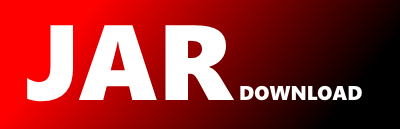
commonMain.aws.sdk.kotlin.services.qldb.model.JournalS3ExportDescription.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.qldb.model
import aws.smithy.kotlin.runtime.SdkDsl
import aws.smithy.kotlin.runtime.time.Instant
/**
* Information about a journal export job, including the ledger name, export ID, creation time, current status, and the parameters of the original export creation request.
*/
public class JournalS3ExportDescription private constructor(builder: Builder) {
/**
* The exclusive end date and time for the range of journal contents that was specified in the original export request.
*/
public val exclusiveEndTime: aws.smithy.kotlin.runtime.time.Instant = requireNotNull(builder.exclusiveEndTime) { "A non-null value must be provided for exclusiveEndTime" }
/**
* The date and time, in epoch time format, when the export job was created. (Epoch time format is the number of seconds elapsed since 12:00:00 AM January 1, 1970 UTC.)
*/
public val exportCreationTime: aws.smithy.kotlin.runtime.time.Instant = requireNotNull(builder.exportCreationTime) { "A non-null value must be provided for exportCreationTime" }
/**
* The UUID (represented in Base62-encoded text) of the journal export job.
*/
public val exportId: kotlin.String = requireNotNull(builder.exportId) { "A non-null value must be provided for exportId" }
/**
* The inclusive start date and time for the range of journal contents that was specified in the original export request.
*/
public val inclusiveStartTime: aws.smithy.kotlin.runtime.time.Instant = requireNotNull(builder.inclusiveStartTime) { "A non-null value must be provided for inclusiveStartTime" }
/**
* The name of the ledger.
*/
public val ledgerName: kotlin.String = requireNotNull(builder.ledgerName) { "A non-null value must be provided for ledgerName" }
/**
* The output format of the exported journal data.
*/
public val outputFormat: aws.sdk.kotlin.services.qldb.model.OutputFormat? = builder.outputFormat
/**
* The Amazon Resource Name (ARN) of the IAM role that grants QLDB permissions for a journal export job to do the following:
* + Write objects into your Amazon Simple Storage Service (Amazon S3) bucket.
* + (Optional) Use your customer managed key in Key Management Service (KMS) for server-side encryption of your exported data.
*/
public val roleArn: kotlin.String = requireNotNull(builder.roleArn) { "A non-null value must be provided for roleArn" }
/**
* The Amazon Simple Storage Service (Amazon S3) bucket location in which a journal export job writes the journal contents.
*/
public val s3ExportConfiguration: aws.sdk.kotlin.services.qldb.model.S3ExportConfiguration? = builder.s3ExportConfiguration
/**
* The current state of the journal export job.
*/
public val status: aws.sdk.kotlin.services.qldb.model.ExportStatus = requireNotNull(builder.status) { "A non-null value must be provided for status" }
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.qldb.model.JournalS3ExportDescription = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("JournalS3ExportDescription(")
append("exclusiveEndTime=$exclusiveEndTime,")
append("exportCreationTime=$exportCreationTime,")
append("exportId=$exportId,")
append("inclusiveStartTime=$inclusiveStartTime,")
append("ledgerName=$ledgerName,")
append("outputFormat=$outputFormat,")
append("roleArn=$roleArn,")
append("s3ExportConfiguration=$s3ExportConfiguration,")
append("status=$status")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = exclusiveEndTime.hashCode()
result = 31 * result + (exportCreationTime.hashCode())
result = 31 * result + (exportId.hashCode())
result = 31 * result + (inclusiveStartTime.hashCode())
result = 31 * result + (ledgerName.hashCode())
result = 31 * result + (outputFormat?.hashCode() ?: 0)
result = 31 * result + (roleArn.hashCode())
result = 31 * result + (s3ExportConfiguration?.hashCode() ?: 0)
result = 31 * result + (status.hashCode())
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as JournalS3ExportDescription
if (exclusiveEndTime != other.exclusiveEndTime) return false
if (exportCreationTime != other.exportCreationTime) return false
if (exportId != other.exportId) return false
if (inclusiveStartTime != other.inclusiveStartTime) return false
if (ledgerName != other.ledgerName) return false
if (outputFormat != other.outputFormat) return false
if (roleArn != other.roleArn) return false
if (s3ExportConfiguration != other.s3ExportConfiguration) return false
if (status != other.status) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.qldb.model.JournalS3ExportDescription = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The exclusive end date and time for the range of journal contents that was specified in the original export request.
*/
public var exclusiveEndTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The date and time, in epoch time format, when the export job was created. (Epoch time format is the number of seconds elapsed since 12:00:00 AM January 1, 1970 UTC.)
*/
public var exportCreationTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The UUID (represented in Base62-encoded text) of the journal export job.
*/
public var exportId: kotlin.String? = null
/**
* The inclusive start date and time for the range of journal contents that was specified in the original export request.
*/
public var inclusiveStartTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The name of the ledger.
*/
public var ledgerName: kotlin.String? = null
/**
* The output format of the exported journal data.
*/
public var outputFormat: aws.sdk.kotlin.services.qldb.model.OutputFormat? = null
/**
* The Amazon Resource Name (ARN) of the IAM role that grants QLDB permissions for a journal export job to do the following:
* + Write objects into your Amazon Simple Storage Service (Amazon S3) bucket.
* + (Optional) Use your customer managed key in Key Management Service (KMS) for server-side encryption of your exported data.
*/
public var roleArn: kotlin.String? = null
/**
* The Amazon Simple Storage Service (Amazon S3) bucket location in which a journal export job writes the journal contents.
*/
public var s3ExportConfiguration: aws.sdk.kotlin.services.qldb.model.S3ExportConfiguration? = null
/**
* The current state of the journal export job.
*/
public var status: aws.sdk.kotlin.services.qldb.model.ExportStatus? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.qldb.model.JournalS3ExportDescription) : this() {
this.exclusiveEndTime = x.exclusiveEndTime
this.exportCreationTime = x.exportCreationTime
this.exportId = x.exportId
this.inclusiveStartTime = x.inclusiveStartTime
this.ledgerName = x.ledgerName
this.outputFormat = x.outputFormat
this.roleArn = x.roleArn
this.s3ExportConfiguration = x.s3ExportConfiguration
this.status = x.status
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.qldb.model.JournalS3ExportDescription = JournalS3ExportDescription(this)
/**
* construct an [aws.sdk.kotlin.services.qldb.model.S3ExportConfiguration] inside the given [block]
*/
public fun s3ExportConfiguration(block: aws.sdk.kotlin.services.qldb.model.S3ExportConfiguration.Builder.() -> kotlin.Unit) {
this.s3ExportConfiguration = aws.sdk.kotlin.services.qldb.model.S3ExportConfiguration.invoke(block)
}
internal fun correctErrors(): Builder {
if (exclusiveEndTime == null) exclusiveEndTime = Instant.fromEpochSeconds(0)
if (exportCreationTime == null) exportCreationTime = Instant.fromEpochSeconds(0)
if (exportId == null) exportId = ""
if (inclusiveStartTime == null) inclusiveStartTime = Instant.fromEpochSeconds(0)
if (ledgerName == null) ledgerName = ""
if (roleArn == null) roleArn = ""
if (status == null) status = ExportStatus.SdkUnknown("no value provided")
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy