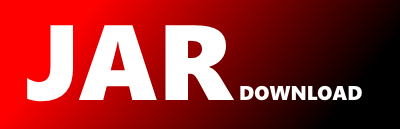
commonMain.aws.sdk.kotlin.services.outposts.model.RackPhysicalProperties.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of outposts-jvm Show documentation
Show all versions of outposts-jvm Show documentation
The AWS SDK for Kotlin client for Outposts
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.outposts.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* Information about the physical and logistical details for racks at sites. For more information about hardware requirements for racks, see [Network readiness checklist](https://docs.aws.amazon.com/outposts/latest/userguide/outposts-requirements.html#checklist) in the Amazon Web Services Outposts User Guide.
*/
public class RackPhysicalProperties private constructor(builder: Builder) {
/**
* The type of fiber used to attach the Outpost to the network.
*/
public val fiberOpticCableType: aws.sdk.kotlin.services.outposts.model.FiberOpticCableType? = builder.fiberOpticCableType
/**
* The maximum rack weight that this site can support. `NO_LIMIT` is over 2000 lbs (907 kg).
*/
public val maximumSupportedWeightLbs: aws.sdk.kotlin.services.outposts.model.MaximumSupportedWeightLbs? = builder.maximumSupportedWeightLbs
/**
* The type of optical standard used to attach the Outpost to the network. This field is dependent on uplink speed, fiber type, and distance to the upstream device. For more information about networking requirements for racks, see [Network](https://docs.aws.amazon.com/outposts/latest/userguide/outposts-requirements.html#facility-networking) in the Amazon Web Services Outposts User Guide.
*/
public val opticalStandard: aws.sdk.kotlin.services.outposts.model.OpticalStandard? = builder.opticalStandard
/**
* The power connector for the hardware.
*/
public val powerConnector: aws.sdk.kotlin.services.outposts.model.PowerConnector? = builder.powerConnector
/**
* The power draw available at the hardware placement position for the rack.
*/
public val powerDrawKva: aws.sdk.kotlin.services.outposts.model.PowerDrawKva? = builder.powerDrawKva
/**
* The position of the power feed.
*/
public val powerFeedDrop: aws.sdk.kotlin.services.outposts.model.PowerFeedDrop? = builder.powerFeedDrop
/**
* The power option that you can provide for hardware.
*/
public val powerPhase: aws.sdk.kotlin.services.outposts.model.PowerPhase? = builder.powerPhase
/**
* The number of uplinks each Outpost network device.
*/
public val uplinkCount: aws.sdk.kotlin.services.outposts.model.UplinkCount? = builder.uplinkCount
/**
* The uplink speed the rack supports for the connection to the Region.
*/
public val uplinkGbps: aws.sdk.kotlin.services.outposts.model.UplinkGbps? = builder.uplinkGbps
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.outposts.model.RackPhysicalProperties = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("RackPhysicalProperties(")
append("fiberOpticCableType=$fiberOpticCableType,")
append("maximumSupportedWeightLbs=$maximumSupportedWeightLbs,")
append("opticalStandard=$opticalStandard,")
append("powerConnector=$powerConnector,")
append("powerDrawKva=$powerDrawKva,")
append("powerFeedDrop=$powerFeedDrop,")
append("powerPhase=$powerPhase,")
append("uplinkCount=$uplinkCount,")
append("uplinkGbps=$uplinkGbps")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = fiberOpticCableType?.hashCode() ?: 0
result = 31 * result + (maximumSupportedWeightLbs?.hashCode() ?: 0)
result = 31 * result + (opticalStandard?.hashCode() ?: 0)
result = 31 * result + (powerConnector?.hashCode() ?: 0)
result = 31 * result + (powerDrawKva?.hashCode() ?: 0)
result = 31 * result + (powerFeedDrop?.hashCode() ?: 0)
result = 31 * result + (powerPhase?.hashCode() ?: 0)
result = 31 * result + (uplinkCount?.hashCode() ?: 0)
result = 31 * result + (uplinkGbps?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as RackPhysicalProperties
if (fiberOpticCableType != other.fiberOpticCableType) return false
if (maximumSupportedWeightLbs != other.maximumSupportedWeightLbs) return false
if (opticalStandard != other.opticalStandard) return false
if (powerConnector != other.powerConnector) return false
if (powerDrawKva != other.powerDrawKva) return false
if (powerFeedDrop != other.powerFeedDrop) return false
if (powerPhase != other.powerPhase) return false
if (uplinkCount != other.uplinkCount) return false
if (uplinkGbps != other.uplinkGbps) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.outposts.model.RackPhysicalProperties = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The type of fiber used to attach the Outpost to the network.
*/
public var fiberOpticCableType: aws.sdk.kotlin.services.outposts.model.FiberOpticCableType? = null
/**
* The maximum rack weight that this site can support. `NO_LIMIT` is over 2000 lbs (907 kg).
*/
public var maximumSupportedWeightLbs: aws.sdk.kotlin.services.outposts.model.MaximumSupportedWeightLbs? = null
/**
* The type of optical standard used to attach the Outpost to the network. This field is dependent on uplink speed, fiber type, and distance to the upstream device. For more information about networking requirements for racks, see [Network](https://docs.aws.amazon.com/outposts/latest/userguide/outposts-requirements.html#facility-networking) in the Amazon Web Services Outposts User Guide.
*/
public var opticalStandard: aws.sdk.kotlin.services.outposts.model.OpticalStandard? = null
/**
* The power connector for the hardware.
*/
public var powerConnector: aws.sdk.kotlin.services.outposts.model.PowerConnector? = null
/**
* The power draw available at the hardware placement position for the rack.
*/
public var powerDrawKva: aws.sdk.kotlin.services.outposts.model.PowerDrawKva? = null
/**
* The position of the power feed.
*/
public var powerFeedDrop: aws.sdk.kotlin.services.outposts.model.PowerFeedDrop? = null
/**
* The power option that you can provide for hardware.
*/
public var powerPhase: aws.sdk.kotlin.services.outposts.model.PowerPhase? = null
/**
* The number of uplinks each Outpost network device.
*/
public var uplinkCount: aws.sdk.kotlin.services.outposts.model.UplinkCount? = null
/**
* The uplink speed the rack supports for the connection to the Region.
*/
public var uplinkGbps: aws.sdk.kotlin.services.outposts.model.UplinkGbps? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.outposts.model.RackPhysicalProperties) : this() {
this.fiberOpticCableType = x.fiberOpticCableType
this.maximumSupportedWeightLbs = x.maximumSupportedWeightLbs
this.opticalStandard = x.opticalStandard
this.powerConnector = x.powerConnector
this.powerDrawKva = x.powerDrawKva
this.powerFeedDrop = x.powerFeedDrop
this.powerPhase = x.powerPhase
this.uplinkCount = x.uplinkCount
this.uplinkGbps = x.uplinkGbps
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.outposts.model.RackPhysicalProperties = RackPhysicalProperties(this)
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy