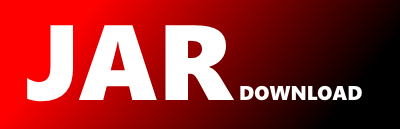
commonMain.aws.sdk.kotlin.services.outposts.model.StartCapacityTaskResponse.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of outposts-jvm Show documentation
Show all versions of outposts-jvm Show documentation
The AWS SDK for Kotlin client for Outposts
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.outposts.model
import aws.smithy.kotlin.runtime.SdkDsl
import aws.smithy.kotlin.runtime.time.Instant
public class StartCapacityTaskResponse private constructor(builder: Builder) {
/**
* ID of the capacity task that you want to start.
*/
public val capacityTaskId: kotlin.String? = builder.capacityTaskId
/**
* Status of the specified capacity task.
*/
public val capacityTaskStatus: aws.sdk.kotlin.services.outposts.model.CapacityTaskStatus? = builder.capacityTaskStatus
/**
* Date that the specified capacity task ran successfully.
*/
public val completionDate: aws.smithy.kotlin.runtime.time.Instant? = builder.completionDate
/**
* Date that the specified capacity task was created.
*/
public val creationDate: aws.smithy.kotlin.runtime.time.Instant? = builder.creationDate
/**
* Results of the dry run showing if the specified capacity task is above or below the available instance capacity.
*/
public val dryRun: kotlin.Boolean = builder.dryRun
/**
* Reason that the specified capacity task failed.
*/
public val failed: aws.sdk.kotlin.services.outposts.model.CapacityTaskFailure? = builder.failed
/**
* User-specified instances that must not be stopped in order to free up the capacity needed to run the capacity task.
*/
public val instancesToExclude: aws.sdk.kotlin.services.outposts.model.InstancesToExclude? = builder.instancesToExclude
/**
* Date that the specified capacity task was last modified.
*/
public val lastModifiedDate: aws.smithy.kotlin.runtime.time.Instant? = builder.lastModifiedDate
/**
* ID of the Amazon Web Services Outposts order of the host associated with the capacity task.
*/
public val orderId: kotlin.String? = builder.orderId
/**
* ID of the Outpost associated with the capacity task.
*/
public val outpostId: kotlin.String? = builder.outpostId
/**
* List of the instance pools requested in the specified capacity task.
*/
public val requestedInstancePools: List? = builder.requestedInstancePools
/**
* User-specified option in case an instance is blocking the capacity task from running.
* + `WAIT_FOR_EVACUATION` - Checks every 10 minutes over 48 hours to determine if instances have stopped and capacity is available to complete the task.
* + `FAIL_TASK` - The capacity task fails.
*/
public val taskActionOnBlockingInstances: aws.sdk.kotlin.services.outposts.model.TaskActionOnBlockingInstances? = builder.taskActionOnBlockingInstances
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.outposts.model.StartCapacityTaskResponse = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("StartCapacityTaskResponse(")
append("capacityTaskId=$capacityTaskId,")
append("capacityTaskStatus=$capacityTaskStatus,")
append("completionDate=$completionDate,")
append("creationDate=$creationDate,")
append("dryRun=$dryRun,")
append("failed=$failed,")
append("instancesToExclude=$instancesToExclude,")
append("lastModifiedDate=$lastModifiedDate,")
append("orderId=$orderId,")
append("outpostId=$outpostId,")
append("requestedInstancePools=$requestedInstancePools,")
append("taskActionOnBlockingInstances=$taskActionOnBlockingInstances")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = capacityTaskId?.hashCode() ?: 0
result = 31 * result + (capacityTaskStatus?.hashCode() ?: 0)
result = 31 * result + (completionDate?.hashCode() ?: 0)
result = 31 * result + (creationDate?.hashCode() ?: 0)
result = 31 * result + (dryRun.hashCode())
result = 31 * result + (failed?.hashCode() ?: 0)
result = 31 * result + (instancesToExclude?.hashCode() ?: 0)
result = 31 * result + (lastModifiedDate?.hashCode() ?: 0)
result = 31 * result + (orderId?.hashCode() ?: 0)
result = 31 * result + (outpostId?.hashCode() ?: 0)
result = 31 * result + (requestedInstancePools?.hashCode() ?: 0)
result = 31 * result + (taskActionOnBlockingInstances?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as StartCapacityTaskResponse
if (capacityTaskId != other.capacityTaskId) return false
if (capacityTaskStatus != other.capacityTaskStatus) return false
if (completionDate != other.completionDate) return false
if (creationDate != other.creationDate) return false
if (dryRun != other.dryRun) return false
if (failed != other.failed) return false
if (instancesToExclude != other.instancesToExclude) return false
if (lastModifiedDate != other.lastModifiedDate) return false
if (orderId != other.orderId) return false
if (outpostId != other.outpostId) return false
if (requestedInstancePools != other.requestedInstancePools) return false
if (taskActionOnBlockingInstances != other.taskActionOnBlockingInstances) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.outposts.model.StartCapacityTaskResponse = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* ID of the capacity task that you want to start.
*/
public var capacityTaskId: kotlin.String? = null
/**
* Status of the specified capacity task.
*/
public var capacityTaskStatus: aws.sdk.kotlin.services.outposts.model.CapacityTaskStatus? = null
/**
* Date that the specified capacity task ran successfully.
*/
public var completionDate: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* Date that the specified capacity task was created.
*/
public var creationDate: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* Results of the dry run showing if the specified capacity task is above or below the available instance capacity.
*/
public var dryRun: kotlin.Boolean = false
/**
* Reason that the specified capacity task failed.
*/
public var failed: aws.sdk.kotlin.services.outposts.model.CapacityTaskFailure? = null
/**
* User-specified instances that must not be stopped in order to free up the capacity needed to run the capacity task.
*/
public var instancesToExclude: aws.sdk.kotlin.services.outposts.model.InstancesToExclude? = null
/**
* Date that the specified capacity task was last modified.
*/
public var lastModifiedDate: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* ID of the Amazon Web Services Outposts order of the host associated with the capacity task.
*/
public var orderId: kotlin.String? = null
/**
* ID of the Outpost associated with the capacity task.
*/
public var outpostId: kotlin.String? = null
/**
* List of the instance pools requested in the specified capacity task.
*/
public var requestedInstancePools: List? = null
/**
* User-specified option in case an instance is blocking the capacity task from running.
* + `WAIT_FOR_EVACUATION` - Checks every 10 minutes over 48 hours to determine if instances have stopped and capacity is available to complete the task.
* + `FAIL_TASK` - The capacity task fails.
*/
public var taskActionOnBlockingInstances: aws.sdk.kotlin.services.outposts.model.TaskActionOnBlockingInstances? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.outposts.model.StartCapacityTaskResponse) : this() {
this.capacityTaskId = x.capacityTaskId
this.capacityTaskStatus = x.capacityTaskStatus
this.completionDate = x.completionDate
this.creationDate = x.creationDate
this.dryRun = x.dryRun
this.failed = x.failed
this.instancesToExclude = x.instancesToExclude
this.lastModifiedDate = x.lastModifiedDate
this.orderId = x.orderId
this.outpostId = x.outpostId
this.requestedInstancePools = x.requestedInstancePools
this.taskActionOnBlockingInstances = x.taskActionOnBlockingInstances
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.outposts.model.StartCapacityTaskResponse = StartCapacityTaskResponse(this)
/**
* construct an [aws.sdk.kotlin.services.outposts.model.CapacityTaskFailure] inside the given [block]
*/
public fun failed(block: aws.sdk.kotlin.services.outposts.model.CapacityTaskFailure.Builder.() -> kotlin.Unit) {
this.failed = aws.sdk.kotlin.services.outposts.model.CapacityTaskFailure.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.outposts.model.InstancesToExclude] inside the given [block]
*/
public fun instancesToExclude(block: aws.sdk.kotlin.services.outposts.model.InstancesToExclude.Builder.() -> kotlin.Unit) {
this.instancesToExclude = aws.sdk.kotlin.services.outposts.model.InstancesToExclude.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy