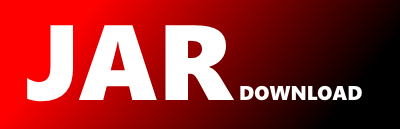
commonMain.aws.sdk.kotlin.services.outposts.model.ComputeAttributes.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of outposts-jvm Show documentation
Show all versions of outposts-jvm Show documentation
The AWS SDK for Kotlin client for Outposts
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.outposts.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* Information about compute hardware assets.
*/
public class ComputeAttributes private constructor(builder: Builder) {
/**
* The host ID of the Dedicated Host on the asset.
*/
public val hostId: kotlin.String? = builder.hostId
/**
* A list of the names of instance families that are currently associated with a given asset.
*/
public val instanceFamilies: List? = builder.instanceFamilies
/**
* The instance type capacities configured for this asset. This can be changed through a capacity task.
*/
public val instanceTypeCapacities: List? = builder.instanceTypeCapacities
/**
* The maximum number of vCPUs possible for the specified asset.
*/
public val maxVcpus: kotlin.Int? = builder.maxVcpus
/**
* The state.
* + ACTIVE - The asset is available and can provide capacity for new compute resources.
* + ISOLATED - The asset is undergoing maintenance and can't provide capacity for new compute resources. Existing compute resources on the asset are not affected.
* + RETIRING - The underlying hardware for the asset is degraded. Capacity for new compute resources is reduced. Amazon Web Services sends notifications for resources that must be stopped before the asset can be replaced.
*/
public val state: aws.sdk.kotlin.services.outposts.model.ComputeAssetState? = builder.state
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.outposts.model.ComputeAttributes = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("ComputeAttributes(")
append("hostId=$hostId,")
append("instanceFamilies=$instanceFamilies,")
append("instanceTypeCapacities=$instanceTypeCapacities,")
append("maxVcpus=$maxVcpus,")
append("state=$state")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = hostId?.hashCode() ?: 0
result = 31 * result + (instanceFamilies?.hashCode() ?: 0)
result = 31 * result + (instanceTypeCapacities?.hashCode() ?: 0)
result = 31 * result + (maxVcpus ?: 0)
result = 31 * result + (state?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as ComputeAttributes
if (hostId != other.hostId) return false
if (instanceFamilies != other.instanceFamilies) return false
if (instanceTypeCapacities != other.instanceTypeCapacities) return false
if (maxVcpus != other.maxVcpus) return false
if (state != other.state) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.outposts.model.ComputeAttributes = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The host ID of the Dedicated Host on the asset.
*/
public var hostId: kotlin.String? = null
/**
* A list of the names of instance families that are currently associated with a given asset.
*/
public var instanceFamilies: List? = null
/**
* The instance type capacities configured for this asset. This can be changed through a capacity task.
*/
public var instanceTypeCapacities: List? = null
/**
* The maximum number of vCPUs possible for the specified asset.
*/
public var maxVcpus: kotlin.Int? = null
/**
* The state.
* + ACTIVE - The asset is available and can provide capacity for new compute resources.
* + ISOLATED - The asset is undergoing maintenance and can't provide capacity for new compute resources. Existing compute resources on the asset are not affected.
* + RETIRING - The underlying hardware for the asset is degraded. Capacity for new compute resources is reduced. Amazon Web Services sends notifications for resources that must be stopped before the asset can be replaced.
*/
public var state: aws.sdk.kotlin.services.outposts.model.ComputeAssetState? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.outposts.model.ComputeAttributes) : this() {
this.hostId = x.hostId
this.instanceFamilies = x.instanceFamilies
this.instanceTypeCapacities = x.instanceTypeCapacities
this.maxVcpus = x.maxVcpus
this.state = x.state
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.outposts.model.ComputeAttributes = ComputeAttributes(this)
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy