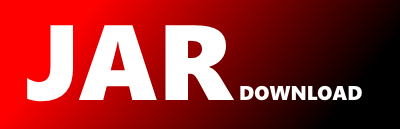
commonMain.aws.sdk.kotlin.services.proton.model.ServicePipeline.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of proton-jvm Show documentation
Show all versions of proton-jvm Show documentation
The AWS SDK for Kotlin client for Proton
The newest version!
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.proton.model
import aws.smithy.kotlin.runtime.SdkDsl
import aws.smithy.kotlin.runtime.time.Instant
/**
* Detailed data of an Proton service instance pipeline resource.
*/
public class ServicePipeline private constructor(builder: Builder) {
/**
* The Amazon Resource Name (ARN) of the service pipeline.
*/
public val arn: kotlin.String = requireNotNull(builder.arn) { "A non-null value must be provided for arn" }
/**
* The time when the service pipeline was created.
*/
public val createdAt: aws.smithy.kotlin.runtime.time.Instant = requireNotNull(builder.createdAt) { "A non-null value must be provided for createdAt" }
/**
* The deployment status of the service pipeline.
*/
public val deploymentStatus: aws.sdk.kotlin.services.proton.model.DeploymentStatus = requireNotNull(builder.deploymentStatus) { "A non-null value must be provided for deploymentStatus" }
/**
* A service pipeline deployment status message.
*/
public val deploymentStatusMessage: kotlin.String? = builder.deploymentStatusMessage
/**
* The ID of the last attempted deployment of this service pipeline.
*/
public val lastAttemptedDeploymentId: kotlin.String? = builder.lastAttemptedDeploymentId
/**
* The time when a deployment of the service pipeline was last attempted.
*/
public val lastDeploymentAttemptedAt: aws.smithy.kotlin.runtime.time.Instant = requireNotNull(builder.lastDeploymentAttemptedAt) { "A non-null value must be provided for lastDeploymentAttemptedAt" }
/**
* The time when the service pipeline was last deployed successfully.
*/
public val lastDeploymentSucceededAt: aws.smithy.kotlin.runtime.time.Instant = requireNotNull(builder.lastDeploymentSucceededAt) { "A non-null value must be provided for lastDeploymentSucceededAt" }
/**
* The ID of the last successful deployment of this service pipeline.
*/
public val lastSucceededDeploymentId: kotlin.String? = builder.lastSucceededDeploymentId
/**
* The service spec that was used to create the service pipeline.
*/
public val spec: kotlin.String? = builder.spec
/**
* The major version of the service template that was used to create the service pipeline.
*/
public val templateMajorVersion: kotlin.String = requireNotNull(builder.templateMajorVersion) { "A non-null value must be provided for templateMajorVersion" }
/**
* The minor version of the service template that was used to create the service pipeline.
*/
public val templateMinorVersion: kotlin.String = requireNotNull(builder.templateMinorVersion) { "A non-null value must be provided for templateMinorVersion" }
/**
* The name of the service template that was used to create the service pipeline.
*/
public val templateName: kotlin.String = requireNotNull(builder.templateName) { "A non-null value must be provided for templateName" }
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.proton.model.ServicePipeline = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("ServicePipeline(")
append("arn=$arn,")
append("createdAt=$createdAt,")
append("deploymentStatus=$deploymentStatus,")
append("deploymentStatusMessage=*** Sensitive Data Redacted ***,")
append("lastAttemptedDeploymentId=$lastAttemptedDeploymentId,")
append("lastDeploymentAttemptedAt=$lastDeploymentAttemptedAt,")
append("lastDeploymentSucceededAt=$lastDeploymentSucceededAt,")
append("lastSucceededDeploymentId=$lastSucceededDeploymentId,")
append("spec=*** Sensitive Data Redacted ***,")
append("templateMajorVersion=$templateMajorVersion,")
append("templateMinorVersion=$templateMinorVersion,")
append("templateName=$templateName")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = arn.hashCode()
result = 31 * result + (createdAt.hashCode())
result = 31 * result + (deploymentStatus.hashCode())
result = 31 * result + (deploymentStatusMessage?.hashCode() ?: 0)
result = 31 * result + (lastAttemptedDeploymentId?.hashCode() ?: 0)
result = 31 * result + (lastDeploymentAttemptedAt.hashCode())
result = 31 * result + (lastDeploymentSucceededAt.hashCode())
result = 31 * result + (lastSucceededDeploymentId?.hashCode() ?: 0)
result = 31 * result + (spec?.hashCode() ?: 0)
result = 31 * result + (templateMajorVersion.hashCode())
result = 31 * result + (templateMinorVersion.hashCode())
result = 31 * result + (templateName.hashCode())
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as ServicePipeline
if (arn != other.arn) return false
if (createdAt != other.createdAt) return false
if (deploymentStatus != other.deploymentStatus) return false
if (deploymentStatusMessage != other.deploymentStatusMessage) return false
if (lastAttemptedDeploymentId != other.lastAttemptedDeploymentId) return false
if (lastDeploymentAttemptedAt != other.lastDeploymentAttemptedAt) return false
if (lastDeploymentSucceededAt != other.lastDeploymentSucceededAt) return false
if (lastSucceededDeploymentId != other.lastSucceededDeploymentId) return false
if (spec != other.spec) return false
if (templateMajorVersion != other.templateMajorVersion) return false
if (templateMinorVersion != other.templateMinorVersion) return false
if (templateName != other.templateName) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.proton.model.ServicePipeline = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The Amazon Resource Name (ARN) of the service pipeline.
*/
public var arn: kotlin.String? = null
/**
* The time when the service pipeline was created.
*/
public var createdAt: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The deployment status of the service pipeline.
*/
public var deploymentStatus: aws.sdk.kotlin.services.proton.model.DeploymentStatus? = null
/**
* A service pipeline deployment status message.
*/
public var deploymentStatusMessage: kotlin.String? = null
/**
* The ID of the last attempted deployment of this service pipeline.
*/
public var lastAttemptedDeploymentId: kotlin.String? = null
/**
* The time when a deployment of the service pipeline was last attempted.
*/
public var lastDeploymentAttemptedAt: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The time when the service pipeline was last deployed successfully.
*/
public var lastDeploymentSucceededAt: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The ID of the last successful deployment of this service pipeline.
*/
public var lastSucceededDeploymentId: kotlin.String? = null
/**
* The service spec that was used to create the service pipeline.
*/
public var spec: kotlin.String? = null
/**
* The major version of the service template that was used to create the service pipeline.
*/
public var templateMajorVersion: kotlin.String? = null
/**
* The minor version of the service template that was used to create the service pipeline.
*/
public var templateMinorVersion: kotlin.String? = null
/**
* The name of the service template that was used to create the service pipeline.
*/
public var templateName: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.proton.model.ServicePipeline) : this() {
this.arn = x.arn
this.createdAt = x.createdAt
this.deploymentStatus = x.deploymentStatus
this.deploymentStatusMessage = x.deploymentStatusMessage
this.lastAttemptedDeploymentId = x.lastAttemptedDeploymentId
this.lastDeploymentAttemptedAt = x.lastDeploymentAttemptedAt
this.lastDeploymentSucceededAt = x.lastDeploymentSucceededAt
this.lastSucceededDeploymentId = x.lastSucceededDeploymentId
this.spec = x.spec
this.templateMajorVersion = x.templateMajorVersion
this.templateMinorVersion = x.templateMinorVersion
this.templateName = x.templateName
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.proton.model.ServicePipeline = ServicePipeline(this)
internal fun correctErrors(): Builder {
if (arn == null) arn = ""
if (createdAt == null) createdAt = Instant.fromEpochSeconds(0)
if (deploymentStatus == null) deploymentStatus = DeploymentStatus.SdkUnknown("no value provided")
if (lastDeploymentAttemptedAt == null) lastDeploymentAttemptedAt = Instant.fromEpochSeconds(0)
if (lastDeploymentSucceededAt == null) lastDeploymentSucceededAt = Instant.fromEpochSeconds(0)
if (templateMajorVersion == null) templateMajorVersion = ""
if (templateMinorVersion == null) templateMinorVersion = ""
if (templateName == null) templateName = ""
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy