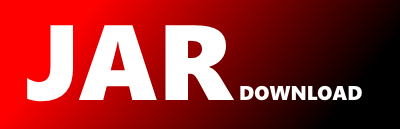
commonMain.aws.sdk.kotlin.services.proton.model.CreateEnvironmentAccountConnectionRequest.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of proton-jvm Show documentation
Show all versions of proton-jvm Show documentation
The AWS SDK for Kotlin client for Proton
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.proton.model
import aws.smithy.kotlin.runtime.SdkDsl
public class CreateEnvironmentAccountConnectionRequest private constructor(builder: Builder) {
/**
* When included, if two identical requests are made with the same client token, Proton returns the environment account connection that the first request created.
*/
public val clientToken: kotlin.String? = builder.clientToken
/**
* The Amazon Resource Name (ARN) of an IAM service role in the environment account. Proton uses this role to provision infrastructure resources using CodeBuild-based provisioning in the associated environment account.
*/
public val codebuildRoleArn: kotlin.String? = builder.codebuildRoleArn
/**
* The Amazon Resource Name (ARN) of the IAM service role that Proton uses when provisioning directly defined components in the associated environment account. It determines the scope of infrastructure that a component can provision in the account.
*
* You must specify `componentRoleArn` to allow directly defined components to be associated with any environments running in this account.
*
* For more information about components, see [Proton components](https://docs.aws.amazon.com/proton/latest/userguide/ag-components.html) in the *Proton User Guide*.
*/
public val componentRoleArn: kotlin.String? = builder.componentRoleArn
/**
* The name of the Proton environment that's created in the associated management account.
*/
public val environmentName: kotlin.String = requireNotNull(builder.environmentName) { "A non-null value must be provided for environmentName" }
/**
* The ID of the management account that accepts or rejects the environment account connection. You create and manage the Proton environment in this account. If the management account accepts the environment account connection, Proton can use the associated IAM role to provision environment infrastructure resources in the associated environment account.
*/
public val managementAccountId: kotlin.String = requireNotNull(builder.managementAccountId) { "A non-null value must be provided for managementAccountId" }
/**
* The Amazon Resource Name (ARN) of the IAM service role that's created in the environment account. Proton uses this role to provision infrastructure resources in the associated environment account.
*/
public val roleArn: kotlin.String? = builder.roleArn
/**
* An optional list of metadata items that you can associate with the Proton environment account connection. A tag is a key-value pair.
*
* For more information, see [Proton resources and tagging](https://docs.aws.amazon.com/proton/latest/userguide/resources.html) in the *Proton User Guide*.
*/
public val tags: List? = builder.tags
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.proton.model.CreateEnvironmentAccountConnectionRequest = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("CreateEnvironmentAccountConnectionRequest(")
append("clientToken=$clientToken,")
append("codebuildRoleArn=$codebuildRoleArn,")
append("componentRoleArn=$componentRoleArn,")
append("environmentName=$environmentName,")
append("managementAccountId=$managementAccountId,")
append("roleArn=$roleArn,")
append("tags=$tags")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = clientToken?.hashCode() ?: 0
result = 31 * result + (codebuildRoleArn?.hashCode() ?: 0)
result = 31 * result + (componentRoleArn?.hashCode() ?: 0)
result = 31 * result + (environmentName.hashCode())
result = 31 * result + (managementAccountId.hashCode())
result = 31 * result + (roleArn?.hashCode() ?: 0)
result = 31 * result + (tags?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as CreateEnvironmentAccountConnectionRequest
if (clientToken != other.clientToken) return false
if (codebuildRoleArn != other.codebuildRoleArn) return false
if (componentRoleArn != other.componentRoleArn) return false
if (environmentName != other.environmentName) return false
if (managementAccountId != other.managementAccountId) return false
if (roleArn != other.roleArn) return false
if (tags != other.tags) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.proton.model.CreateEnvironmentAccountConnectionRequest = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* When included, if two identical requests are made with the same client token, Proton returns the environment account connection that the first request created.
*/
public var clientToken: kotlin.String? = null
/**
* The Amazon Resource Name (ARN) of an IAM service role in the environment account. Proton uses this role to provision infrastructure resources using CodeBuild-based provisioning in the associated environment account.
*/
public var codebuildRoleArn: kotlin.String? = null
/**
* The Amazon Resource Name (ARN) of the IAM service role that Proton uses when provisioning directly defined components in the associated environment account. It determines the scope of infrastructure that a component can provision in the account.
*
* You must specify `componentRoleArn` to allow directly defined components to be associated with any environments running in this account.
*
* For more information about components, see [Proton components](https://docs.aws.amazon.com/proton/latest/userguide/ag-components.html) in the *Proton User Guide*.
*/
public var componentRoleArn: kotlin.String? = null
/**
* The name of the Proton environment that's created in the associated management account.
*/
public var environmentName: kotlin.String? = null
/**
* The ID of the management account that accepts or rejects the environment account connection. You create and manage the Proton environment in this account. If the management account accepts the environment account connection, Proton can use the associated IAM role to provision environment infrastructure resources in the associated environment account.
*/
public var managementAccountId: kotlin.String? = null
/**
* The Amazon Resource Name (ARN) of the IAM service role that's created in the environment account. Proton uses this role to provision infrastructure resources in the associated environment account.
*/
public var roleArn: kotlin.String? = null
/**
* An optional list of metadata items that you can associate with the Proton environment account connection. A tag is a key-value pair.
*
* For more information, see [Proton resources and tagging](https://docs.aws.amazon.com/proton/latest/userguide/resources.html) in the *Proton User Guide*.
*/
public var tags: List? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.proton.model.CreateEnvironmentAccountConnectionRequest) : this() {
this.clientToken = x.clientToken
this.codebuildRoleArn = x.codebuildRoleArn
this.componentRoleArn = x.componentRoleArn
this.environmentName = x.environmentName
this.managementAccountId = x.managementAccountId
this.roleArn = x.roleArn
this.tags = x.tags
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.proton.model.CreateEnvironmentAccountConnectionRequest = CreateEnvironmentAccountConnectionRequest(this)
internal fun correctErrors(): Builder {
if (environmentName == null) environmentName = ""
if (managementAccountId == null) managementAccountId = ""
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy