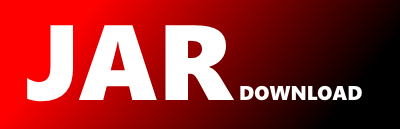
commonMain.aws.sdk.kotlin.services.mediapackagevod.model.DashPackage.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mediapackagevod-jvm Show documentation
Show all versions of mediapackagevod-jvm Show documentation
The AWS SDK for Kotlin client for MediaPackage Vod
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.mediapackagevod.model
/**
* A Dynamic Adaptive Streaming over HTTP (DASH) packaging configuration.
*/
public class DashPackage private constructor(builder: Builder) {
/**
* A list of DASH manifest configurations.
*/
public val dashManifests: List? = builder.dashManifests
/**
* A Dynamic Adaptive Streaming over HTTP (DASH) encryption configuration.
*/
public val encryption: aws.sdk.kotlin.services.mediapackagevod.model.DashEncryption? = builder.encryption
/**
* When includeEncoderConfigurationInSegments is set to true, MediaPackage places your encoder's Sequence Parameter Set (SPS), Picture Parameter Set (PPS), and Video Parameter Set (VPS) metadata in every video segment instead of in the init fragment. This lets you use different SPS/PPS/VPS settings for your assets during content playback.
*/
public val includeEncoderConfigurationInSegments: kotlin.Boolean? = builder.includeEncoderConfigurationInSegments
/**
* When enabled, an I-Frame only stream will be included in the output.
*/
public val includeIframeOnlyStream: kotlin.Boolean? = builder.includeIframeOnlyStream
/**
* A list of triggers that controls when the outgoing Dynamic Adaptive Streaming over HTTP (DASH) Media Presentation Description (MPD) will be partitioned into multiple periods. If empty, the content will not be partitioned into more than one period. If the list contains "ADS", new periods will be created where the Asset contains SCTE-35 ad markers.
*/
public val periodTriggers: List? = builder.periodTriggers
/**
* Duration (in seconds) of each segment. Actual segments will be rounded to the nearest multiple of the source segment duration.
*/
public val segmentDurationSeconds: kotlin.Int? = builder.segmentDurationSeconds
/**
* Determines the type of SegmentTemplate included in the Media Presentation Description (MPD). When set to NUMBER_WITH_TIMELINE, a full timeline is presented in each SegmentTemplate, with $Number$ media URLs. When set to TIME_WITH_TIMELINE, a full timeline is presented in each SegmentTemplate, with $Time$ media URLs. When set to NUMBER_WITH_DURATION, only a duration is included in each SegmentTemplate, with $Number$ media URLs.
*/
public val segmentTemplateFormat: aws.sdk.kotlin.services.mediapackagevod.model.SegmentTemplateFormat? = builder.segmentTemplateFormat
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.mediapackagevod.model.DashPackage = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("DashPackage(")
append("dashManifests=$dashManifests,")
append("encryption=$encryption,")
append("includeEncoderConfigurationInSegments=$includeEncoderConfigurationInSegments,")
append("includeIframeOnlyStream=$includeIframeOnlyStream,")
append("periodTriggers=$periodTriggers,")
append("segmentDurationSeconds=$segmentDurationSeconds,")
append("segmentTemplateFormat=$segmentTemplateFormat")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = dashManifests?.hashCode() ?: 0
result = 31 * result + (encryption?.hashCode() ?: 0)
result = 31 * result + (includeEncoderConfigurationInSegments?.hashCode() ?: 0)
result = 31 * result + (includeIframeOnlyStream?.hashCode() ?: 0)
result = 31 * result + (periodTriggers?.hashCode() ?: 0)
result = 31 * result + (segmentDurationSeconds ?: 0)
result = 31 * result + (segmentTemplateFormat?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as DashPackage
if (dashManifests != other.dashManifests) return false
if (encryption != other.encryption) return false
if (includeEncoderConfigurationInSegments != other.includeEncoderConfigurationInSegments) return false
if (includeIframeOnlyStream != other.includeIframeOnlyStream) return false
if (periodTriggers != other.periodTriggers) return false
if (segmentDurationSeconds != other.segmentDurationSeconds) return false
if (segmentTemplateFormat != other.segmentTemplateFormat) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.mediapackagevod.model.DashPackage = Builder(this).apply(block).build()
public class Builder {
/**
* A list of DASH manifest configurations.
*/
public var dashManifests: List? = null
/**
* A Dynamic Adaptive Streaming over HTTP (DASH) encryption configuration.
*/
public var encryption: aws.sdk.kotlin.services.mediapackagevod.model.DashEncryption? = null
/**
* When includeEncoderConfigurationInSegments is set to true, MediaPackage places your encoder's Sequence Parameter Set (SPS), Picture Parameter Set (PPS), and Video Parameter Set (VPS) metadata in every video segment instead of in the init fragment. This lets you use different SPS/PPS/VPS settings for your assets during content playback.
*/
public var includeEncoderConfigurationInSegments: kotlin.Boolean? = null
/**
* When enabled, an I-Frame only stream will be included in the output.
*/
public var includeIframeOnlyStream: kotlin.Boolean? = null
/**
* A list of triggers that controls when the outgoing Dynamic Adaptive Streaming over HTTP (DASH) Media Presentation Description (MPD) will be partitioned into multiple periods. If empty, the content will not be partitioned into more than one period. If the list contains "ADS", new periods will be created where the Asset contains SCTE-35 ad markers.
*/
public var periodTriggers: List? = null
/**
* Duration (in seconds) of each segment. Actual segments will be rounded to the nearest multiple of the source segment duration.
*/
public var segmentDurationSeconds: kotlin.Int? = null
/**
* Determines the type of SegmentTemplate included in the Media Presentation Description (MPD). When set to NUMBER_WITH_TIMELINE, a full timeline is presented in each SegmentTemplate, with $Number$ media URLs. When set to TIME_WITH_TIMELINE, a full timeline is presented in each SegmentTemplate, with $Time$ media URLs. When set to NUMBER_WITH_DURATION, only a duration is included in each SegmentTemplate, with $Number$ media URLs.
*/
public var segmentTemplateFormat: aws.sdk.kotlin.services.mediapackagevod.model.SegmentTemplateFormat? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.mediapackagevod.model.DashPackage) : this() {
this.dashManifests = x.dashManifests
this.encryption = x.encryption
this.includeEncoderConfigurationInSegments = x.includeEncoderConfigurationInSegments
this.includeIframeOnlyStream = x.includeIframeOnlyStream
this.periodTriggers = x.periodTriggers
this.segmentDurationSeconds = x.segmentDurationSeconds
this.segmentTemplateFormat = x.segmentTemplateFormat
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.mediapackagevod.model.DashPackage = DashPackage(this)
/**
* construct an [aws.sdk.kotlin.services.mediapackagevod.model.DashEncryption] inside the given [block]
*/
public fun encryption(block: aws.sdk.kotlin.services.mediapackagevod.model.DashEncryption.Builder.() -> kotlin.Unit) {
this.encryption = aws.sdk.kotlin.services.mediapackagevod.model.DashEncryption.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy