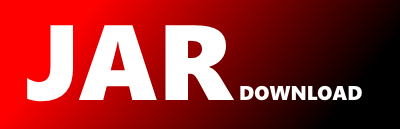
commonMain.aws.sdk.kotlin.services.proton.model.EnvironmentAccountConnection.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of proton-jvm Show documentation
Show all versions of proton-jvm Show documentation
The AWS SDK for Kotlin client for Proton
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.proton.model
import aws.smithy.kotlin.runtime.SdkDsl
import aws.smithy.kotlin.runtime.time.Instant
/**
* Detailed data of an Proton environment account connection resource.
*/
public class EnvironmentAccountConnection private constructor(builder: Builder) {
/**
* The Amazon Resource Name (ARN) of the environment account connection.
*/
public val arn: kotlin.String = requireNotNull(builder.arn) { "A non-null value must be provided for arn" }
/**
* The Amazon Resource Name (ARN) of an IAM service role in the environment account. Proton uses this role to provision infrastructure resources using CodeBuild-based provisioning in the associated environment account.
*/
public val codebuildRoleArn: kotlin.String? = builder.codebuildRoleArn
/**
* The Amazon Resource Name (ARN) of the IAM service role that Proton uses when provisioning directly defined components in the associated environment account. It determines the scope of infrastructure that a component can provision in the account.
*
* The environment account connection must have a `componentRoleArn` to allow directly defined components to be associated with any environments running in the account.
*
* For more information about components, see [Proton components](https://docs.aws.amazon.com/proton/latest/userguide/ag-components.html) in the *Proton User Guide*.
*/
public val componentRoleArn: kotlin.String? = builder.componentRoleArn
/**
* The environment account that's connected to the environment account connection.
*/
public val environmentAccountId: kotlin.String = requireNotNull(builder.environmentAccountId) { "A non-null value must be provided for environmentAccountId" }
/**
* The name of the environment that's associated with the environment account connection.
*/
public val environmentName: kotlin.String = requireNotNull(builder.environmentName) { "A non-null value must be provided for environmentName" }
/**
* The ID of the environment account connection.
*/
public val id: kotlin.String = requireNotNull(builder.id) { "A non-null value must be provided for id" }
/**
* The time when the environment account connection was last modified.
*/
public val lastModifiedAt: aws.smithy.kotlin.runtime.time.Instant = requireNotNull(builder.lastModifiedAt) { "A non-null value must be provided for lastModifiedAt" }
/**
* The ID of the management account that's connected to the environment account connection.
*/
public val managementAccountId: kotlin.String = requireNotNull(builder.managementAccountId) { "A non-null value must be provided for managementAccountId" }
/**
* The time when the environment account connection request was made.
*/
public val requestedAt: aws.smithy.kotlin.runtime.time.Instant = requireNotNull(builder.requestedAt) { "A non-null value must be provided for requestedAt" }
/**
* The IAM service role that's associated with the environment account connection.
*/
public val roleArn: kotlin.String = requireNotNull(builder.roleArn) { "A non-null value must be provided for roleArn" }
/**
* The status of the environment account connection.
*/
public val status: aws.sdk.kotlin.services.proton.model.EnvironmentAccountConnectionStatus = requireNotNull(builder.status) { "A non-null value must be provided for status" }
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.proton.model.EnvironmentAccountConnection = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("EnvironmentAccountConnection(")
append("arn=$arn,")
append("codebuildRoleArn=$codebuildRoleArn,")
append("componentRoleArn=$componentRoleArn,")
append("environmentAccountId=$environmentAccountId,")
append("environmentName=$environmentName,")
append("id=$id,")
append("lastModifiedAt=$lastModifiedAt,")
append("managementAccountId=$managementAccountId,")
append("requestedAt=$requestedAt,")
append("roleArn=$roleArn,")
append("status=$status")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = arn.hashCode()
result = 31 * result + (codebuildRoleArn?.hashCode() ?: 0)
result = 31 * result + (componentRoleArn?.hashCode() ?: 0)
result = 31 * result + (environmentAccountId.hashCode())
result = 31 * result + (environmentName.hashCode())
result = 31 * result + (id.hashCode())
result = 31 * result + (lastModifiedAt.hashCode())
result = 31 * result + (managementAccountId.hashCode())
result = 31 * result + (requestedAt.hashCode())
result = 31 * result + (roleArn.hashCode())
result = 31 * result + (status.hashCode())
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as EnvironmentAccountConnection
if (arn != other.arn) return false
if (codebuildRoleArn != other.codebuildRoleArn) return false
if (componentRoleArn != other.componentRoleArn) return false
if (environmentAccountId != other.environmentAccountId) return false
if (environmentName != other.environmentName) return false
if (id != other.id) return false
if (lastModifiedAt != other.lastModifiedAt) return false
if (managementAccountId != other.managementAccountId) return false
if (requestedAt != other.requestedAt) return false
if (roleArn != other.roleArn) return false
if (status != other.status) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.proton.model.EnvironmentAccountConnection = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The Amazon Resource Name (ARN) of the environment account connection.
*/
public var arn: kotlin.String? = null
/**
* The Amazon Resource Name (ARN) of an IAM service role in the environment account. Proton uses this role to provision infrastructure resources using CodeBuild-based provisioning in the associated environment account.
*/
public var codebuildRoleArn: kotlin.String? = null
/**
* The Amazon Resource Name (ARN) of the IAM service role that Proton uses when provisioning directly defined components in the associated environment account. It determines the scope of infrastructure that a component can provision in the account.
*
* The environment account connection must have a `componentRoleArn` to allow directly defined components to be associated with any environments running in the account.
*
* For more information about components, see [Proton components](https://docs.aws.amazon.com/proton/latest/userguide/ag-components.html) in the *Proton User Guide*.
*/
public var componentRoleArn: kotlin.String? = null
/**
* The environment account that's connected to the environment account connection.
*/
public var environmentAccountId: kotlin.String? = null
/**
* The name of the environment that's associated with the environment account connection.
*/
public var environmentName: kotlin.String? = null
/**
* The ID of the environment account connection.
*/
public var id: kotlin.String? = null
/**
* The time when the environment account connection was last modified.
*/
public var lastModifiedAt: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The ID of the management account that's connected to the environment account connection.
*/
public var managementAccountId: kotlin.String? = null
/**
* The time when the environment account connection request was made.
*/
public var requestedAt: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The IAM service role that's associated with the environment account connection.
*/
public var roleArn: kotlin.String? = null
/**
* The status of the environment account connection.
*/
public var status: aws.sdk.kotlin.services.proton.model.EnvironmentAccountConnectionStatus? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.proton.model.EnvironmentAccountConnection) : this() {
this.arn = x.arn
this.codebuildRoleArn = x.codebuildRoleArn
this.componentRoleArn = x.componentRoleArn
this.environmentAccountId = x.environmentAccountId
this.environmentName = x.environmentName
this.id = x.id
this.lastModifiedAt = x.lastModifiedAt
this.managementAccountId = x.managementAccountId
this.requestedAt = x.requestedAt
this.roleArn = x.roleArn
this.status = x.status
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.proton.model.EnvironmentAccountConnection = EnvironmentAccountConnection(this)
internal fun correctErrors(): Builder {
if (arn == null) arn = ""
if (environmentAccountId == null) environmentAccountId = ""
if (environmentName == null) environmentName = ""
if (id == null) id = ""
if (lastModifiedAt == null) lastModifiedAt = Instant.fromEpochSeconds(0)
if (managementAccountId == null) managementAccountId = ""
if (requestedAt == null) requestedAt = Instant.fromEpochSeconds(0)
if (roleArn == null) roleArn = ""
if (status == null) status = EnvironmentAccountConnectionStatus.SdkUnknown("no value provided")
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy