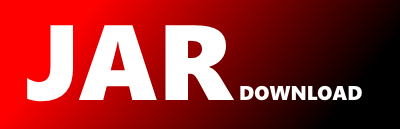
commonMain.aws.sdk.kotlin.services.mediapackage.model.CreateOriginEndpointResponse.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mediapackage Show documentation
Show all versions of mediapackage Show documentation
AWS Elemental MediaPackage
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.mediapackage.model
class CreateOriginEndpointResponse private constructor(builder: Builder) {
/**
* The Amazon Resource Name (ARN) assigned to the OriginEndpoint.
*/
val arn: kotlin.String? = builder.arn
/**
* CDN Authorization credentials
*/
val authorization: aws.sdk.kotlin.services.mediapackage.model.Authorization? = builder.authorization
/**
* The ID of the Channel the OriginEndpoint is associated with.
*/
val channelId: kotlin.String? = builder.channelId
/**
* A Common Media Application Format (CMAF) packaging configuration.
*/
val cmafPackage: aws.sdk.kotlin.services.mediapackage.model.CmafPackage? = builder.cmafPackage
/**
* A Dynamic Adaptive Streaming over HTTP (DASH) packaging configuration.
*/
val dashPackage: aws.sdk.kotlin.services.mediapackage.model.DashPackage? = builder.dashPackage
/**
* A short text description of the OriginEndpoint.
*/
val description: kotlin.String? = builder.description
/**
* An HTTP Live Streaming (HLS) packaging configuration.
*/
val hlsPackage: aws.sdk.kotlin.services.mediapackage.model.HlsPackage? = builder.hlsPackage
/**
* The ID of the OriginEndpoint.
*/
val id: kotlin.String? = builder.id
/**
* A short string appended to the end of the OriginEndpoint URL.
*/
val manifestName: kotlin.String? = builder.manifestName
/**
* A Microsoft Smooth Streaming (MSS) packaging configuration.
*/
val mssPackage: aws.sdk.kotlin.services.mediapackage.model.MssPackage? = builder.mssPackage
/**
* Control whether origination of video is allowed for this OriginEndpoint. If set to ALLOW, the OriginEndpoint may by requested, pursuant to any other form of access control. If set to DENY, the OriginEndpoint may not be requested. This can be helpful for Live to VOD harvesting, or for temporarily disabling origination
*/
val origination: aws.sdk.kotlin.services.mediapackage.model.Origination? = builder.origination
/**
* Maximum duration (seconds) of content to retain for startover playback. If not specified, startover playback will be disabled for the OriginEndpoint.
*/
val startoverWindowSeconds: kotlin.Int? = builder.startoverWindowSeconds
/**
* A collection of tags associated with a resource
*/
val tags: Map? = builder.tags
/**
* Amount of delay (seconds) to enforce on the playback of live content. If not specified, there will be no time delay in effect for the OriginEndpoint.
*/
val timeDelaySeconds: kotlin.Int? = builder.timeDelaySeconds
/**
* The URL of the packaged OriginEndpoint for consumption.
*/
val url: kotlin.String? = builder.url
/**
* A list of source IP CIDR blocks that will be allowed to access the OriginEndpoint.
*/
val whitelist: List? = builder.whitelist
companion object {
operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.mediapackage.model.CreateOriginEndpointResponse = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("CreateOriginEndpointResponse(")
append("arn=$arn,")
append("authorization=$authorization,")
append("channelId=$channelId,")
append("cmafPackage=$cmafPackage,")
append("dashPackage=$dashPackage,")
append("description=$description,")
append("hlsPackage=$hlsPackage,")
append("id=$id,")
append("manifestName=$manifestName,")
append("mssPackage=$mssPackage,")
append("origination=$origination,")
append("startoverWindowSeconds=$startoverWindowSeconds,")
append("tags=$tags,")
append("timeDelaySeconds=$timeDelaySeconds,")
append("url=$url,")
append("whitelist=$whitelist)")
}
override fun hashCode(): kotlin.Int {
var result = arn?.hashCode() ?: 0
result = 31 * result + (authorization?.hashCode() ?: 0)
result = 31 * result + (channelId?.hashCode() ?: 0)
result = 31 * result + (cmafPackage?.hashCode() ?: 0)
result = 31 * result + (dashPackage?.hashCode() ?: 0)
result = 31 * result + (description?.hashCode() ?: 0)
result = 31 * result + (hlsPackage?.hashCode() ?: 0)
result = 31 * result + (id?.hashCode() ?: 0)
result = 31 * result + (manifestName?.hashCode() ?: 0)
result = 31 * result + (mssPackage?.hashCode() ?: 0)
result = 31 * result + (origination?.hashCode() ?: 0)
result = 31 * result + (startoverWindowSeconds ?: 0)
result = 31 * result + (tags?.hashCode() ?: 0)
result = 31 * result + (timeDelaySeconds ?: 0)
result = 31 * result + (url?.hashCode() ?: 0)
result = 31 * result + (whitelist?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as CreateOriginEndpointResponse
if (arn != other.arn) return false
if (authorization != other.authorization) return false
if (channelId != other.channelId) return false
if (cmafPackage != other.cmafPackage) return false
if (dashPackage != other.dashPackage) return false
if (description != other.description) return false
if (hlsPackage != other.hlsPackage) return false
if (id != other.id) return false
if (manifestName != other.manifestName) return false
if (mssPackage != other.mssPackage) return false
if (origination != other.origination) return false
if (startoverWindowSeconds != other.startoverWindowSeconds) return false
if (tags != other.tags) return false
if (timeDelaySeconds != other.timeDelaySeconds) return false
if (url != other.url) return false
if (whitelist != other.whitelist) return false
return true
}
inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.mediapackage.model.CreateOriginEndpointResponse = Builder(this).apply(block).build()
class Builder {
/**
* The Amazon Resource Name (ARN) assigned to the OriginEndpoint.
*/
var arn: kotlin.String? = null
/**
* CDN Authorization credentials
*/
var authorization: aws.sdk.kotlin.services.mediapackage.model.Authorization? = null
/**
* The ID of the Channel the OriginEndpoint is associated with.
*/
var channelId: kotlin.String? = null
/**
* A Common Media Application Format (CMAF) packaging configuration.
*/
var cmafPackage: aws.sdk.kotlin.services.mediapackage.model.CmafPackage? = null
/**
* A Dynamic Adaptive Streaming over HTTP (DASH) packaging configuration.
*/
var dashPackage: aws.sdk.kotlin.services.mediapackage.model.DashPackage? = null
/**
* A short text description of the OriginEndpoint.
*/
var description: kotlin.String? = null
/**
* An HTTP Live Streaming (HLS) packaging configuration.
*/
var hlsPackage: aws.sdk.kotlin.services.mediapackage.model.HlsPackage? = null
/**
* The ID of the OriginEndpoint.
*/
var id: kotlin.String? = null
/**
* A short string appended to the end of the OriginEndpoint URL.
*/
var manifestName: kotlin.String? = null
/**
* A Microsoft Smooth Streaming (MSS) packaging configuration.
*/
var mssPackage: aws.sdk.kotlin.services.mediapackage.model.MssPackage? = null
/**
* Control whether origination of video is allowed for this OriginEndpoint. If set to ALLOW, the OriginEndpoint may by requested, pursuant to any other form of access control. If set to DENY, the OriginEndpoint may not be requested. This can be helpful for Live to VOD harvesting, or for temporarily disabling origination
*/
var origination: aws.sdk.kotlin.services.mediapackage.model.Origination? = null
/**
* Maximum duration (seconds) of content to retain for startover playback. If not specified, startover playback will be disabled for the OriginEndpoint.
*/
var startoverWindowSeconds: kotlin.Int? = null
/**
* A collection of tags associated with a resource
*/
var tags: Map? = null
/**
* Amount of delay (seconds) to enforce on the playback of live content. If not specified, there will be no time delay in effect for the OriginEndpoint.
*/
var timeDelaySeconds: kotlin.Int? = null
/**
* The URL of the packaged OriginEndpoint for consumption.
*/
var url: kotlin.String? = null
/**
* A list of source IP CIDR blocks that will be allowed to access the OriginEndpoint.
*/
var whitelist: List? = null
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.mediapackage.model.CreateOriginEndpointResponse) : this() {
this.arn = x.arn
this.authorization = x.authorization
this.channelId = x.channelId
this.cmafPackage = x.cmafPackage
this.dashPackage = x.dashPackage
this.description = x.description
this.hlsPackage = x.hlsPackage
this.id = x.id
this.manifestName = x.manifestName
this.mssPackage = x.mssPackage
this.origination = x.origination
this.startoverWindowSeconds = x.startoverWindowSeconds
this.tags = x.tags
this.timeDelaySeconds = x.timeDelaySeconds
this.url = x.url
this.whitelist = x.whitelist
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.mediapackage.model.CreateOriginEndpointResponse = CreateOriginEndpointResponse(this)
/**
* construct an [aws.sdk.kotlin.services.mediapackage.model.Authorization] inside the given [block]
*/
fun authorization(block: aws.sdk.kotlin.services.mediapackage.model.Authorization.Builder.() -> kotlin.Unit) {
this.authorization = aws.sdk.kotlin.services.mediapackage.model.Authorization.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.mediapackage.model.CmafPackage] inside the given [block]
*/
fun cmafPackage(block: aws.sdk.kotlin.services.mediapackage.model.CmafPackage.Builder.() -> kotlin.Unit) {
this.cmafPackage = aws.sdk.kotlin.services.mediapackage.model.CmafPackage.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.mediapackage.model.DashPackage] inside the given [block]
*/
fun dashPackage(block: aws.sdk.kotlin.services.mediapackage.model.DashPackage.Builder.() -> kotlin.Unit) {
this.dashPackage = aws.sdk.kotlin.services.mediapackage.model.DashPackage.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.mediapackage.model.HlsPackage] inside the given [block]
*/
fun hlsPackage(block: aws.sdk.kotlin.services.mediapackage.model.HlsPackage.Builder.() -> kotlin.Unit) {
this.hlsPackage = aws.sdk.kotlin.services.mediapackage.model.HlsPackage.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.mediapackage.model.MssPackage] inside the given [block]
*/
fun mssPackage(block: aws.sdk.kotlin.services.mediapackage.model.MssPackage.Builder.() -> kotlin.Unit) {
this.mssPackage = aws.sdk.kotlin.services.mediapackage.model.MssPackage.invoke(block)
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy