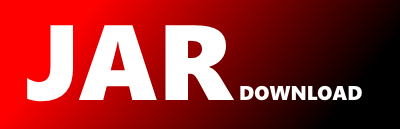
commonMain.aws.sdk.kotlin.services.mediapackagevod.model.DashManifest.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mediapackagevod-jvm Show documentation
Show all versions of mediapackagevod-jvm Show documentation
The AWS SDK for Kotlin client for MediaPackage Vod
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.mediapackagevod.model
/**
* A DASH manifest configuration.
*/
public class DashManifest private constructor(builder: Builder) {
/**
* Determines the position of some tags in the Media Presentation Description (MPD). When set to FULL, elements like SegmentTemplate and ContentProtection are included in each Representation. When set to COMPACT, duplicate elements are combined and presented at the AdaptationSet level.
*/
public val manifestLayout: aws.sdk.kotlin.services.mediapackagevod.model.ManifestLayout? = builder.manifestLayout
/**
* An optional string to include in the name of the manifest.
*/
public val manifestName: kotlin.String? = builder.manifestName
/**
* Minimum duration (in seconds) that a player will buffer media before starting the presentation.
*/
public val minBufferTimeSeconds: kotlin.Int? = builder.minBufferTimeSeconds
/**
* The Dynamic Adaptive Streaming over HTTP (DASH) profile type. When set to "HBBTV_1_5", HbbTV 1.5 compliant output is enabled.
*/
public val profile: aws.sdk.kotlin.services.mediapackagevod.model.Profile? = builder.profile
/**
* The source of scte markers used. When set to SEGMENTS, the scte markers are sourced from the segments of the ingested content. When set to MANIFEST, the scte markers are sourced from the manifest of the ingested content.
*/
public val scteMarkersSource: aws.sdk.kotlin.services.mediapackagevod.model.ScteMarkersSource? = builder.scteMarkersSource
/**
* A StreamSelection configuration.
*/
public val streamSelection: aws.sdk.kotlin.services.mediapackagevod.model.StreamSelection? = builder.streamSelection
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.mediapackagevod.model.DashManifest = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("DashManifest(")
append("manifestLayout=$manifestLayout,")
append("manifestName=$manifestName,")
append("minBufferTimeSeconds=$minBufferTimeSeconds,")
append("profile=$profile,")
append("scteMarkersSource=$scteMarkersSource,")
append("streamSelection=$streamSelection")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = manifestLayout?.hashCode() ?: 0
result = 31 * result + (manifestName?.hashCode() ?: 0)
result = 31 * result + (minBufferTimeSeconds ?: 0)
result = 31 * result + (profile?.hashCode() ?: 0)
result = 31 * result + (scteMarkersSource?.hashCode() ?: 0)
result = 31 * result + (streamSelection?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as DashManifest
if (manifestLayout != other.manifestLayout) return false
if (manifestName != other.manifestName) return false
if (minBufferTimeSeconds != other.minBufferTimeSeconds) return false
if (profile != other.profile) return false
if (scteMarkersSource != other.scteMarkersSource) return false
if (streamSelection != other.streamSelection) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.mediapackagevod.model.DashManifest = Builder(this).apply(block).build()
public class Builder {
/**
* Determines the position of some tags in the Media Presentation Description (MPD). When set to FULL, elements like SegmentTemplate and ContentProtection are included in each Representation. When set to COMPACT, duplicate elements are combined and presented at the AdaptationSet level.
*/
public var manifestLayout: aws.sdk.kotlin.services.mediapackagevod.model.ManifestLayout? = null
/**
* An optional string to include in the name of the manifest.
*/
public var manifestName: kotlin.String? = null
/**
* Minimum duration (in seconds) that a player will buffer media before starting the presentation.
*/
public var minBufferTimeSeconds: kotlin.Int? = null
/**
* The Dynamic Adaptive Streaming over HTTP (DASH) profile type. When set to "HBBTV_1_5", HbbTV 1.5 compliant output is enabled.
*/
public var profile: aws.sdk.kotlin.services.mediapackagevod.model.Profile? = null
/**
* The source of scte markers used. When set to SEGMENTS, the scte markers are sourced from the segments of the ingested content. When set to MANIFEST, the scte markers are sourced from the manifest of the ingested content.
*/
public var scteMarkersSource: aws.sdk.kotlin.services.mediapackagevod.model.ScteMarkersSource? = null
/**
* A StreamSelection configuration.
*/
public var streamSelection: aws.sdk.kotlin.services.mediapackagevod.model.StreamSelection? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.mediapackagevod.model.DashManifest) : this() {
this.manifestLayout = x.manifestLayout
this.manifestName = x.manifestName
this.minBufferTimeSeconds = x.minBufferTimeSeconds
this.profile = x.profile
this.scteMarkersSource = x.scteMarkersSource
this.streamSelection = x.streamSelection
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.mediapackagevod.model.DashManifest = DashManifest(this)
/**
* construct an [aws.sdk.kotlin.services.mediapackagevod.model.StreamSelection] inside the given [block]
*/
public fun streamSelection(block: aws.sdk.kotlin.services.mediapackagevod.model.StreamSelection.Builder.() -> kotlin.Unit) {
this.streamSelection = aws.sdk.kotlin.services.mediapackagevod.model.StreamSelection.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy