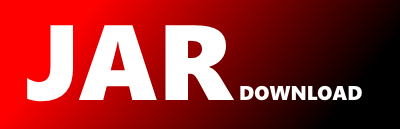
commonMain.aws.sdk.kotlin.services.outposts.model.StartCapacityTaskRequest.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of outposts-jvm Show documentation
Show all versions of outposts-jvm Show documentation
The AWS SDK for Kotlin client for Outposts
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.outposts.model
import aws.smithy.kotlin.runtime.SdkDsl
public class StartCapacityTaskRequest private constructor(builder: Builder) {
/**
* You can request a dry run to determine if the instance type and instance size changes is above or below available instance capacity. Requesting a dry run does not make any changes to your plan.
*/
public val dryRun: kotlin.Boolean? = builder.dryRun
/**
* The instance pools specified in the capacity task.
*/
public val instancePools: List? = builder.instancePools
/**
* List of user-specified running instances that must not be stopped in order to free up the capacity needed to run the capacity task.
*/
public val instancesToExclude: aws.sdk.kotlin.services.outposts.model.InstancesToExclude? = builder.instancesToExclude
/**
* The ID of the Amazon Web Services Outposts order associated with the specified capacity task.
*/
public val orderId: kotlin.String? = builder.orderId
/**
* The ID or ARN of the Outposts associated with the specified capacity task.
*/
public val outpostIdentifier: kotlin.String? = builder.outpostIdentifier
/**
* Specify one of the following options in case an instance is blocking the capacity task from running.
* + `WAIT_FOR_EVACUATION` - Checks every 10 minutes over 48 hours to determine if instances have stopped and capacity is available to complete the task.
* + `FAIL_TASK` - The capacity task fails.
*/
public val taskActionOnBlockingInstances: aws.sdk.kotlin.services.outposts.model.TaskActionOnBlockingInstances? = builder.taskActionOnBlockingInstances
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.outposts.model.StartCapacityTaskRequest = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("StartCapacityTaskRequest(")
append("dryRun=$dryRun,")
append("instancePools=$instancePools,")
append("instancesToExclude=$instancesToExclude,")
append("orderId=$orderId,")
append("outpostIdentifier=$outpostIdentifier,")
append("taskActionOnBlockingInstances=$taskActionOnBlockingInstances")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = dryRun?.hashCode() ?: 0
result = 31 * result + (instancePools?.hashCode() ?: 0)
result = 31 * result + (instancesToExclude?.hashCode() ?: 0)
result = 31 * result + (orderId?.hashCode() ?: 0)
result = 31 * result + (outpostIdentifier?.hashCode() ?: 0)
result = 31 * result + (taskActionOnBlockingInstances?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as StartCapacityTaskRequest
if (dryRun != other.dryRun) return false
if (instancePools != other.instancePools) return false
if (instancesToExclude != other.instancesToExclude) return false
if (orderId != other.orderId) return false
if (outpostIdentifier != other.outpostIdentifier) return false
if (taskActionOnBlockingInstances != other.taskActionOnBlockingInstances) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.outposts.model.StartCapacityTaskRequest = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* You can request a dry run to determine if the instance type and instance size changes is above or below available instance capacity. Requesting a dry run does not make any changes to your plan.
*/
public var dryRun: kotlin.Boolean? = null
/**
* The instance pools specified in the capacity task.
*/
public var instancePools: List? = null
/**
* List of user-specified running instances that must not be stopped in order to free up the capacity needed to run the capacity task.
*/
public var instancesToExclude: aws.sdk.kotlin.services.outposts.model.InstancesToExclude? = null
/**
* The ID of the Amazon Web Services Outposts order associated with the specified capacity task.
*/
public var orderId: kotlin.String? = null
/**
* The ID or ARN of the Outposts associated with the specified capacity task.
*/
public var outpostIdentifier: kotlin.String? = null
/**
* Specify one of the following options in case an instance is blocking the capacity task from running.
* + `WAIT_FOR_EVACUATION` - Checks every 10 minutes over 48 hours to determine if instances have stopped and capacity is available to complete the task.
* + `FAIL_TASK` - The capacity task fails.
*/
public var taskActionOnBlockingInstances: aws.sdk.kotlin.services.outposts.model.TaskActionOnBlockingInstances? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.outposts.model.StartCapacityTaskRequest) : this() {
this.dryRun = x.dryRun
this.instancePools = x.instancePools
this.instancesToExclude = x.instancesToExclude
this.orderId = x.orderId
this.outpostIdentifier = x.outpostIdentifier
this.taskActionOnBlockingInstances = x.taskActionOnBlockingInstances
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.outposts.model.StartCapacityTaskRequest = StartCapacityTaskRequest(this)
/**
* construct an [aws.sdk.kotlin.services.outposts.model.InstancesToExclude] inside the given [block]
*/
public fun instancesToExclude(block: aws.sdk.kotlin.services.outposts.model.InstancesToExclude.Builder.() -> kotlin.Unit) {
this.instancesToExclude = aws.sdk.kotlin.services.outposts.model.InstancesToExclude.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy