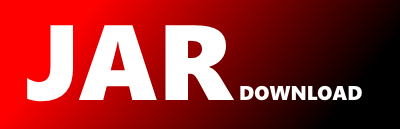
commonMain.aws.sdk.kotlin.services.proton.DefaultProtonClient.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of proton-jvm Show documentation
Show all versions of proton-jvm Show documentation
The AWS SDK for Kotlin client for Proton
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.proton
import aws.sdk.kotlin.runtime.http.ApiMetadata
import aws.sdk.kotlin.runtime.http.AwsUserAgentMetadata
import aws.sdk.kotlin.runtime.http.interceptors.AwsSpanInterceptor
import aws.sdk.kotlin.runtime.http.interceptors.BusinessMetricsInterceptor
import aws.sdk.kotlin.runtime.http.middleware.AwsRetryHeaderMiddleware
import aws.sdk.kotlin.runtime.http.middleware.RecursionDetection
import aws.sdk.kotlin.runtime.http.middleware.UserAgent
import aws.sdk.kotlin.services.proton.auth.ProtonAuthSchemeProviderAdapter
import aws.sdk.kotlin.services.proton.auth.ProtonIdentityProviderConfigAdapter
import aws.sdk.kotlin.services.proton.endpoints.internal.EndpointResolverAdapter
import aws.sdk.kotlin.services.proton.model.*
import aws.sdk.kotlin.services.proton.serde.*
import aws.smithy.kotlin.runtime.auth.AuthSchemeId
import aws.smithy.kotlin.runtime.auth.awssigning.AwsSigningAttributes
import aws.smithy.kotlin.runtime.auth.awssigning.DefaultAwsSigner
import aws.smithy.kotlin.runtime.awsprotocol.AwsAttributes
import aws.smithy.kotlin.runtime.awsprotocol.json.AwsJsonProtocol
import aws.smithy.kotlin.runtime.client.SdkClientOption
import aws.smithy.kotlin.runtime.collections.attributesOf
import aws.smithy.kotlin.runtime.collections.putIfAbsent
import aws.smithy.kotlin.runtime.collections.putIfAbsentNotNull
import aws.smithy.kotlin.runtime.http.SdkHttpClient
import aws.smithy.kotlin.runtime.http.auth.AuthScheme
import aws.smithy.kotlin.runtime.http.auth.SigV4AuthScheme
import aws.smithy.kotlin.runtime.http.operation.OperationAuthConfig
import aws.smithy.kotlin.runtime.http.operation.OperationMetrics
import aws.smithy.kotlin.runtime.http.operation.SdkHttpOperation
import aws.smithy.kotlin.runtime.http.operation.context
import aws.smithy.kotlin.runtime.http.operation.roundTrip
import aws.smithy.kotlin.runtime.http.operation.telemetry
import aws.smithy.kotlin.runtime.io.SdkManagedGroup
import aws.smithy.kotlin.runtime.io.addIfManaged
import aws.smithy.kotlin.runtime.operation.ExecutionContext
internal class DefaultProtonClient(override val config: ProtonClient.Config) : ProtonClient {
private val managedResources = SdkManagedGroup()
private val client = SdkHttpClient(config.httpClient)
private val identityProviderConfig = ProtonIdentityProviderConfigAdapter(config)
private val configuredAuthSchemes = with(config.authSchemes.associateBy(AuthScheme::schemeId).toMutableMap()){
getOrPut(AuthSchemeId.AwsSigV4){
SigV4AuthScheme(DefaultAwsSigner, "proton")
}
toMap()
}
private val authSchemeAdapter = ProtonAuthSchemeProviderAdapter(config)
private val telemetryScope = "aws.sdk.kotlin.services.proton"
private val opMetrics = OperationMetrics(telemetryScope, config.telemetryProvider)
init {
managedResources.addIfManaged(config.httpClient)
managedResources.addIfManaged(config.credentialsProvider)
}
private val awsUserAgentMetadata = AwsUserAgentMetadata.fromEnvironment(ApiMetadata(ServiceId, SdkVersion), config.applicationId)
/**
* In a management account, an environment account connection request is accepted. When the environment account connection request is accepted, Proton can use the associated IAM role to provision environment infrastructure resources in the associated environment account.
*
* For more information, see [Environment account connections](https://docs.aws.amazon.com/proton/latest/userguide/ag-env-account-connections.html) in the *Proton User guide*.
*/
override suspend fun acceptEnvironmentAccountConnection(input: AcceptEnvironmentAccountConnectionRequest): AcceptEnvironmentAccountConnectionResponse {
val op = SdkHttpOperation.build {
serializeWith = AcceptEnvironmentAccountConnectionOperationSerializer()
deserializeWith = AcceptEnvironmentAccountConnectionOperationDeserializer()
operationName = "AcceptEnvironmentAccountConnection"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Attempts to cancel a component deployment (for a component that is in the `IN_PROGRESS` deployment status).
*
* For more information about components, see [Proton components](https://docs.aws.amazon.com/proton/latest/userguide/ag-components.html) in the *Proton User Guide*.
*/
override suspend fun cancelComponentDeployment(input: CancelComponentDeploymentRequest): CancelComponentDeploymentResponse {
val op = SdkHttpOperation.build {
serializeWith = CancelComponentDeploymentOperationSerializer()
deserializeWith = CancelComponentDeploymentOperationDeserializer()
operationName = "CancelComponentDeployment"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Attempts to cancel an environment deployment on an UpdateEnvironment action, if the deployment is `IN_PROGRESS`. For more information, see [Update an environment](https://docs.aws.amazon.com/proton/latest/userguide/ag-env-update.html) in the *Proton User guide*.
*
* The following list includes potential cancellation scenarios.
* + If the cancellation attempt succeeds, the resulting deployment state is `CANCELLED`.
* + If the cancellation attempt fails, the resulting deployment state is `FAILED`.
* + If the current UpdateEnvironment action succeeds before the cancellation attempt starts, the resulting deployment state is `SUCCEEDED` and the cancellation attempt has no effect.
*/
override suspend fun cancelEnvironmentDeployment(input: CancelEnvironmentDeploymentRequest): CancelEnvironmentDeploymentResponse {
val op = SdkHttpOperation.build {
serializeWith = CancelEnvironmentDeploymentOperationSerializer()
deserializeWith = CancelEnvironmentDeploymentOperationDeserializer()
operationName = "CancelEnvironmentDeployment"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Attempts to cancel a service instance deployment on an UpdateServiceInstance action, if the deployment is `IN_PROGRESS`. For more information, see [Update a service instance](https://docs.aws.amazon.com/proton/latest/userguide/ag-svc-instance-update.html) in the *Proton User guide*.
*
* The following list includes potential cancellation scenarios.
* + If the cancellation attempt succeeds, the resulting deployment state is `CANCELLED`.
* + If the cancellation attempt fails, the resulting deployment state is `FAILED`.
* + If the current UpdateServiceInstance action succeeds before the cancellation attempt starts, the resulting deployment state is `SUCCEEDED` and the cancellation attempt has no effect.
*/
override suspend fun cancelServiceInstanceDeployment(input: CancelServiceInstanceDeploymentRequest): CancelServiceInstanceDeploymentResponse {
val op = SdkHttpOperation.build {
serializeWith = CancelServiceInstanceDeploymentOperationSerializer()
deserializeWith = CancelServiceInstanceDeploymentOperationDeserializer()
operationName = "CancelServiceInstanceDeployment"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Attempts to cancel a service pipeline deployment on an UpdateServicePipeline action, if the deployment is `IN_PROGRESS`. For more information, see [Update a service pipeline](https://docs.aws.amazon.com/proton/latest/userguide/ag-svc-pipeline-update.html) in the *Proton User guide*.
*
* The following list includes potential cancellation scenarios.
* + If the cancellation attempt succeeds, the resulting deployment state is `CANCELLED`.
* + If the cancellation attempt fails, the resulting deployment state is `FAILED`.
* + If the current UpdateServicePipeline action succeeds before the cancellation attempt starts, the resulting deployment state is `SUCCEEDED` and the cancellation attempt has no effect.
*/
override suspend fun cancelServicePipelineDeployment(input: CancelServicePipelineDeploymentRequest): CancelServicePipelineDeploymentResponse {
val op = SdkHttpOperation.build {
serializeWith = CancelServicePipelineDeploymentOperationSerializer()
deserializeWith = CancelServicePipelineDeploymentOperationDeserializer()
operationName = "CancelServicePipelineDeployment"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Create an Proton component. A component is an infrastructure extension for a service instance.
*
* For more information about components, see [Proton components](https://docs.aws.amazon.com/proton/latest/userguide/ag-components.html) in the *Proton User Guide*.
*/
override suspend fun createComponent(input: CreateComponentRequest): CreateComponentResponse {
val op = SdkHttpOperation.build {
serializeWith = CreateComponentOperationSerializer()
deserializeWith = CreateComponentOperationDeserializer()
operationName = "CreateComponent"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Deploy a new environment. An Proton environment is created from an environment template that defines infrastructure and resources that can be shared across services.
*
* **You can provision environments using the following methods:**
* + Amazon Web Services-managed provisioning: Proton makes direct calls to provision your resources.
* + Self-managed provisioning: Proton makes pull requests on your repository to provide compiled infrastructure as code (IaC) files that your IaC engine uses to provision resources.
*
* For more information, see [Environments](https://docs.aws.amazon.com/proton/latest/userguide/ag-environments.html) and [Provisioning methods](https://docs.aws.amazon.com/proton/latest/userguide/ag-works-prov-methods.html) in the *Proton User Guide*.
*/
override suspend fun createEnvironment(input: CreateEnvironmentRequest): CreateEnvironmentResponse {
val op = SdkHttpOperation.build {
serializeWith = CreateEnvironmentOperationSerializer()
deserializeWith = CreateEnvironmentOperationDeserializer()
operationName = "CreateEnvironment"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Create an environment account connection in an environment account so that environment infrastructure resources can be provisioned in the environment account from a management account.
*
* An environment account connection is a secure bi-directional connection between a *management account* and an *environment account* that maintains authorization and permissions. For more information, see [Environment account connections](https://docs.aws.amazon.com/proton/latest/userguide/ag-env-account-connections.html) in the *Proton User guide*.
*/
override suspend fun createEnvironmentAccountConnection(input: CreateEnvironmentAccountConnectionRequest): CreateEnvironmentAccountConnectionResponse {
val op = SdkHttpOperation.build {
serializeWith = CreateEnvironmentAccountConnectionOperationSerializer()
deserializeWith = CreateEnvironmentAccountConnectionOperationDeserializer()
operationName = "CreateEnvironmentAccountConnection"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Create an environment template for Proton. For more information, see [Environment Templates](https://docs.aws.amazon.com/proton/latest/userguide/ag-templates.html) in the *Proton User Guide*.
*
* You can create an environment template in one of the two following ways:
* + Register and publish a *standard* environment template that instructs Proton to deploy and manage environment infrastructure.
* + Register and publish a *customer managed* environment template that connects Proton to your existing provisioned infrastructure that you manage. Proton *doesn't* manage your existing provisioned infrastructure. To create an environment template for customer provisioned and managed infrastructure, include the `provisioning` parameter and set the value to `CUSTOMER_MANAGED`. For more information, see [Register and publish an environment template](https://docs.aws.amazon.com/proton/latest/userguide/template-create.html) in the *Proton User Guide*.
*/
override suspend fun createEnvironmentTemplate(input: CreateEnvironmentTemplateRequest): CreateEnvironmentTemplateResponse {
val op = SdkHttpOperation.build {
serializeWith = CreateEnvironmentTemplateOperationSerializer()
deserializeWith = CreateEnvironmentTemplateOperationDeserializer()
operationName = "CreateEnvironmentTemplate"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Create a new major or minor version of an environment template. A major version of an environment template is a version that *isn't* backwards compatible. A minor version of an environment template is a version that's backwards compatible within its major version.
*/
override suspend fun createEnvironmentTemplateVersion(input: CreateEnvironmentTemplateVersionRequest): CreateEnvironmentTemplateVersionResponse {
val op = SdkHttpOperation.build {
serializeWith = CreateEnvironmentTemplateVersionOperationSerializer()
deserializeWith = CreateEnvironmentTemplateVersionOperationDeserializer()
operationName = "CreateEnvironmentTemplateVersion"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Create and register a link to a repository. Proton uses the link to repeatedly access the repository, to either push to it (self-managed provisioning) or pull from it (template sync). You can share a linked repository across multiple resources (like environments using self-managed provisioning, or synced templates). When you create a repository link, Proton creates a [service-linked role](https://docs.aws.amazon.com/proton/latest/userguide/using-service-linked-roles.html) for you.
*
* For more information, see [Self-managed provisioning](https://docs.aws.amazon.com/proton/latest/userguide/ag-works-prov-methods.html#ag-works-prov-methods-self), [Template bundles](https://docs.aws.amazon.com/proton/latest/userguide/ag-template-authoring.html#ag-template-bundles), and [Template sync configurations](https://docs.aws.amazon.com/proton/latest/userguide/ag-template-sync-configs.html) in the *Proton User Guide*.
*/
override suspend fun createRepository(input: CreateRepositoryRequest): CreateRepositoryResponse {
val op = SdkHttpOperation.build {
serializeWith = CreateRepositoryOperationSerializer()
deserializeWith = CreateRepositoryOperationDeserializer()
operationName = "CreateRepository"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Create an Proton service. An Proton service is an instantiation of a service template and often includes several service instances and pipeline. For more information, see [Services](https://docs.aws.amazon.com/proton/latest/userguide/ag-services.html) in the *Proton User Guide*.
*/
override suspend fun createService(input: CreateServiceRequest): CreateServiceResponse {
val op = SdkHttpOperation.build {
serializeWith = CreateServiceOperationSerializer()
deserializeWith = CreateServiceOperationDeserializer()
operationName = "CreateService"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Create a service instance.
*/
override suspend fun createServiceInstance(input: CreateServiceInstanceRequest): CreateServiceInstanceResponse {
val op = SdkHttpOperation.build {
serializeWith = CreateServiceInstanceOperationSerializer()
deserializeWith = CreateServiceInstanceOperationDeserializer()
operationName = "CreateServiceInstance"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Create the Proton Ops configuration file.
*/
override suspend fun createServiceSyncConfig(input: CreateServiceSyncConfigRequest): CreateServiceSyncConfigResponse {
val op = SdkHttpOperation.build {
serializeWith = CreateServiceSyncConfigOperationSerializer()
deserializeWith = CreateServiceSyncConfigOperationDeserializer()
operationName = "CreateServiceSyncConfig"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Create a service template. The administrator creates a service template to define standardized infrastructure and an optional CI/CD service pipeline. Developers, in turn, select the service template from Proton. If the selected service template includes a service pipeline definition, they provide a link to their source code repository. Proton then deploys and manages the infrastructure defined by the selected service template. For more information, see [Proton templates](https://docs.aws.amazon.com/proton/latest/userguide/ag-templates.html) in the *Proton User Guide*.
*/
override suspend fun createServiceTemplate(input: CreateServiceTemplateRequest): CreateServiceTemplateResponse {
val op = SdkHttpOperation.build {
serializeWith = CreateServiceTemplateOperationSerializer()
deserializeWith = CreateServiceTemplateOperationDeserializer()
operationName = "CreateServiceTemplate"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Create a new major or minor version of a service template. A major version of a service template is a version that *isn't* backward compatible. A minor version of a service template is a version that's backward compatible within its major version.
*/
override suspend fun createServiceTemplateVersion(input: CreateServiceTemplateVersionRequest): CreateServiceTemplateVersionResponse {
val op = SdkHttpOperation.build {
serializeWith = CreateServiceTemplateVersionOperationSerializer()
deserializeWith = CreateServiceTemplateVersionOperationDeserializer()
operationName = "CreateServiceTemplateVersion"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Set up a template to create new template versions automatically by tracking a linked repository. A linked repository is a repository that has been registered with Proton. For more information, see CreateRepository.
*
* When a commit is pushed to your linked repository, Proton checks for changes to your repository template bundles. If it detects a template bundle change, a new major or minor version of its template is created, if the version doesn’t already exist. For more information, see [Template sync configurations](https://docs.aws.amazon.com/proton/latest/userguide/ag-template-sync-configs.html) in the *Proton User Guide*.
*/
override suspend fun createTemplateSyncConfig(input: CreateTemplateSyncConfigRequest): CreateTemplateSyncConfigResponse {
val op = SdkHttpOperation.build {
serializeWith = CreateTemplateSyncConfigOperationSerializer()
deserializeWith = CreateTemplateSyncConfigOperationDeserializer()
operationName = "CreateTemplateSyncConfig"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Delete an Proton component resource.
*
* For more information about components, see [Proton components](https://docs.aws.amazon.com/proton/latest/userguide/ag-components.html) in the *Proton User Guide*.
*/
override suspend fun deleteComponent(input: DeleteComponentRequest): DeleteComponentResponse {
val op = SdkHttpOperation.build {
serializeWith = DeleteComponentOperationSerializer()
deserializeWith = DeleteComponentOperationDeserializer()
operationName = "DeleteComponent"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Delete the deployment.
*/
override suspend fun deleteDeployment(input: DeleteDeploymentRequest): DeleteDeploymentResponse {
val op = SdkHttpOperation.build {
serializeWith = DeleteDeploymentOperationSerializer()
deserializeWith = DeleteDeploymentOperationDeserializer()
operationName = "DeleteDeployment"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Delete an environment.
*/
override suspend fun deleteEnvironment(input: DeleteEnvironmentRequest): DeleteEnvironmentResponse {
val op = SdkHttpOperation.build {
serializeWith = DeleteEnvironmentOperationSerializer()
deserializeWith = DeleteEnvironmentOperationDeserializer()
operationName = "DeleteEnvironment"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* In an environment account, delete an environment account connection.
*
* After you delete an environment account connection that’s in use by an Proton environment, Proton *can’t* manage the environment infrastructure resources until a new environment account connection is accepted for the environment account and associated environment. You're responsible for cleaning up provisioned resources that remain without an environment connection.
*
* For more information, see [Environment account connections](https://docs.aws.amazon.com/proton/latest/userguide/ag-env-account-connections.html) in the *Proton User guide*.
*/
override suspend fun deleteEnvironmentAccountConnection(input: DeleteEnvironmentAccountConnectionRequest): DeleteEnvironmentAccountConnectionResponse {
val op = SdkHttpOperation.build {
serializeWith = DeleteEnvironmentAccountConnectionOperationSerializer()
deserializeWith = DeleteEnvironmentAccountConnectionOperationDeserializer()
operationName = "DeleteEnvironmentAccountConnection"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* If no other major or minor versions of an environment template exist, delete the environment template.
*/
override suspend fun deleteEnvironmentTemplate(input: DeleteEnvironmentTemplateRequest): DeleteEnvironmentTemplateResponse {
val op = SdkHttpOperation.build {
serializeWith = DeleteEnvironmentTemplateOperationSerializer()
deserializeWith = DeleteEnvironmentTemplateOperationDeserializer()
operationName = "DeleteEnvironmentTemplate"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* If no other minor versions of an environment template exist, delete a major version of the environment template if it's not the `Recommended` version. Delete the `Recommended` version of the environment template if no other major versions or minor versions of the environment template exist. A major version of an environment template is a version that's not backward compatible.
*
* Delete a minor version of an environment template if it *isn't* the `Recommended` version. Delete a `Recommended` minor version of the environment template if no other minor versions of the environment template exist. A minor version of an environment template is a version that's backward compatible.
*/
override suspend fun deleteEnvironmentTemplateVersion(input: DeleteEnvironmentTemplateVersionRequest): DeleteEnvironmentTemplateVersionResponse {
val op = SdkHttpOperation.build {
serializeWith = DeleteEnvironmentTemplateVersionOperationSerializer()
deserializeWith = DeleteEnvironmentTemplateVersionOperationDeserializer()
operationName = "DeleteEnvironmentTemplateVersion"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* De-register and unlink your repository.
*/
override suspend fun deleteRepository(input: DeleteRepositoryRequest): DeleteRepositoryResponse {
val op = SdkHttpOperation.build {
serializeWith = DeleteRepositoryOperationSerializer()
deserializeWith = DeleteRepositoryOperationDeserializer()
operationName = "DeleteRepository"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Delete a service, with its instances and pipeline.
*
* You can't delete a service if it has any service instances that have components attached to them.
*
* For more information about components, see [Proton components](https://docs.aws.amazon.com/proton/latest/userguide/ag-components.html) in the *Proton User Guide*.
*/
override suspend fun deleteService(input: DeleteServiceRequest): DeleteServiceResponse {
val op = SdkHttpOperation.build {
serializeWith = DeleteServiceOperationSerializer()
deserializeWith = DeleteServiceOperationDeserializer()
operationName = "DeleteService"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Delete the Proton Ops file.
*/
override suspend fun deleteServiceSyncConfig(input: DeleteServiceSyncConfigRequest): DeleteServiceSyncConfigResponse {
val op = SdkHttpOperation.build {
serializeWith = DeleteServiceSyncConfigOperationSerializer()
deserializeWith = DeleteServiceSyncConfigOperationDeserializer()
operationName = "DeleteServiceSyncConfig"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* If no other major or minor versions of the service template exist, delete the service template.
*/
override suspend fun deleteServiceTemplate(input: DeleteServiceTemplateRequest): DeleteServiceTemplateResponse {
val op = SdkHttpOperation.build {
serializeWith = DeleteServiceTemplateOperationSerializer()
deserializeWith = DeleteServiceTemplateOperationDeserializer()
operationName = "DeleteServiceTemplate"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* If no other minor versions of a service template exist, delete a major version of the service template if it's not the `Recommended` version. Delete the `Recommended` version of the service template if no other major versions or minor versions of the service template exist. A major version of a service template is a version that *isn't* backwards compatible.
*
* Delete a minor version of a service template if it's not the `Recommended` version. Delete a `Recommended` minor version of the service template if no other minor versions of the service template exist. A minor version of a service template is a version that's backwards compatible.
*/
override suspend fun deleteServiceTemplateVersion(input: DeleteServiceTemplateVersionRequest): DeleteServiceTemplateVersionResponse {
val op = SdkHttpOperation.build {
serializeWith = DeleteServiceTemplateVersionOperationSerializer()
deserializeWith = DeleteServiceTemplateVersionOperationDeserializer()
operationName = "DeleteServiceTemplateVersion"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Delete a template sync configuration.
*/
override suspend fun deleteTemplateSyncConfig(input: DeleteTemplateSyncConfigRequest): DeleteTemplateSyncConfigResponse {
val op = SdkHttpOperation.build {
serializeWith = DeleteTemplateSyncConfigOperationSerializer()
deserializeWith = DeleteTemplateSyncConfigOperationDeserializer()
operationName = "DeleteTemplateSyncConfig"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Get detail data for Proton account-wide settings.
*/
override suspend fun getAccountSettings(input: GetAccountSettingsRequest): GetAccountSettingsResponse {
val op = SdkHttpOperation.build {
serializeWith = GetAccountSettingsOperationSerializer()
deserializeWith = GetAccountSettingsOperationDeserializer()
operationName = "GetAccountSettings"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Get detailed data for a component.
*
* For more information about components, see [Proton components](https://docs.aws.amazon.com/proton/latest/userguide/ag-components.html) in the *Proton User Guide*.
*/
override suspend fun getComponent(input: GetComponentRequest): GetComponentResponse {
val op = SdkHttpOperation.build {
serializeWith = GetComponentOperationSerializer()
deserializeWith = GetComponentOperationDeserializer()
operationName = "GetComponent"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Get detailed data for a deployment.
*/
override suspend fun getDeployment(input: GetDeploymentRequest): GetDeploymentResponse {
val op = SdkHttpOperation.build {
serializeWith = GetDeploymentOperationSerializer()
deserializeWith = GetDeploymentOperationDeserializer()
operationName = "GetDeployment"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Get detailed data for an environment.
*/
override suspend fun getEnvironment(input: GetEnvironmentRequest): GetEnvironmentResponse {
val op = SdkHttpOperation.build {
serializeWith = GetEnvironmentOperationSerializer()
deserializeWith = GetEnvironmentOperationDeserializer()
operationName = "GetEnvironment"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* In an environment account, get the detailed data for an environment account connection.
*
* For more information, see [Environment account connections](https://docs.aws.amazon.com/proton/latest/userguide/ag-env-account-connections.html) in the *Proton User guide*.
*/
override suspend fun getEnvironmentAccountConnection(input: GetEnvironmentAccountConnectionRequest): GetEnvironmentAccountConnectionResponse {
val op = SdkHttpOperation.build {
serializeWith = GetEnvironmentAccountConnectionOperationSerializer()
deserializeWith = GetEnvironmentAccountConnectionOperationDeserializer()
operationName = "GetEnvironmentAccountConnection"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Get detailed data for an environment template.
*/
override suspend fun getEnvironmentTemplate(input: GetEnvironmentTemplateRequest): GetEnvironmentTemplateResponse {
val op = SdkHttpOperation.build {
serializeWith = GetEnvironmentTemplateOperationSerializer()
deserializeWith = GetEnvironmentTemplateOperationDeserializer()
operationName = "GetEnvironmentTemplate"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Get detailed data for a major or minor version of an environment template.
*/
override suspend fun getEnvironmentTemplateVersion(input: GetEnvironmentTemplateVersionRequest): GetEnvironmentTemplateVersionResponse {
val op = SdkHttpOperation.build {
serializeWith = GetEnvironmentTemplateVersionOperationSerializer()
deserializeWith = GetEnvironmentTemplateVersionOperationDeserializer()
operationName = "GetEnvironmentTemplateVersion"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Get detail data for a linked repository.
*/
override suspend fun getRepository(input: GetRepositoryRequest): GetRepositoryResponse {
val op = SdkHttpOperation.build {
serializeWith = GetRepositoryOperationSerializer()
deserializeWith = GetRepositoryOperationDeserializer()
operationName = "GetRepository"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Get the sync status of a repository used for Proton template sync. For more information about template sync, see .
*
* A repository sync status isn't tied to the Proton Repository resource (or any other Proton resource). Therefore, tags on an Proton Repository resource have no effect on this action. Specifically, you can't use these tags to control access to this action using Attribute-based access control (ABAC).
*
* For more information about ABAC, see [ABAC](https://docs.aws.amazon.com/proton/latest/userguide/security_iam_service-with-iam.html#security_iam_service-with-iam-tags) in the *Proton User Guide*.
*/
override suspend fun getRepositorySyncStatus(input: GetRepositorySyncStatusRequest): GetRepositorySyncStatusResponse {
val op = SdkHttpOperation.build {
serializeWith = GetRepositorySyncStatusOperationSerializer()
deserializeWith = GetRepositorySyncStatusOperationDeserializer()
operationName = "GetRepositorySyncStatus"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Get counts of Proton resources.
*
* For infrastructure-provisioning resources (environments, services, service instances, pipelines), the action returns staleness counts. A resource is stale when it's behind the recommended version of the Proton template that it uses and it needs an update to become current.
*
* The action returns staleness counts (counts of resources that are up-to-date, behind a template major version, or behind a template minor version), the total number of resources, and the number of resources that are in a failed state, grouped by resource type. Components, environments, and service templates return less information - see the `components`, `environments`, and `serviceTemplates` field descriptions.
*
* For context, the action also returns the total number of each type of Proton template in the Amazon Web Services account.
*
* For more information, see [Proton dashboard](https://docs.aws.amazon.com/proton/latest/userguide/monitoring-dashboard.html) in the *Proton User Guide*.
*/
override suspend fun getResourcesSummary(input: GetResourcesSummaryRequest): GetResourcesSummaryResponse {
val op = SdkHttpOperation.build {
serializeWith = GetResourcesSummaryOperationSerializer()
deserializeWith = GetResourcesSummaryOperationDeserializer()
operationName = "GetResourcesSummary"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Get detailed data for a service.
*/
override suspend fun getService(input: GetServiceRequest): GetServiceResponse {
val op = SdkHttpOperation.build {
serializeWith = GetServiceOperationSerializer()
deserializeWith = GetServiceOperationDeserializer()
operationName = "GetService"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Get detailed data for a service instance. A service instance is an instantiation of service template and it runs in a specific environment.
*/
override suspend fun getServiceInstance(input: GetServiceInstanceRequest): GetServiceInstanceResponse {
val op = SdkHttpOperation.build {
serializeWith = GetServiceInstanceOperationSerializer()
deserializeWith = GetServiceInstanceOperationDeserializer()
operationName = "GetServiceInstance"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Get the status of the synced service instance.
*/
override suspend fun getServiceInstanceSyncStatus(input: GetServiceInstanceSyncStatusRequest): GetServiceInstanceSyncStatusResponse {
val op = SdkHttpOperation.build {
serializeWith = GetServiceInstanceSyncStatusOperationSerializer()
deserializeWith = GetServiceInstanceSyncStatusOperationDeserializer()
operationName = "GetServiceInstanceSyncStatus"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Get detailed data for the service sync blocker summary.
*/
override suspend fun getServiceSyncBlockerSummary(input: GetServiceSyncBlockerSummaryRequest): GetServiceSyncBlockerSummaryResponse {
val op = SdkHttpOperation.build {
serializeWith = GetServiceSyncBlockerSummaryOperationSerializer()
deserializeWith = GetServiceSyncBlockerSummaryOperationDeserializer()
operationName = "GetServiceSyncBlockerSummary"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Get detailed information for the service sync configuration.
*/
override suspend fun getServiceSyncConfig(input: GetServiceSyncConfigRequest): GetServiceSyncConfigResponse {
val op = SdkHttpOperation.build {
serializeWith = GetServiceSyncConfigOperationSerializer()
deserializeWith = GetServiceSyncConfigOperationDeserializer()
operationName = "GetServiceSyncConfig"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Get detailed data for a service template.
*/
override suspend fun getServiceTemplate(input: GetServiceTemplateRequest): GetServiceTemplateResponse {
val op = SdkHttpOperation.build {
serializeWith = GetServiceTemplateOperationSerializer()
deserializeWith = GetServiceTemplateOperationDeserializer()
operationName = "GetServiceTemplate"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Get detailed data for a major or minor version of a service template.
*/
override suspend fun getServiceTemplateVersion(input: GetServiceTemplateVersionRequest): GetServiceTemplateVersionResponse {
val op = SdkHttpOperation.build {
serializeWith = GetServiceTemplateVersionOperationSerializer()
deserializeWith = GetServiceTemplateVersionOperationDeserializer()
operationName = "GetServiceTemplateVersion"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Get detail data for a template sync configuration.
*/
override suspend fun getTemplateSyncConfig(input: GetTemplateSyncConfigRequest): GetTemplateSyncConfigResponse {
val op = SdkHttpOperation.build {
serializeWith = GetTemplateSyncConfigOperationSerializer()
deserializeWith = GetTemplateSyncConfigOperationDeserializer()
operationName = "GetTemplateSyncConfig"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Get the status of a template sync.
*/
override suspend fun getTemplateSyncStatus(input: GetTemplateSyncStatusRequest): GetTemplateSyncStatusResponse {
val op = SdkHttpOperation.build {
serializeWith = GetTemplateSyncStatusOperationSerializer()
deserializeWith = GetTemplateSyncStatusOperationDeserializer()
operationName = "GetTemplateSyncStatus"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Get a list of component Infrastructure as Code (IaC) outputs.
*
* For more information about components, see [Proton components](https://docs.aws.amazon.com/proton/latest/userguide/ag-components.html) in the *Proton User Guide*.
*/
override suspend fun listComponentOutputs(input: ListComponentOutputsRequest): ListComponentOutputsResponse {
val op = SdkHttpOperation.build {
serializeWith = ListComponentOutputsOperationSerializer()
deserializeWith = ListComponentOutputsOperationDeserializer()
operationName = "ListComponentOutputs"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* List provisioned resources for a component with details.
*
* For more information about components, see [Proton components](https://docs.aws.amazon.com/proton/latest/userguide/ag-components.html) in the *Proton User Guide*.
*/
override suspend fun listComponentProvisionedResources(input: ListComponentProvisionedResourcesRequest): ListComponentProvisionedResourcesResponse {
val op = SdkHttpOperation.build {
serializeWith = ListComponentProvisionedResourcesOperationSerializer()
deserializeWith = ListComponentProvisionedResourcesOperationDeserializer()
operationName = "ListComponentProvisionedResources"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* List components with summary data. You can filter the result list by environment, service, or a single service instance.
*
* For more information about components, see [Proton components](https://docs.aws.amazon.com/proton/latest/userguide/ag-components.html) in the *Proton User Guide*.
*/
override suspend fun listComponents(input: ListComponentsRequest): ListComponentsResponse {
val op = SdkHttpOperation.build {
serializeWith = ListComponentsOperationSerializer()
deserializeWith = ListComponentsOperationDeserializer()
operationName = "ListComponents"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* List deployments. You can filter the result list by environment, service, or a single service instance.
*/
override suspend fun listDeployments(input: ListDeploymentsRequest): ListDeploymentsResponse {
val op = SdkHttpOperation.build {
serializeWith = ListDeploymentsOperationSerializer()
deserializeWith = ListDeploymentsOperationDeserializer()
operationName = "ListDeployments"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* View a list of environment account connections.
*
* For more information, see [Environment account connections](https://docs.aws.amazon.com/proton/latest/userguide/ag-env-account-connections.html) in the *Proton User guide*.
*/
override suspend fun listEnvironmentAccountConnections(input: ListEnvironmentAccountConnectionsRequest): ListEnvironmentAccountConnectionsResponse {
val op = SdkHttpOperation.build {
serializeWith = ListEnvironmentAccountConnectionsOperationSerializer()
deserializeWith = ListEnvironmentAccountConnectionsOperationDeserializer()
operationName = "ListEnvironmentAccountConnections"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* List the infrastructure as code outputs for your environment.
*/
override suspend fun listEnvironmentOutputs(input: ListEnvironmentOutputsRequest): ListEnvironmentOutputsResponse {
val op = SdkHttpOperation.build {
serializeWith = ListEnvironmentOutputsOperationSerializer()
deserializeWith = ListEnvironmentOutputsOperationDeserializer()
operationName = "ListEnvironmentOutputs"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* List the provisioned resources for your environment.
*/
override suspend fun listEnvironmentProvisionedResources(input: ListEnvironmentProvisionedResourcesRequest): ListEnvironmentProvisionedResourcesResponse {
val op = SdkHttpOperation.build {
serializeWith = ListEnvironmentProvisionedResourcesOperationSerializer()
deserializeWith = ListEnvironmentProvisionedResourcesOperationDeserializer()
operationName = "ListEnvironmentProvisionedResources"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* List major or minor versions of an environment template with detail data.
*/
override suspend fun listEnvironmentTemplateVersions(input: ListEnvironmentTemplateVersionsRequest): ListEnvironmentTemplateVersionsResponse {
val op = SdkHttpOperation.build {
serializeWith = ListEnvironmentTemplateVersionsOperationSerializer()
deserializeWith = ListEnvironmentTemplateVersionsOperationDeserializer()
operationName = "ListEnvironmentTemplateVersions"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* List environment templates.
*/
override suspend fun listEnvironmentTemplates(input: ListEnvironmentTemplatesRequest): ListEnvironmentTemplatesResponse {
val op = SdkHttpOperation.build {
serializeWith = ListEnvironmentTemplatesOperationSerializer()
deserializeWith = ListEnvironmentTemplatesOperationDeserializer()
operationName = "ListEnvironmentTemplates"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* List environments with detail data summaries.
*/
override suspend fun listEnvironments(input: ListEnvironmentsRequest): ListEnvironmentsResponse {
val op = SdkHttpOperation.build {
serializeWith = ListEnvironmentsOperationSerializer()
deserializeWith = ListEnvironmentsOperationDeserializer()
operationName = "ListEnvironments"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* List linked repositories with detail data.
*/
override suspend fun listRepositories(input: ListRepositoriesRequest): ListRepositoriesResponse {
val op = SdkHttpOperation.build {
serializeWith = ListRepositoriesOperationSerializer()
deserializeWith = ListRepositoriesOperationDeserializer()
operationName = "ListRepositories"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* List repository sync definitions with detail data.
*/
override suspend fun listRepositorySyncDefinitions(input: ListRepositorySyncDefinitionsRequest): ListRepositorySyncDefinitionsResponse {
val op = SdkHttpOperation.build {
serializeWith = ListRepositorySyncDefinitionsOperationSerializer()
deserializeWith = ListRepositorySyncDefinitionsOperationDeserializer()
operationName = "ListRepositorySyncDefinitions"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Get a list service of instance Infrastructure as Code (IaC) outputs.
*/
override suspend fun listServiceInstanceOutputs(input: ListServiceInstanceOutputsRequest): ListServiceInstanceOutputsResponse {
val op = SdkHttpOperation.build {
serializeWith = ListServiceInstanceOutputsOperationSerializer()
deserializeWith = ListServiceInstanceOutputsOperationDeserializer()
operationName = "ListServiceInstanceOutputs"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* List provisioned resources for a service instance with details.
*/
override suspend fun listServiceInstanceProvisionedResources(input: ListServiceInstanceProvisionedResourcesRequest): ListServiceInstanceProvisionedResourcesResponse {
val op = SdkHttpOperation.build {
serializeWith = ListServiceInstanceProvisionedResourcesOperationSerializer()
deserializeWith = ListServiceInstanceProvisionedResourcesOperationDeserializer()
operationName = "ListServiceInstanceProvisionedResources"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* List service instances with summary data. This action lists service instances of all services in the Amazon Web Services account.
*/
override suspend fun listServiceInstances(input: ListServiceInstancesRequest): ListServiceInstancesResponse {
val op = SdkHttpOperation.build {
serializeWith = ListServiceInstancesOperationSerializer()
deserializeWith = ListServiceInstancesOperationDeserializer()
operationName = "ListServiceInstances"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Get a list of service pipeline Infrastructure as Code (IaC) outputs.
*/
override suspend fun listServicePipelineOutputs(input: ListServicePipelineOutputsRequest): ListServicePipelineOutputsResponse {
val op = SdkHttpOperation.build {
serializeWith = ListServicePipelineOutputsOperationSerializer()
deserializeWith = ListServicePipelineOutputsOperationDeserializer()
operationName = "ListServicePipelineOutputs"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* List provisioned resources for a service and pipeline with details.
*/
override suspend fun listServicePipelineProvisionedResources(input: ListServicePipelineProvisionedResourcesRequest): ListServicePipelineProvisionedResourcesResponse {
val op = SdkHttpOperation.build {
serializeWith = ListServicePipelineProvisionedResourcesOperationSerializer()
deserializeWith = ListServicePipelineProvisionedResourcesOperationDeserializer()
operationName = "ListServicePipelineProvisionedResources"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* List major or minor versions of a service template with detail data.
*/
override suspend fun listServiceTemplateVersions(input: ListServiceTemplateVersionsRequest): ListServiceTemplateVersionsResponse {
val op = SdkHttpOperation.build {
serializeWith = ListServiceTemplateVersionsOperationSerializer()
deserializeWith = ListServiceTemplateVersionsOperationDeserializer()
operationName = "ListServiceTemplateVersions"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* List service templates with detail data.
*/
override suspend fun listServiceTemplates(input: ListServiceTemplatesRequest): ListServiceTemplatesResponse {
val op = SdkHttpOperation.build {
serializeWith = ListServiceTemplatesOperationSerializer()
deserializeWith = ListServiceTemplatesOperationDeserializer()
operationName = "ListServiceTemplates"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* List services with summaries of detail data.
*/
override suspend fun listServices(input: ListServicesRequest): ListServicesResponse {
val op = SdkHttpOperation.build {
serializeWith = ListServicesOperationSerializer()
deserializeWith = ListServicesOperationDeserializer()
operationName = "ListServices"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* List tags for a resource. For more information, see [Proton resources and tagging](https://docs.aws.amazon.com/proton/latest/userguide/resources.html) in the *Proton User Guide*.
*/
override suspend fun listTagsForResource(input: ListTagsForResourceRequest): ListTagsForResourceResponse {
val op = SdkHttpOperation.build {
serializeWith = ListTagsForResourceOperationSerializer()
deserializeWith = ListTagsForResourceOperationDeserializer()
operationName = "ListTagsForResource"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Notify Proton of status changes to a provisioned resource when you use self-managed provisioning.
*
* For more information, see [Self-managed provisioning](https://docs.aws.amazon.com/proton/latest/userguide/ag-works-prov-methods.html#ag-works-prov-methods-self) in the *Proton User Guide*.
*/
override suspend fun notifyResourceDeploymentStatusChange(input: NotifyResourceDeploymentStatusChangeRequest): NotifyResourceDeploymentStatusChangeResponse {
val op = SdkHttpOperation.build {
serializeWith = NotifyResourceDeploymentStatusChangeOperationSerializer()
deserializeWith = NotifyResourceDeploymentStatusChangeOperationDeserializer()
operationName = "NotifyResourceDeploymentStatusChange"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* In a management account, reject an environment account connection from another environment account.
*
* After you reject an environment account connection request, you *can't* accept or use the rejected environment account connection.
*
* You *can’t* reject an environment account connection that's connected to an environment.
*
* For more information, see [Environment account connections](https://docs.aws.amazon.com/proton/latest/userguide/ag-env-account-connections.html) in the *Proton User guide*.
*/
override suspend fun rejectEnvironmentAccountConnection(input: RejectEnvironmentAccountConnectionRequest): RejectEnvironmentAccountConnectionResponse {
val op = SdkHttpOperation.build {
serializeWith = RejectEnvironmentAccountConnectionOperationSerializer()
deserializeWith = RejectEnvironmentAccountConnectionOperationDeserializer()
operationName = "RejectEnvironmentAccountConnection"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Tag a resource. A tag is a key-value pair of metadata that you associate with an Proton resource.
*
* For more information, see [Proton resources and tagging](https://docs.aws.amazon.com/proton/latest/userguide/resources.html) in the *Proton User Guide*.
*/
override suspend fun tagResource(input: TagResourceRequest): TagResourceResponse {
val op = SdkHttpOperation.build {
serializeWith = TagResourceOperationSerializer()
deserializeWith = TagResourceOperationDeserializer()
operationName = "TagResource"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Remove a customer tag from a resource. A tag is a key-value pair of metadata associated with an Proton resource.
*
* For more information, see [Proton resources and tagging](https://docs.aws.amazon.com/proton/latest/userguide/resources.html) in the *Proton User Guide*.
*/
override suspend fun untagResource(input: UntagResourceRequest): UntagResourceResponse {
val op = SdkHttpOperation.build {
serializeWith = UntagResourceOperationSerializer()
deserializeWith = UntagResourceOperationDeserializer()
operationName = "UntagResource"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Update Proton settings that are used for multiple services in the Amazon Web Services account.
*/
override suspend fun updateAccountSettings(input: UpdateAccountSettingsRequest): UpdateAccountSettingsResponse {
val op = SdkHttpOperation.build {
serializeWith = UpdateAccountSettingsOperationSerializer()
deserializeWith = UpdateAccountSettingsOperationDeserializer()
operationName = "UpdateAccountSettings"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Update a component.
*
* There are a few modes for updating a component. The `deploymentType` field defines the mode.
*
* You can't update a component while its deployment status, or the deployment status of a service instance attached to it, is `IN_PROGRESS`.
*
* For more information about components, see [Proton components](https://docs.aws.amazon.com/proton/latest/userguide/ag-components.html) in the *Proton User Guide*.
*/
override suspend fun updateComponent(input: UpdateComponentRequest): UpdateComponentResponse {
val op = SdkHttpOperation.build {
serializeWith = UpdateComponentOperationSerializer()
deserializeWith = UpdateComponentOperationDeserializer()
operationName = "UpdateComponent"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Update an environment.
*
* If the environment is associated with an environment account connection, *don't* update or include the `protonServiceRoleArn` and `provisioningRepository` parameter to update or connect to an environment account connection.
*
* You can only update to a new environment account connection if that connection was created in the same environment account that the current environment account connection was created in. The account connection must also be associated with the current environment.
*
* If the environment *isn't* associated with an environment account connection, *don't* update or include the `environmentAccountConnectionId` parameter. You *can't* update or connect the environment to an environment account connection if it *isn't* already associated with an environment connection.
*
* You can update either the `environmentAccountConnectionId` or `protonServiceRoleArn` parameter and value. You can’t update both.
*
* If the environment was configured for Amazon Web Services-managed provisioning, omit the `provisioningRepository` parameter.
*
* If the environment was configured for self-managed provisioning, specify the `provisioningRepository` parameter and omit the `protonServiceRoleArn` and `environmentAccountConnectionId` parameters.
*
* For more information, see [Environments](https://docs.aws.amazon.com/proton/latest/userguide/ag-environments.html) and [Provisioning methods](https://docs.aws.amazon.com/proton/latest/userguide/ag-works-prov-methods.html) in the *Proton User Guide*.
*
* There are four modes for updating an environment. The `deploymentType` field defines the mode.
*
* `NONE`
*
* In this mode, a deployment *doesn't* occur. Only the requested metadata parameters are updated.
*
* `CURRENT_VERSION`
*
* In this mode, the environment is deployed and updated with the new spec that you provide. Only requested parameters are updated. *Don’t* include minor or major version parameters when you use this `deployment-type`.
*
* `MINOR_VERSION`
*
* In this mode, the environment is deployed and updated with the published, recommended (latest) minor version of the current major version in use, by default. You can also specify a different minor version of the current major version in use.
*
* `MAJOR_VERSION`
*
* In this mode, the environment is deployed and updated with the published, recommended (latest) major and minor version of the current template, by default. You can also specify a different major version that's higher than the major version in use and a minor version.
*/
override suspend fun updateEnvironment(input: UpdateEnvironmentRequest): UpdateEnvironmentResponse {
val op = SdkHttpOperation.build {
serializeWith = UpdateEnvironmentOperationSerializer()
deserializeWith = UpdateEnvironmentOperationDeserializer()
operationName = "UpdateEnvironment"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* In an environment account, update an environment account connection to use a new IAM role.
*
* For more information, see [Environment account connections](https://docs.aws.amazon.com/proton/latest/userguide/ag-env-account-connections.html) in the *Proton User guide*.
*/
override suspend fun updateEnvironmentAccountConnection(input: UpdateEnvironmentAccountConnectionRequest): UpdateEnvironmentAccountConnectionResponse {
val op = SdkHttpOperation.build {
serializeWith = UpdateEnvironmentAccountConnectionOperationSerializer()
deserializeWith = UpdateEnvironmentAccountConnectionOperationDeserializer()
operationName = "UpdateEnvironmentAccountConnection"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Update an environment template.
*/
override suspend fun updateEnvironmentTemplate(input: UpdateEnvironmentTemplateRequest): UpdateEnvironmentTemplateResponse {
val op = SdkHttpOperation.build {
serializeWith = UpdateEnvironmentTemplateOperationSerializer()
deserializeWith = UpdateEnvironmentTemplateOperationDeserializer()
operationName = "UpdateEnvironmentTemplate"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Update a major or minor version of an environment template.
*/
override suspend fun updateEnvironmentTemplateVersion(input: UpdateEnvironmentTemplateVersionRequest): UpdateEnvironmentTemplateVersionResponse {
val op = SdkHttpOperation.build {
serializeWith = UpdateEnvironmentTemplateVersionOperationSerializer()
deserializeWith = UpdateEnvironmentTemplateVersionOperationDeserializer()
operationName = "UpdateEnvironmentTemplateVersion"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Edit a service description or use a spec to add and delete service instances.
*
* Existing service instances and the service pipeline *can't* be edited using this API. They can only be deleted.
*
* Use the `description` parameter to modify the description.
*
* Edit the `spec` parameter to add or delete instances.
*
* You can't delete a service instance (remove it from the spec) if it has an attached component.
*
* For more information about components, see [Proton components](https://docs.aws.amazon.com/proton/latest/userguide/ag-components.html) in the *Proton User Guide*.
*/
override suspend fun updateService(input: UpdateServiceRequest): UpdateServiceResponse {
val op = SdkHttpOperation.build {
serializeWith = UpdateServiceOperationSerializer()
deserializeWith = UpdateServiceOperationDeserializer()
operationName = "UpdateService"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Update a service instance.
*
* There are a few modes for updating a service instance. The `deploymentType` field defines the mode.
*
* You can't update a service instance while its deployment status, or the deployment status of a component attached to it, is `IN_PROGRESS`.
*
* For more information about components, see [Proton components](https://docs.aws.amazon.com/proton/latest/userguide/ag-components.html) in the *Proton User Guide*.
*/
override suspend fun updateServiceInstance(input: UpdateServiceInstanceRequest): UpdateServiceInstanceResponse {
val op = SdkHttpOperation.build {
serializeWith = UpdateServiceInstanceOperationSerializer()
deserializeWith = UpdateServiceInstanceOperationDeserializer()
operationName = "UpdateServiceInstance"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Update the service pipeline.
*
* There are four modes for updating a service pipeline. The `deploymentType` field defines the mode.
*
* `NONE`
*
* In this mode, a deployment *doesn't* occur. Only the requested metadata parameters are updated.
*
* `CURRENT_VERSION`
*
* In this mode, the service pipeline is deployed and updated with the new spec that you provide. Only requested parameters are updated. *Don’t* include major or minor version parameters when you use this `deployment-type`.
*
* `MINOR_VERSION`
*
* In this mode, the service pipeline is deployed and updated with the published, recommended (latest) minor version of the current major version in use, by default. You can specify a different minor version of the current major version in use.
*
* `MAJOR_VERSION`
*
* In this mode, the service pipeline is deployed and updated with the published, recommended (latest) major and minor version of the current template by default. You can specify a different major version that's higher than the major version in use and a minor version.
*/
override suspend fun updateServicePipeline(input: UpdateServicePipelineRequest): UpdateServicePipelineResponse {
val op = SdkHttpOperation.build {
serializeWith = UpdateServicePipelineOperationSerializer()
deserializeWith = UpdateServicePipelineOperationDeserializer()
operationName = "UpdateServicePipeline"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Update the service sync blocker by resolving it.
*/
override suspend fun updateServiceSyncBlocker(input: UpdateServiceSyncBlockerRequest): UpdateServiceSyncBlockerResponse {
val op = SdkHttpOperation.build {
serializeWith = UpdateServiceSyncBlockerOperationSerializer()
deserializeWith = UpdateServiceSyncBlockerOperationDeserializer()
operationName = "UpdateServiceSyncBlocker"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Update the Proton Ops config file.
*/
override suspend fun updateServiceSyncConfig(input: UpdateServiceSyncConfigRequest): UpdateServiceSyncConfigResponse {
val op = SdkHttpOperation.build {
serializeWith = UpdateServiceSyncConfigOperationSerializer()
deserializeWith = UpdateServiceSyncConfigOperationDeserializer()
operationName = "UpdateServiceSyncConfig"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Update a service template.
*/
override suspend fun updateServiceTemplate(input: UpdateServiceTemplateRequest): UpdateServiceTemplateResponse {
val op = SdkHttpOperation.build {
serializeWith = UpdateServiceTemplateOperationSerializer()
deserializeWith = UpdateServiceTemplateOperationDeserializer()
operationName = "UpdateServiceTemplate"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Update a major or minor version of a service template.
*/
override suspend fun updateServiceTemplateVersion(input: UpdateServiceTemplateVersionRequest): UpdateServiceTemplateVersionResponse {
val op = SdkHttpOperation.build {
serializeWith = UpdateServiceTemplateVersionOperationSerializer()
deserializeWith = UpdateServiceTemplateVersionOperationDeserializer()
operationName = "UpdateServiceTemplateVersion"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Update template sync configuration parameters, except for the `templateName` and `templateType`. Repository details (branch, name, and provider) should be of a linked repository. A linked repository is a repository that has been registered with Proton. For more information, see CreateRepository.
*/
override suspend fun updateTemplateSyncConfig(input: UpdateTemplateSyncConfigRequest): UpdateTemplateSyncConfigResponse {
val op = SdkHttpOperation.build {
serializeWith = UpdateTemplateSyncConfigOperationSerializer()
deserializeWith = UpdateTemplateSyncConfigOperationDeserializer()
operationName = "UpdateTemplateSyncConfig"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("AwsProton20200720", "1.0"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
override fun close() {
managedResources.unshareAll()
}
/**
* merge the defaults configured for the service into the execution context before firing off a request
*/
private fun mergeServiceDefaults(ctx: ExecutionContext) {
ctx.putIfAbsent(SdkClientOption.ClientName, config.clientName)
ctx.putIfAbsent(SdkClientOption.LogMode, config.logMode)
ctx.putIfAbsentNotNull(SdkClientOption.IdempotencyTokenProvider, config.idempotencyTokenProvider)
ctx.putIfAbsentNotNull(AwsAttributes.Region, config.region)
ctx.putIfAbsentNotNull(AwsSigningAttributes.SigningRegion, config.region)
ctx.putIfAbsent(AwsSigningAttributes.SigningService, "proton")
ctx.putIfAbsent(AwsSigningAttributes.CredentialsProvider, config.credentialsProvider)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy