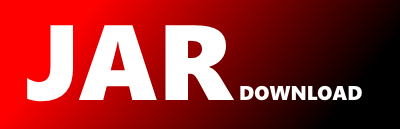
aws.sdk.kotlin.services.migrationhubrefactorspaces.model.GetApplicationResponse.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.migrationhubrefactorspaces.model
import aws.smithy.kotlin.runtime.time.Instant
class GetApplicationResponse private constructor(builder: Builder) {
/**
* The endpoint URL of the API Gateway proxy.
*/
val apiGatewayProxy: aws.sdk.kotlin.services.migrationhubrefactorspaces.model.ApiGatewayProxyConfig? = builder.apiGatewayProxy
/**
* The unique identifier of the application.
*/
val applicationId: kotlin.String? = builder.applicationId
/**
* The Amazon Resource Name (ARN) of the application.
*/
val arn: kotlin.String? = builder.arn
/**
* The Amazon Web Services account ID of the application creator.
*/
val createdByAccountId: kotlin.String? = builder.createdByAccountId
/**
* A timestamp that indicates when the application is created.
*/
val createdTime: aws.smithy.kotlin.runtime.time.Instant? = builder.createdTime
/**
* The unique identifier of the environment.
*/
val environmentId: kotlin.String? = builder.environmentId
/**
* Any error associated with the application resource.
*/
val error: aws.sdk.kotlin.services.migrationhubrefactorspaces.model.ErrorResponse? = builder.error
/**
* A timestamp that indicates when the application was last updated.
*/
val lastUpdatedTime: aws.smithy.kotlin.runtime.time.Instant? = builder.lastUpdatedTime
/**
* The name of the application.
*/
val name: kotlin.String? = builder.name
/**
* The Amazon Web Services account ID of the application owner.
*/
val ownerAccountId: kotlin.String? = builder.ownerAccountId
/**
* The proxy type of the proxy created within the application.
*/
val proxyType: aws.sdk.kotlin.services.migrationhubrefactorspaces.model.ProxyType? = builder.proxyType
/**
* The current state of the application.
*/
val state: aws.sdk.kotlin.services.migrationhubrefactorspaces.model.ApplicationState? = builder.state
/**
* The tags assigned to the application. A tag is a label that you assign to an Amazon Web Services resource. Each tag consists of a key-value pair.
*/
val tags: Map? = builder.tags
/**
* The ID of the virtual private cloud (VPC).
*/
val vpcId: kotlin.String? = builder.vpcId
companion object {
operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.migrationhubrefactorspaces.model.GetApplicationResponse = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("GetApplicationResponse(")
append("apiGatewayProxy=$apiGatewayProxy,")
append("applicationId=$applicationId,")
append("arn=$arn,")
append("createdByAccountId=$createdByAccountId,")
append("createdTime=$createdTime,")
append("environmentId=$environmentId,")
append("error=$error,")
append("lastUpdatedTime=$lastUpdatedTime,")
append("name=$name,")
append("ownerAccountId=$ownerAccountId,")
append("proxyType=$proxyType,")
append("state=$state,")
append("tags=*** Sensitive Data Redacted ***,")
append("vpcId=$vpcId)")
}
override fun hashCode(): kotlin.Int {
var result = apiGatewayProxy?.hashCode() ?: 0
result = 31 * result + (applicationId?.hashCode() ?: 0)
result = 31 * result + (arn?.hashCode() ?: 0)
result = 31 * result + (createdByAccountId?.hashCode() ?: 0)
result = 31 * result + (createdTime?.hashCode() ?: 0)
result = 31 * result + (environmentId?.hashCode() ?: 0)
result = 31 * result + (error?.hashCode() ?: 0)
result = 31 * result + (lastUpdatedTime?.hashCode() ?: 0)
result = 31 * result + (name?.hashCode() ?: 0)
result = 31 * result + (ownerAccountId?.hashCode() ?: 0)
result = 31 * result + (proxyType?.hashCode() ?: 0)
result = 31 * result + (state?.hashCode() ?: 0)
result = 31 * result + (tags?.hashCode() ?: 0)
result = 31 * result + (vpcId?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as GetApplicationResponse
if (apiGatewayProxy != other.apiGatewayProxy) return false
if (applicationId != other.applicationId) return false
if (arn != other.arn) return false
if (createdByAccountId != other.createdByAccountId) return false
if (createdTime != other.createdTime) return false
if (environmentId != other.environmentId) return false
if (error != other.error) return false
if (lastUpdatedTime != other.lastUpdatedTime) return false
if (name != other.name) return false
if (ownerAccountId != other.ownerAccountId) return false
if (proxyType != other.proxyType) return false
if (state != other.state) return false
if (tags != other.tags) return false
if (vpcId != other.vpcId) return false
return true
}
inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.migrationhubrefactorspaces.model.GetApplicationResponse = Builder(this).apply(block).build()
class Builder {
/**
* The endpoint URL of the API Gateway proxy.
*/
var apiGatewayProxy: aws.sdk.kotlin.services.migrationhubrefactorspaces.model.ApiGatewayProxyConfig? = null
/**
* The unique identifier of the application.
*/
var applicationId: kotlin.String? = null
/**
* The Amazon Resource Name (ARN) of the application.
*/
var arn: kotlin.String? = null
/**
* The Amazon Web Services account ID of the application creator.
*/
var createdByAccountId: kotlin.String? = null
/**
* A timestamp that indicates when the application is created.
*/
var createdTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The unique identifier of the environment.
*/
var environmentId: kotlin.String? = null
/**
* Any error associated with the application resource.
*/
var error: aws.sdk.kotlin.services.migrationhubrefactorspaces.model.ErrorResponse? = null
/**
* A timestamp that indicates when the application was last updated.
*/
var lastUpdatedTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The name of the application.
*/
var name: kotlin.String? = null
/**
* The Amazon Web Services account ID of the application owner.
*/
var ownerAccountId: kotlin.String? = null
/**
* The proxy type of the proxy created within the application.
*/
var proxyType: aws.sdk.kotlin.services.migrationhubrefactorspaces.model.ProxyType? = null
/**
* The current state of the application.
*/
var state: aws.sdk.kotlin.services.migrationhubrefactorspaces.model.ApplicationState? = null
/**
* The tags assigned to the application. A tag is a label that you assign to an Amazon Web Services resource. Each tag consists of a key-value pair.
*/
var tags: Map? = null
/**
* The ID of the virtual private cloud (VPC).
*/
var vpcId: kotlin.String? = null
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.migrationhubrefactorspaces.model.GetApplicationResponse) : this() {
this.apiGatewayProxy = x.apiGatewayProxy
this.applicationId = x.applicationId
this.arn = x.arn
this.createdByAccountId = x.createdByAccountId
this.createdTime = x.createdTime
this.environmentId = x.environmentId
this.error = x.error
this.lastUpdatedTime = x.lastUpdatedTime
this.name = x.name
this.ownerAccountId = x.ownerAccountId
this.proxyType = x.proxyType
this.state = x.state
this.tags = x.tags
this.vpcId = x.vpcId
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.migrationhubrefactorspaces.model.GetApplicationResponse = GetApplicationResponse(this)
/**
* construct an [aws.sdk.kotlin.services.migrationhubrefactorspaces.model.ApiGatewayProxyConfig] inside the given [block]
*/
fun apiGatewayProxy(block: aws.sdk.kotlin.services.migrationhubrefactorspaces.model.ApiGatewayProxyConfig.Builder.() -> kotlin.Unit) {
this.apiGatewayProxy = aws.sdk.kotlin.services.migrationhubrefactorspaces.model.ApiGatewayProxyConfig.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.migrationhubrefactorspaces.model.ErrorResponse] inside the given [block]
*/
fun error(block: aws.sdk.kotlin.services.migrationhubrefactorspaces.model.ErrorResponse.Builder.() -> kotlin.Unit) {
this.error = aws.sdk.kotlin.services.migrationhubrefactorspaces.model.ErrorResponse.invoke(block)
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy