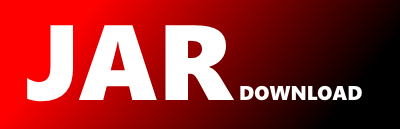
commonMain.aws.sdk.kotlin.services.outposts.model.UpdateSiteRackPhysicalPropertiesRequest.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of outposts-jvm Show documentation
Show all versions of outposts-jvm Show documentation
The AWS SDK for Kotlin client for Outposts
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.outposts.model
import aws.smithy.kotlin.runtime.SdkDsl
public class UpdateSiteRackPhysicalPropertiesRequest private constructor(builder: Builder) {
/**
* The type of fiber that you will use to attach the Outpost to your network.
*/
public val fiberOpticCableType: aws.sdk.kotlin.services.outposts.model.FiberOpticCableType? = builder.fiberOpticCableType
/**
* The maximum rack weight that this site can support. `NO_LIMIT` is over 2000lbs.
*/
public val maximumSupportedWeightLbs: aws.sdk.kotlin.services.outposts.model.MaximumSupportedWeightLbs? = builder.maximumSupportedWeightLbs
/**
* The type of optical standard that you will use to attach the Outpost to your network. This field is dependent on uplink speed, fiber type, and distance to the upstream device. For more information about networking requirements for racks, see [Network](https://docs.aws.amazon.com/outposts/latest/userguide/outposts-requirements.html#facility-networking) in the Amazon Web Services Outposts User Guide.
* + `OPTIC_10GBASE_SR`: 10GBASE-SR
* + `OPTIC_10GBASE_IR`: 10GBASE-IR
* + `OPTIC_10GBASE_LR`: 10GBASE-LR
* + `OPTIC_40GBASE_SR`: 40GBASE-SR
* + `OPTIC_40GBASE_ESR`: 40GBASE-ESR
* + `OPTIC_40GBASE_IR4_LR4L`: 40GBASE-IR (LR4L)
* + `OPTIC_40GBASE_LR4`: 40GBASE-LR4
* + `OPTIC_100GBASE_SR4`: 100GBASE-SR4
* + `OPTIC_100GBASE_CWDM4`: 100GBASE-CWDM4
* + `OPTIC_100GBASE_LR4`: 100GBASE-LR4
* + `OPTIC_100G_PSM4_MSA`: 100G PSM4 MSA
* + `OPTIC_1000BASE_LX`: 1000Base-LX
* + `OPTIC_1000BASE_SX` : 1000Base-SX
*/
public val opticalStandard: aws.sdk.kotlin.services.outposts.model.OpticalStandard? = builder.opticalStandard
/**
* The power connector that Amazon Web Services should plan to provide for connections to the hardware. Note the correlation between `PowerPhase` and `PowerConnector`.
* + Single-phase AC feed
* + **L6-30P** – (common in US); 30A; single phase
* + **IEC309 (blue)** – P+N+E, 6hr; 32 A; single phase
* + Three-phase AC feed
* + **AH530P7W (red)** – 3P+N+E, 7hr; 30A; three phase
* + **AH532P6W (red)** – 3P+N+E, 6hr; 32A; three phase
*/
public val powerConnector: aws.sdk.kotlin.services.outposts.model.PowerConnector? = builder.powerConnector
/**
* The power draw, in kVA, available at the hardware placement position for the rack.
*/
public val powerDrawKva: aws.sdk.kotlin.services.outposts.model.PowerDrawKva? = builder.powerDrawKva
/**
* Indicates whether the power feed comes above or below the rack.
*/
public val powerFeedDrop: aws.sdk.kotlin.services.outposts.model.PowerFeedDrop? = builder.powerFeedDrop
/**
* The power option that you can provide for hardware.
* + Single-phase AC feed: 200 V to 277 V, 50 Hz or 60 Hz
* + Three-phase AC feed: 346 V to 480 V, 50 Hz or 60 Hz
*/
public val powerPhase: aws.sdk.kotlin.services.outposts.model.PowerPhase? = builder.powerPhase
/**
* The ID or the Amazon Resource Name (ARN) of the site.
*/
public val siteId: kotlin.String? = builder.siteId
/**
* Racks come with two Outpost network devices. Depending on the supported uplink speed at the site, the Outpost network devices provide a variable number of uplinks. Specify the number of uplinks for each Outpost network device that you intend to use to connect the rack to your network. Note the correlation between `UplinkGbps` and `UplinkCount`.
* + 1Gbps - Uplinks available: 1, 2, 4, 6, 8
* + 10Gbps - Uplinks available: 1, 2, 4, 8, 12, 16
* + 40 and 100 Gbps- Uplinks available: 1, 2, 4
*/
public val uplinkCount: aws.sdk.kotlin.services.outposts.model.UplinkCount? = builder.uplinkCount
/**
* The uplink speed the rack should support for the connection to the Region.
*/
public val uplinkGbps: aws.sdk.kotlin.services.outposts.model.UplinkGbps? = builder.uplinkGbps
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.outposts.model.UpdateSiteRackPhysicalPropertiesRequest = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("UpdateSiteRackPhysicalPropertiesRequest(")
append("fiberOpticCableType=$fiberOpticCableType,")
append("maximumSupportedWeightLbs=$maximumSupportedWeightLbs,")
append("opticalStandard=$opticalStandard,")
append("powerConnector=$powerConnector,")
append("powerDrawKva=$powerDrawKva,")
append("powerFeedDrop=$powerFeedDrop,")
append("powerPhase=$powerPhase,")
append("siteId=$siteId,")
append("uplinkCount=$uplinkCount,")
append("uplinkGbps=$uplinkGbps")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = fiberOpticCableType?.hashCode() ?: 0
result = 31 * result + (maximumSupportedWeightLbs?.hashCode() ?: 0)
result = 31 * result + (opticalStandard?.hashCode() ?: 0)
result = 31 * result + (powerConnector?.hashCode() ?: 0)
result = 31 * result + (powerDrawKva?.hashCode() ?: 0)
result = 31 * result + (powerFeedDrop?.hashCode() ?: 0)
result = 31 * result + (powerPhase?.hashCode() ?: 0)
result = 31 * result + (siteId?.hashCode() ?: 0)
result = 31 * result + (uplinkCount?.hashCode() ?: 0)
result = 31 * result + (uplinkGbps?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as UpdateSiteRackPhysicalPropertiesRequest
if (fiberOpticCableType != other.fiberOpticCableType) return false
if (maximumSupportedWeightLbs != other.maximumSupportedWeightLbs) return false
if (opticalStandard != other.opticalStandard) return false
if (powerConnector != other.powerConnector) return false
if (powerDrawKva != other.powerDrawKva) return false
if (powerFeedDrop != other.powerFeedDrop) return false
if (powerPhase != other.powerPhase) return false
if (siteId != other.siteId) return false
if (uplinkCount != other.uplinkCount) return false
if (uplinkGbps != other.uplinkGbps) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.outposts.model.UpdateSiteRackPhysicalPropertiesRequest = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The type of fiber that you will use to attach the Outpost to your network.
*/
public var fiberOpticCableType: aws.sdk.kotlin.services.outposts.model.FiberOpticCableType? = null
/**
* The maximum rack weight that this site can support. `NO_LIMIT` is over 2000lbs.
*/
public var maximumSupportedWeightLbs: aws.sdk.kotlin.services.outposts.model.MaximumSupportedWeightLbs? = null
/**
* The type of optical standard that you will use to attach the Outpost to your network. This field is dependent on uplink speed, fiber type, and distance to the upstream device. For more information about networking requirements for racks, see [Network](https://docs.aws.amazon.com/outposts/latest/userguide/outposts-requirements.html#facility-networking) in the Amazon Web Services Outposts User Guide.
* + `OPTIC_10GBASE_SR`: 10GBASE-SR
* + `OPTIC_10GBASE_IR`: 10GBASE-IR
* + `OPTIC_10GBASE_LR`: 10GBASE-LR
* + `OPTIC_40GBASE_SR`: 40GBASE-SR
* + `OPTIC_40GBASE_ESR`: 40GBASE-ESR
* + `OPTIC_40GBASE_IR4_LR4L`: 40GBASE-IR (LR4L)
* + `OPTIC_40GBASE_LR4`: 40GBASE-LR4
* + `OPTIC_100GBASE_SR4`: 100GBASE-SR4
* + `OPTIC_100GBASE_CWDM4`: 100GBASE-CWDM4
* + `OPTIC_100GBASE_LR4`: 100GBASE-LR4
* + `OPTIC_100G_PSM4_MSA`: 100G PSM4 MSA
* + `OPTIC_1000BASE_LX`: 1000Base-LX
* + `OPTIC_1000BASE_SX` : 1000Base-SX
*/
public var opticalStandard: aws.sdk.kotlin.services.outposts.model.OpticalStandard? = null
/**
* The power connector that Amazon Web Services should plan to provide for connections to the hardware. Note the correlation between `PowerPhase` and `PowerConnector`.
* + Single-phase AC feed
* + **L6-30P** – (common in US); 30A; single phase
* + **IEC309 (blue)** – P+N+E, 6hr; 32 A; single phase
* + Three-phase AC feed
* + **AH530P7W (red)** – 3P+N+E, 7hr; 30A; three phase
* + **AH532P6W (red)** – 3P+N+E, 6hr; 32A; three phase
*/
public var powerConnector: aws.sdk.kotlin.services.outposts.model.PowerConnector? = null
/**
* The power draw, in kVA, available at the hardware placement position for the rack.
*/
public var powerDrawKva: aws.sdk.kotlin.services.outposts.model.PowerDrawKva? = null
/**
* Indicates whether the power feed comes above or below the rack.
*/
public var powerFeedDrop: aws.sdk.kotlin.services.outposts.model.PowerFeedDrop? = null
/**
* The power option that you can provide for hardware.
* + Single-phase AC feed: 200 V to 277 V, 50 Hz or 60 Hz
* + Three-phase AC feed: 346 V to 480 V, 50 Hz or 60 Hz
*/
public var powerPhase: aws.sdk.kotlin.services.outposts.model.PowerPhase? = null
/**
* The ID or the Amazon Resource Name (ARN) of the site.
*/
public var siteId: kotlin.String? = null
/**
* Racks come with two Outpost network devices. Depending on the supported uplink speed at the site, the Outpost network devices provide a variable number of uplinks. Specify the number of uplinks for each Outpost network device that you intend to use to connect the rack to your network. Note the correlation between `UplinkGbps` and `UplinkCount`.
* + 1Gbps - Uplinks available: 1, 2, 4, 6, 8
* + 10Gbps - Uplinks available: 1, 2, 4, 8, 12, 16
* + 40 and 100 Gbps- Uplinks available: 1, 2, 4
*/
public var uplinkCount: aws.sdk.kotlin.services.outposts.model.UplinkCount? = null
/**
* The uplink speed the rack should support for the connection to the Region.
*/
public var uplinkGbps: aws.sdk.kotlin.services.outposts.model.UplinkGbps? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.outposts.model.UpdateSiteRackPhysicalPropertiesRequest) : this() {
this.fiberOpticCableType = x.fiberOpticCableType
this.maximumSupportedWeightLbs = x.maximumSupportedWeightLbs
this.opticalStandard = x.opticalStandard
this.powerConnector = x.powerConnector
this.powerDrawKva = x.powerDrawKva
this.powerFeedDrop = x.powerFeedDrop
this.powerPhase = x.powerPhase
this.siteId = x.siteId
this.uplinkCount = x.uplinkCount
this.uplinkGbps = x.uplinkGbps
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.outposts.model.UpdateSiteRackPhysicalPropertiesRequest = UpdateSiteRackPhysicalPropertiesRequest(this)
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy