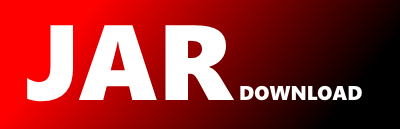
commonMain.aws.sdk.kotlin.services.mediapackagevod.model.CmafPackage.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mediapackagevod-jvm Show documentation
Show all versions of mediapackagevod-jvm Show documentation
The AWS SDK for Kotlin client for MediaPackage Vod
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.mediapackagevod.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* A CMAF packaging configuration.
*/
public class CmafPackage private constructor(builder: Builder) {
/**
* A CMAF encryption configuration.
*/
public val encryption: aws.sdk.kotlin.services.mediapackagevod.model.CmafEncryption? = builder.encryption
/**
* A list of HLS manifest configurations.
*/
public val hlsManifests: List? = builder.hlsManifests
/**
* When includeEncoderConfigurationInSegments is set to true, MediaPackage places your encoder's Sequence Parameter Set (SPS), Picture Parameter Set (PPS), and Video Parameter Set (VPS) metadata in every video segment instead of in the init fragment. This lets you use different SPS/PPS/VPS settings for your assets during content playback.
*/
public val includeEncoderConfigurationInSegments: kotlin.Boolean? = builder.includeEncoderConfigurationInSegments
/**
* Duration (in seconds) of each fragment. Actual fragments will be rounded to the nearest multiple of the source fragment duration.
*/
public val segmentDurationSeconds: kotlin.Int? = builder.segmentDurationSeconds
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.mediapackagevod.model.CmafPackage = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("CmafPackage(")
append("encryption=$encryption,")
append("hlsManifests=$hlsManifests,")
append("includeEncoderConfigurationInSegments=$includeEncoderConfigurationInSegments,")
append("segmentDurationSeconds=$segmentDurationSeconds")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = encryption?.hashCode() ?: 0
result = 31 * result + (hlsManifests?.hashCode() ?: 0)
result = 31 * result + (includeEncoderConfigurationInSegments?.hashCode() ?: 0)
result = 31 * result + (segmentDurationSeconds ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as CmafPackage
if (encryption != other.encryption) return false
if (hlsManifests != other.hlsManifests) return false
if (includeEncoderConfigurationInSegments != other.includeEncoderConfigurationInSegments) return false
if (segmentDurationSeconds != other.segmentDurationSeconds) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.mediapackagevod.model.CmafPackage = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* A CMAF encryption configuration.
*/
public var encryption: aws.sdk.kotlin.services.mediapackagevod.model.CmafEncryption? = null
/**
* A list of HLS manifest configurations.
*/
public var hlsManifests: List? = null
/**
* When includeEncoderConfigurationInSegments is set to true, MediaPackage places your encoder's Sequence Parameter Set (SPS), Picture Parameter Set (PPS), and Video Parameter Set (VPS) metadata in every video segment instead of in the init fragment. This lets you use different SPS/PPS/VPS settings for your assets during content playback.
*/
public var includeEncoderConfigurationInSegments: kotlin.Boolean? = null
/**
* Duration (in seconds) of each fragment. Actual fragments will be rounded to the nearest multiple of the source fragment duration.
*/
public var segmentDurationSeconds: kotlin.Int? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.mediapackagevod.model.CmafPackage) : this() {
this.encryption = x.encryption
this.hlsManifests = x.hlsManifests
this.includeEncoderConfigurationInSegments = x.includeEncoderConfigurationInSegments
this.segmentDurationSeconds = x.segmentDurationSeconds
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.mediapackagevod.model.CmafPackage = CmafPackage(this)
/**
* construct an [aws.sdk.kotlin.services.mediapackagevod.model.CmafEncryption] inside the given [block]
*/
public fun encryption(block: aws.sdk.kotlin.services.mediapackagevod.model.CmafEncryption.Builder.() -> kotlin.Unit) {
this.encryption = aws.sdk.kotlin.services.mediapackagevod.model.CmafEncryption.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy