Channel.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mediapackage Show documentation
Show all versions of mediapackage Show documentation
AWS Elemental MediaPackage
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.mediapackage.model
/**
* A Channel resource configuration.
*/
class Channel private constructor(builder: Builder) {
/**
* The Amazon Resource Name (ARN) assigned to the Channel.
*/
val arn: kotlin.String? = builder.arn
/**
* A short text description of the Channel.
*/
val description: kotlin.String? = builder.description
/**
* Configure egress access logging.
*/
val egressAccessLogs: aws.sdk.kotlin.services.mediapackage.model.EgressAccessLogs? = builder.egressAccessLogs
/**
* An HTTP Live Streaming (HLS) ingest resource configuration.
*/
val hlsIngest: aws.sdk.kotlin.services.mediapackage.model.HlsIngest? = builder.hlsIngest
/**
* The ID of the Channel.
*/
val id: kotlin.String? = builder.id
/**
* Configure ingress access logging.
*/
val ingressAccessLogs: aws.sdk.kotlin.services.mediapackage.model.IngressAccessLogs? = builder.ingressAccessLogs
/**
* A collection of tags associated with a resource
*/
val tags: Map? = builder.tags
companion object {
operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.mediapackage.model.Channel = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("Channel(")
append("arn=$arn,")
append("description=$description,")
append("egressAccessLogs=$egressAccessLogs,")
append("hlsIngest=$hlsIngest,")
append("id=$id,")
append("ingressAccessLogs=$ingressAccessLogs,")
append("tags=$tags)")
}
override fun hashCode(): kotlin.Int {
var result = arn?.hashCode() ?: 0
result = 31 * result + (description?.hashCode() ?: 0)
result = 31 * result + (egressAccessLogs?.hashCode() ?: 0)
result = 31 * result + (hlsIngest?.hashCode() ?: 0)
result = 31 * result + (id?.hashCode() ?: 0)
result = 31 * result + (ingressAccessLogs?.hashCode() ?: 0)
result = 31 * result + (tags?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as Channel
if (arn != other.arn) return false
if (description != other.description) return false
if (egressAccessLogs != other.egressAccessLogs) return false
if (hlsIngest != other.hlsIngest) return false
if (id != other.id) return false
if (ingressAccessLogs != other.ingressAccessLogs) return false
if (tags != other.tags) return false
return true
}
inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.mediapackage.model.Channel = Builder(this).apply(block).build()
class Builder {
/**
* The Amazon Resource Name (ARN) assigned to the Channel.
*/
var arn: kotlin.String? = null
/**
* A short text description of the Channel.
*/
var description: kotlin.String? = null
/**
* Configure egress access logging.
*/
var egressAccessLogs: aws.sdk.kotlin.services.mediapackage.model.EgressAccessLogs? = null
/**
* An HTTP Live Streaming (HLS) ingest resource configuration.
*/
var hlsIngest: aws.sdk.kotlin.services.mediapackage.model.HlsIngest? = null
/**
* The ID of the Channel.
*/
var id: kotlin.String? = null
/**
* Configure ingress access logging.
*/
var ingressAccessLogs: aws.sdk.kotlin.services.mediapackage.model.IngressAccessLogs? = null
/**
* A collection of tags associated with a resource
*/
var tags: Map? = null
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.mediapackage.model.Channel) : this() {
this.arn = x.arn
this.description = x.description
this.egressAccessLogs = x.egressAccessLogs
this.hlsIngest = x.hlsIngest
this.id = x.id
this.ingressAccessLogs = x.ingressAccessLogs
this.tags = x.tags
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.mediapackage.model.Channel = Channel(this)
/**
* construct an [aws.sdk.kotlin.services.mediapackage.model.EgressAccessLogs] inside the given [block]
*/
fun egressAccessLogs(block: aws.sdk.kotlin.services.mediapackage.model.EgressAccessLogs.Builder.() -> kotlin.Unit) {
this.egressAccessLogs = aws.sdk.kotlin.services.mediapackage.model.EgressAccessLogs.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.mediapackage.model.HlsIngest] inside the given [block]
*/
fun hlsIngest(block: aws.sdk.kotlin.services.mediapackage.model.HlsIngest.Builder.() -> kotlin.Unit) {
this.hlsIngest = aws.sdk.kotlin.services.mediapackage.model.HlsIngest.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.mediapackage.model.IngressAccessLogs] inside the given [block]
*/
fun ingressAccessLogs(block: aws.sdk.kotlin.services.mediapackage.model.IngressAccessLogs.Builder.() -> kotlin.Unit) {
this.ingressAccessLogs = aws.sdk.kotlin.services.mediapackage.model.IngressAccessLogs.invoke(block)
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy