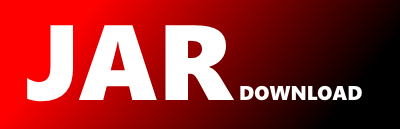
commonMain.aws.sdk.kotlin.services.proton.model.CountsSummary.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of proton-jvm Show documentation
Show all versions of proton-jvm Show documentation
The AWS SDK for Kotlin client for Proton
The newest version!
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.proton.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* Summary counts of each Proton resource type.
*/
public class CountsSummary private constructor(builder: Builder) {
/**
* The total number of components in the Amazon Web Services account.
*
* The semantics of the `components` field are different from the semantics of results for other infrastructure-provisioning resources. That's because at this time components don't have associated templates, therefore they don't have the concept of staleness. The `components` object will only contain `total` and `failed` members.
*/
public val components: aws.sdk.kotlin.services.proton.model.ResourceCountsSummary? = builder.components
/**
* The total number of environment templates in the Amazon Web Services account. The `environmentTemplates` object will only contain `total` members.
*/
public val environmentTemplates: aws.sdk.kotlin.services.proton.model.ResourceCountsSummary? = builder.environmentTemplates
/**
* The staleness counts for Proton environments in the Amazon Web Services account. The `environments` object will only contain `total` members.
*/
public val environments: aws.sdk.kotlin.services.proton.model.ResourceCountsSummary? = builder.environments
/**
* The staleness counts for Proton pipelines in the Amazon Web Services account.
*/
public val pipelines: aws.sdk.kotlin.services.proton.model.ResourceCountsSummary? = builder.pipelines
/**
* The staleness counts for Proton service instances in the Amazon Web Services account.
*/
public val serviceInstances: aws.sdk.kotlin.services.proton.model.ResourceCountsSummary? = builder.serviceInstances
/**
* The total number of service templates in the Amazon Web Services account. The `serviceTemplates` object will only contain `total` members.
*/
public val serviceTemplates: aws.sdk.kotlin.services.proton.model.ResourceCountsSummary? = builder.serviceTemplates
/**
* The staleness counts for Proton services in the Amazon Web Services account.
*/
public val services: aws.sdk.kotlin.services.proton.model.ResourceCountsSummary? = builder.services
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.proton.model.CountsSummary = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("CountsSummary(")
append("components=$components,")
append("environmentTemplates=$environmentTemplates,")
append("environments=$environments,")
append("pipelines=$pipelines,")
append("serviceInstances=$serviceInstances,")
append("serviceTemplates=$serviceTemplates,")
append("services=$services")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = components?.hashCode() ?: 0
result = 31 * result + (environmentTemplates?.hashCode() ?: 0)
result = 31 * result + (environments?.hashCode() ?: 0)
result = 31 * result + (pipelines?.hashCode() ?: 0)
result = 31 * result + (serviceInstances?.hashCode() ?: 0)
result = 31 * result + (serviceTemplates?.hashCode() ?: 0)
result = 31 * result + (services?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as CountsSummary
if (components != other.components) return false
if (environmentTemplates != other.environmentTemplates) return false
if (environments != other.environments) return false
if (pipelines != other.pipelines) return false
if (serviceInstances != other.serviceInstances) return false
if (serviceTemplates != other.serviceTemplates) return false
if (services != other.services) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.proton.model.CountsSummary = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The total number of components in the Amazon Web Services account.
*
* The semantics of the `components` field are different from the semantics of results for other infrastructure-provisioning resources. That's because at this time components don't have associated templates, therefore they don't have the concept of staleness. The `components` object will only contain `total` and `failed` members.
*/
public var components: aws.sdk.kotlin.services.proton.model.ResourceCountsSummary? = null
/**
* The total number of environment templates in the Amazon Web Services account. The `environmentTemplates` object will only contain `total` members.
*/
public var environmentTemplates: aws.sdk.kotlin.services.proton.model.ResourceCountsSummary? = null
/**
* The staleness counts for Proton environments in the Amazon Web Services account. The `environments` object will only contain `total` members.
*/
public var environments: aws.sdk.kotlin.services.proton.model.ResourceCountsSummary? = null
/**
* The staleness counts for Proton pipelines in the Amazon Web Services account.
*/
public var pipelines: aws.sdk.kotlin.services.proton.model.ResourceCountsSummary? = null
/**
* The staleness counts for Proton service instances in the Amazon Web Services account.
*/
public var serviceInstances: aws.sdk.kotlin.services.proton.model.ResourceCountsSummary? = null
/**
* The total number of service templates in the Amazon Web Services account. The `serviceTemplates` object will only contain `total` members.
*/
public var serviceTemplates: aws.sdk.kotlin.services.proton.model.ResourceCountsSummary? = null
/**
* The staleness counts for Proton services in the Amazon Web Services account.
*/
public var services: aws.sdk.kotlin.services.proton.model.ResourceCountsSummary? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.proton.model.CountsSummary) : this() {
this.components = x.components
this.environmentTemplates = x.environmentTemplates
this.environments = x.environments
this.pipelines = x.pipelines
this.serviceInstances = x.serviceInstances
this.serviceTemplates = x.serviceTemplates
this.services = x.services
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.proton.model.CountsSummary = CountsSummary(this)
/**
* construct an [aws.sdk.kotlin.services.proton.model.ResourceCountsSummary] inside the given [block]
*/
public fun components(block: aws.sdk.kotlin.services.proton.model.ResourceCountsSummary.Builder.() -> kotlin.Unit) {
this.components = aws.sdk.kotlin.services.proton.model.ResourceCountsSummary.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.proton.model.ResourceCountsSummary] inside the given [block]
*/
public fun environmentTemplates(block: aws.sdk.kotlin.services.proton.model.ResourceCountsSummary.Builder.() -> kotlin.Unit) {
this.environmentTemplates = aws.sdk.kotlin.services.proton.model.ResourceCountsSummary.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.proton.model.ResourceCountsSummary] inside the given [block]
*/
public fun environments(block: aws.sdk.kotlin.services.proton.model.ResourceCountsSummary.Builder.() -> kotlin.Unit) {
this.environments = aws.sdk.kotlin.services.proton.model.ResourceCountsSummary.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.proton.model.ResourceCountsSummary] inside the given [block]
*/
public fun pipelines(block: aws.sdk.kotlin.services.proton.model.ResourceCountsSummary.Builder.() -> kotlin.Unit) {
this.pipelines = aws.sdk.kotlin.services.proton.model.ResourceCountsSummary.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.proton.model.ResourceCountsSummary] inside the given [block]
*/
public fun serviceInstances(block: aws.sdk.kotlin.services.proton.model.ResourceCountsSummary.Builder.() -> kotlin.Unit) {
this.serviceInstances = aws.sdk.kotlin.services.proton.model.ResourceCountsSummary.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.proton.model.ResourceCountsSummary] inside the given [block]
*/
public fun serviceTemplates(block: aws.sdk.kotlin.services.proton.model.ResourceCountsSummary.Builder.() -> kotlin.Unit) {
this.serviceTemplates = aws.sdk.kotlin.services.proton.model.ResourceCountsSummary.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.proton.model.ResourceCountsSummary] inside the given [block]
*/
public fun services(block: aws.sdk.kotlin.services.proton.model.ResourceCountsSummary.Builder.() -> kotlin.Unit) {
this.services = aws.sdk.kotlin.services.proton.model.ResourceCountsSummary.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy