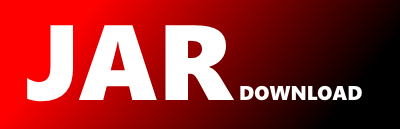
commonMain.aws.sdk.kotlin.services.proton.model.Deployment.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of proton-jvm Show documentation
Show all versions of proton-jvm Show documentation
The AWS SDK for Kotlin client for Proton
The newest version!
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.proton.model
import aws.smithy.kotlin.runtime.SdkDsl
import aws.smithy.kotlin.runtime.time.Instant
/**
* The detailed information about a deployment.
*/
public class Deployment private constructor(builder: Builder) {
/**
* The Amazon Resource Name (ARN) of the deployment.
*/
public val arn: kotlin.String = requireNotNull(builder.arn) { "A non-null value must be provided for arn" }
/**
* The date and time the deployment was completed.
*/
public val completedAt: aws.smithy.kotlin.runtime.time.Instant? = builder.completedAt
/**
* The name of the component associated with this deployment.
*/
public val componentName: kotlin.String? = builder.componentName
/**
* The date and time the deployment was created.
*/
public val createdAt: aws.smithy.kotlin.runtime.time.Instant = requireNotNull(builder.createdAt) { "A non-null value must be provided for createdAt" }
/**
* The status of the deployment.
*/
public val deploymentStatus: aws.sdk.kotlin.services.proton.model.DeploymentStatus = requireNotNull(builder.deploymentStatus) { "A non-null value must be provided for deploymentStatus" }
/**
* The deployment status message.
*/
public val deploymentStatusMessage: kotlin.String? = builder.deploymentStatusMessage
/**
* The name of the environment associated with this deployment.
*/
public val environmentName: kotlin.String = requireNotNull(builder.environmentName) { "A non-null value must be provided for environmentName" }
/**
* The ID of the deployment.
*/
public val id: kotlin.String = requireNotNull(builder.id) { "A non-null value must be provided for id" }
/**
* The initial state of the target resource at the time of the deployment.
*/
public val initialState: aws.sdk.kotlin.services.proton.model.DeploymentState? = builder.initialState
/**
* The ID of the last attempted deployment.
*/
public val lastAttemptedDeploymentId: kotlin.String? = builder.lastAttemptedDeploymentId
/**
* The date and time the deployment was last modified.
*/
public val lastModifiedAt: aws.smithy.kotlin.runtime.time.Instant = requireNotNull(builder.lastModifiedAt) { "A non-null value must be provided for lastModifiedAt" }
/**
* The ID of the last successful deployment.
*/
public val lastSucceededDeploymentId: kotlin.String? = builder.lastSucceededDeploymentId
/**
* The name of the deployment's service instance.
*/
public val serviceInstanceName: kotlin.String? = builder.serviceInstanceName
/**
* The name of the service in this deployment.
*/
public val serviceName: kotlin.String? = builder.serviceName
/**
* The Amazon Resource Name (ARN) of the target of the deployment.
*/
public val targetArn: kotlin.String = requireNotNull(builder.targetArn) { "A non-null value must be provided for targetArn" }
/**
* The date and time the depoyment target was created.
*/
public val targetResourceCreatedAt: aws.smithy.kotlin.runtime.time.Instant = requireNotNull(builder.targetResourceCreatedAt) { "A non-null value must be provided for targetResourceCreatedAt" }
/**
* The resource type of the deployment target. It can be an environment, service, service instance, or component.
*/
public val targetResourceType: aws.sdk.kotlin.services.proton.model.DeploymentTargetResourceType = requireNotNull(builder.targetResourceType) { "A non-null value must be provided for targetResourceType" }
/**
* The target state of the target resource at the time of the deployment.
*/
public val targetState: aws.sdk.kotlin.services.proton.model.DeploymentState? = builder.targetState
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.proton.model.Deployment = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("Deployment(")
append("arn=$arn,")
append("completedAt=$completedAt,")
append("componentName=$componentName,")
append("createdAt=$createdAt,")
append("deploymentStatus=$deploymentStatus,")
append("deploymentStatusMessage=*** Sensitive Data Redacted ***,")
append("environmentName=$environmentName,")
append("id=$id,")
append("initialState=$initialState,")
append("lastAttemptedDeploymentId=$lastAttemptedDeploymentId,")
append("lastModifiedAt=$lastModifiedAt,")
append("lastSucceededDeploymentId=$lastSucceededDeploymentId,")
append("serviceInstanceName=$serviceInstanceName,")
append("serviceName=$serviceName,")
append("targetArn=$targetArn,")
append("targetResourceCreatedAt=$targetResourceCreatedAt,")
append("targetResourceType=$targetResourceType,")
append("targetState=$targetState")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = arn.hashCode()
result = 31 * result + (completedAt?.hashCode() ?: 0)
result = 31 * result + (componentName?.hashCode() ?: 0)
result = 31 * result + (createdAt.hashCode())
result = 31 * result + (deploymentStatus.hashCode())
result = 31 * result + (deploymentStatusMessage?.hashCode() ?: 0)
result = 31 * result + (environmentName.hashCode())
result = 31 * result + (id.hashCode())
result = 31 * result + (initialState?.hashCode() ?: 0)
result = 31 * result + (lastAttemptedDeploymentId?.hashCode() ?: 0)
result = 31 * result + (lastModifiedAt.hashCode())
result = 31 * result + (lastSucceededDeploymentId?.hashCode() ?: 0)
result = 31 * result + (serviceInstanceName?.hashCode() ?: 0)
result = 31 * result + (serviceName?.hashCode() ?: 0)
result = 31 * result + (targetArn.hashCode())
result = 31 * result + (targetResourceCreatedAt.hashCode())
result = 31 * result + (targetResourceType.hashCode())
result = 31 * result + (targetState?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as Deployment
if (arn != other.arn) return false
if (completedAt != other.completedAt) return false
if (componentName != other.componentName) return false
if (createdAt != other.createdAt) return false
if (deploymentStatus != other.deploymentStatus) return false
if (deploymentStatusMessage != other.deploymentStatusMessage) return false
if (environmentName != other.environmentName) return false
if (id != other.id) return false
if (initialState != other.initialState) return false
if (lastAttemptedDeploymentId != other.lastAttemptedDeploymentId) return false
if (lastModifiedAt != other.lastModifiedAt) return false
if (lastSucceededDeploymentId != other.lastSucceededDeploymentId) return false
if (serviceInstanceName != other.serviceInstanceName) return false
if (serviceName != other.serviceName) return false
if (targetArn != other.targetArn) return false
if (targetResourceCreatedAt != other.targetResourceCreatedAt) return false
if (targetResourceType != other.targetResourceType) return false
if (targetState != other.targetState) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.proton.model.Deployment = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The Amazon Resource Name (ARN) of the deployment.
*/
public var arn: kotlin.String? = null
/**
* The date and time the deployment was completed.
*/
public var completedAt: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The name of the component associated with this deployment.
*/
public var componentName: kotlin.String? = null
/**
* The date and time the deployment was created.
*/
public var createdAt: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The status of the deployment.
*/
public var deploymentStatus: aws.sdk.kotlin.services.proton.model.DeploymentStatus? = null
/**
* The deployment status message.
*/
public var deploymentStatusMessage: kotlin.String? = null
/**
* The name of the environment associated with this deployment.
*/
public var environmentName: kotlin.String? = null
/**
* The ID of the deployment.
*/
public var id: kotlin.String? = null
/**
* The initial state of the target resource at the time of the deployment.
*/
public var initialState: aws.sdk.kotlin.services.proton.model.DeploymentState? = null
/**
* The ID of the last attempted deployment.
*/
public var lastAttemptedDeploymentId: kotlin.String? = null
/**
* The date and time the deployment was last modified.
*/
public var lastModifiedAt: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The ID of the last successful deployment.
*/
public var lastSucceededDeploymentId: kotlin.String? = null
/**
* The name of the deployment's service instance.
*/
public var serviceInstanceName: kotlin.String? = null
/**
* The name of the service in this deployment.
*/
public var serviceName: kotlin.String? = null
/**
* The Amazon Resource Name (ARN) of the target of the deployment.
*/
public var targetArn: kotlin.String? = null
/**
* The date and time the depoyment target was created.
*/
public var targetResourceCreatedAt: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The resource type of the deployment target. It can be an environment, service, service instance, or component.
*/
public var targetResourceType: aws.sdk.kotlin.services.proton.model.DeploymentTargetResourceType? = null
/**
* The target state of the target resource at the time of the deployment.
*/
public var targetState: aws.sdk.kotlin.services.proton.model.DeploymentState? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.proton.model.Deployment) : this() {
this.arn = x.arn
this.completedAt = x.completedAt
this.componentName = x.componentName
this.createdAt = x.createdAt
this.deploymentStatus = x.deploymentStatus
this.deploymentStatusMessage = x.deploymentStatusMessage
this.environmentName = x.environmentName
this.id = x.id
this.initialState = x.initialState
this.lastAttemptedDeploymentId = x.lastAttemptedDeploymentId
this.lastModifiedAt = x.lastModifiedAt
this.lastSucceededDeploymentId = x.lastSucceededDeploymentId
this.serviceInstanceName = x.serviceInstanceName
this.serviceName = x.serviceName
this.targetArn = x.targetArn
this.targetResourceCreatedAt = x.targetResourceCreatedAt
this.targetResourceType = x.targetResourceType
this.targetState = x.targetState
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.proton.model.Deployment = Deployment(this)
internal fun correctErrors(): Builder {
if (arn == null) arn = ""
if (createdAt == null) createdAt = Instant.fromEpochSeconds(0)
if (deploymentStatus == null) deploymentStatus = DeploymentStatus.SdkUnknown("no value provided")
if (environmentName == null) environmentName = ""
if (id == null) id = ""
if (lastModifiedAt == null) lastModifiedAt = Instant.fromEpochSeconds(0)
if (targetArn == null) targetArn = ""
if (targetResourceCreatedAt == null) targetResourceCreatedAt = Instant.fromEpochSeconds(0)
if (targetResourceType == null) targetResourceType = DeploymentTargetResourceType.SdkUnknown("no value provided")
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy