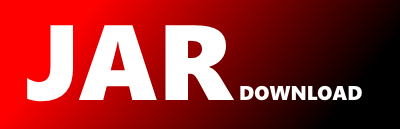
commonMain.aws.sdk.kotlin.services.outposts.model.Address.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of outposts-jvm Show documentation
Show all versions of outposts-jvm Show documentation
The AWS SDK for Kotlin client for Outposts
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.outposts.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* Information about an address.
*/
public class Address private constructor(builder: Builder) {
/**
* The first line of the address.
*/
public val addressLine1: kotlin.String = requireNotNull(builder.addressLine1) { "A non-null value must be provided for addressLine1" }
/**
* The second line of the address.
*/
public val addressLine2: kotlin.String? = builder.addressLine2
/**
* The third line of the address.
*/
public val addressLine3: kotlin.String? = builder.addressLine3
/**
* The city for the address.
*/
public val city: kotlin.String = requireNotNull(builder.city) { "A non-null value must be provided for city" }
/**
* The name of the contact.
*/
public val contactName: kotlin.String? = builder.contactName
/**
* The phone number of the contact.
*/
public val contactPhoneNumber: kotlin.String? = builder.contactPhoneNumber
/**
* The ISO-3166 two-letter country code for the address.
*/
public val countryCode: kotlin.String = requireNotNull(builder.countryCode) { "A non-null value must be provided for countryCode" }
/**
* The district or county for the address.
*/
public val districtOrCounty: kotlin.String? = builder.districtOrCounty
/**
* The municipality for the address.
*/
public val municipality: kotlin.String? = builder.municipality
/**
* The postal code for the address.
*/
public val postalCode: kotlin.String = requireNotNull(builder.postalCode) { "A non-null value must be provided for postalCode" }
/**
* The state for the address.
*/
public val stateOrRegion: kotlin.String = requireNotNull(builder.stateOrRegion) { "A non-null value must be provided for stateOrRegion" }
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.outposts.model.Address = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("Address(")
append("addressLine1=$addressLine1,")
append("addressLine2=$addressLine2,")
append("addressLine3=$addressLine3,")
append("city=$city,")
append("contactName=$contactName,")
append("contactPhoneNumber=$contactPhoneNumber,")
append("countryCode=$countryCode,")
append("districtOrCounty=$districtOrCounty,")
append("municipality=$municipality,")
append("postalCode=$postalCode,")
append("stateOrRegion=$stateOrRegion")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = addressLine1.hashCode()
result = 31 * result + (addressLine2?.hashCode() ?: 0)
result = 31 * result + (addressLine3?.hashCode() ?: 0)
result = 31 * result + (city.hashCode())
result = 31 * result + (contactName?.hashCode() ?: 0)
result = 31 * result + (contactPhoneNumber?.hashCode() ?: 0)
result = 31 * result + (countryCode.hashCode())
result = 31 * result + (districtOrCounty?.hashCode() ?: 0)
result = 31 * result + (municipality?.hashCode() ?: 0)
result = 31 * result + (postalCode.hashCode())
result = 31 * result + (stateOrRegion.hashCode())
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as Address
if (addressLine1 != other.addressLine1) return false
if (addressLine2 != other.addressLine2) return false
if (addressLine3 != other.addressLine3) return false
if (city != other.city) return false
if (contactName != other.contactName) return false
if (contactPhoneNumber != other.contactPhoneNumber) return false
if (countryCode != other.countryCode) return false
if (districtOrCounty != other.districtOrCounty) return false
if (municipality != other.municipality) return false
if (postalCode != other.postalCode) return false
if (stateOrRegion != other.stateOrRegion) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.outposts.model.Address = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The first line of the address.
*/
public var addressLine1: kotlin.String? = null
/**
* The second line of the address.
*/
public var addressLine2: kotlin.String? = null
/**
* The third line of the address.
*/
public var addressLine3: kotlin.String? = null
/**
* The city for the address.
*/
public var city: kotlin.String? = null
/**
* The name of the contact.
*/
public var contactName: kotlin.String? = null
/**
* The phone number of the contact.
*/
public var contactPhoneNumber: kotlin.String? = null
/**
* The ISO-3166 two-letter country code for the address.
*/
public var countryCode: kotlin.String? = null
/**
* The district or county for the address.
*/
public var districtOrCounty: kotlin.String? = null
/**
* The municipality for the address.
*/
public var municipality: kotlin.String? = null
/**
* The postal code for the address.
*/
public var postalCode: kotlin.String? = null
/**
* The state for the address.
*/
public var stateOrRegion: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.outposts.model.Address) : this() {
this.addressLine1 = x.addressLine1
this.addressLine2 = x.addressLine2
this.addressLine3 = x.addressLine3
this.city = x.city
this.contactName = x.contactName
this.contactPhoneNumber = x.contactPhoneNumber
this.countryCode = x.countryCode
this.districtOrCounty = x.districtOrCounty
this.municipality = x.municipality
this.postalCode = x.postalCode
this.stateOrRegion = x.stateOrRegion
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.outposts.model.Address = Address(this)
internal fun correctErrors(): Builder {
if (addressLine1 == null) addressLine1 = ""
if (city == null) city = ""
if (countryCode == null) countryCode = ""
if (postalCode == null) postalCode = ""
if (stateOrRegion == null) stateOrRegion = ""
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy