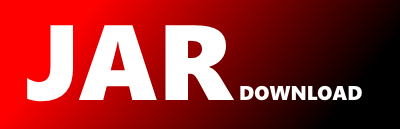
commonMain.aws.sdk.kotlin.services.proton.model.UpdateComponentRequest.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of proton-jvm Show documentation
Show all versions of proton-jvm Show documentation
The AWS SDK for Kotlin client for Proton
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.proton.model
import aws.smithy.kotlin.runtime.SdkDsl
public class UpdateComponentRequest private constructor(builder: Builder) {
/**
* The client token for the updated component.
*/
public val clientToken: kotlin.String? = builder.clientToken
/**
* The deployment type. It defines the mode for updating a component, as follows:
*
* `NONE`
*
* In this mode, a deployment *doesn't* occur. Only the requested metadata parameters are updated. You can only specify `description` in this mode.
*
* `CURRENT_VERSION`
*
* In this mode, the component is deployed and updated with the new `serviceSpec`, `templateSource`, and/or `type` that you provide. Only requested parameters are updated.
*/
public val deploymentType: aws.sdk.kotlin.services.proton.model.ComponentDeploymentUpdateType = requireNotNull(builder.deploymentType) { "A non-null value must be provided for deploymentType" }
/**
* An optional customer-provided description of the component.
*/
public val description: kotlin.String? = builder.description
/**
* The name of the component to update.
*/
public val name: kotlin.String = requireNotNull(builder.name) { "A non-null value must be provided for name" }
/**
* The name of the service instance that you want to attach this component to. Don't specify to keep the component's current service instance attachment. Specify an empty string to detach the component from the service instance it's attached to. Specify non-empty values for both `serviceInstanceName` and `serviceName` or for neither of them.
*/
public val serviceInstanceName: kotlin.String? = builder.serviceInstanceName
/**
* The name of the service that `serviceInstanceName` is associated with. Don't specify to keep the component's current service instance attachment. Specify an empty string to detach the component from the service instance it's attached to. Specify non-empty values for both `serviceInstanceName` and `serviceName` or for neither of them.
*/
public val serviceName: kotlin.String? = builder.serviceName
/**
* The service spec that you want the component to use to access service inputs. Set this only when the component is attached to a service instance.
*/
public val serviceSpec: kotlin.String? = builder.serviceSpec
/**
* A path to the Infrastructure as Code (IaC) file describing infrastructure that a custom component provisions.
*
* Components support a single IaC file, even if you use Terraform as your template language.
*/
public val templateFile: kotlin.String? = builder.templateFile
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.proton.model.UpdateComponentRequest = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("UpdateComponentRequest(")
append("clientToken=$clientToken,")
append("deploymentType=$deploymentType,")
append("description=*** Sensitive Data Redacted ***,")
append("name=$name,")
append("serviceInstanceName=$serviceInstanceName,")
append("serviceName=$serviceName,")
append("serviceSpec=*** Sensitive Data Redacted ***,")
append("templateFile=*** Sensitive Data Redacted ***")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = clientToken?.hashCode() ?: 0
result = 31 * result + (deploymentType.hashCode())
result = 31 * result + (description?.hashCode() ?: 0)
result = 31 * result + (name.hashCode())
result = 31 * result + (serviceInstanceName?.hashCode() ?: 0)
result = 31 * result + (serviceName?.hashCode() ?: 0)
result = 31 * result + (serviceSpec?.hashCode() ?: 0)
result = 31 * result + (templateFile?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as UpdateComponentRequest
if (clientToken != other.clientToken) return false
if (deploymentType != other.deploymentType) return false
if (description != other.description) return false
if (name != other.name) return false
if (serviceInstanceName != other.serviceInstanceName) return false
if (serviceName != other.serviceName) return false
if (serviceSpec != other.serviceSpec) return false
if (templateFile != other.templateFile) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.proton.model.UpdateComponentRequest = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The client token for the updated component.
*/
public var clientToken: kotlin.String? = null
/**
* The deployment type. It defines the mode for updating a component, as follows:
*
* `NONE`
*
* In this mode, a deployment *doesn't* occur. Only the requested metadata parameters are updated. You can only specify `description` in this mode.
*
* `CURRENT_VERSION`
*
* In this mode, the component is deployed and updated with the new `serviceSpec`, `templateSource`, and/or `type` that you provide. Only requested parameters are updated.
*/
public var deploymentType: aws.sdk.kotlin.services.proton.model.ComponentDeploymentUpdateType? = null
/**
* An optional customer-provided description of the component.
*/
public var description: kotlin.String? = null
/**
* The name of the component to update.
*/
public var name: kotlin.String? = null
/**
* The name of the service instance that you want to attach this component to. Don't specify to keep the component's current service instance attachment. Specify an empty string to detach the component from the service instance it's attached to. Specify non-empty values for both `serviceInstanceName` and `serviceName` or for neither of them.
*/
public var serviceInstanceName: kotlin.String? = null
/**
* The name of the service that `serviceInstanceName` is associated with. Don't specify to keep the component's current service instance attachment. Specify an empty string to detach the component from the service instance it's attached to. Specify non-empty values for both `serviceInstanceName` and `serviceName` or for neither of them.
*/
public var serviceName: kotlin.String? = null
/**
* The service spec that you want the component to use to access service inputs. Set this only when the component is attached to a service instance.
*/
public var serviceSpec: kotlin.String? = null
/**
* A path to the Infrastructure as Code (IaC) file describing infrastructure that a custom component provisions.
*
* Components support a single IaC file, even if you use Terraform as your template language.
*/
public var templateFile: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.proton.model.UpdateComponentRequest) : this() {
this.clientToken = x.clientToken
this.deploymentType = x.deploymentType
this.description = x.description
this.name = x.name
this.serviceInstanceName = x.serviceInstanceName
this.serviceName = x.serviceName
this.serviceSpec = x.serviceSpec
this.templateFile = x.templateFile
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.proton.model.UpdateComponentRequest = UpdateComponentRequest(this)
internal fun correctErrors(): Builder {
if (deploymentType == null) deploymentType = ComponentDeploymentUpdateType.SdkUnknown("no value provided")
if (name == null) name = ""
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy