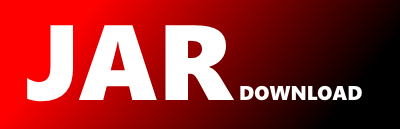
commonMain.aws.sdk.kotlin.services.rdsdata.model.BatchExecuteStatementRequest.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rdsdata-jvm Show documentation
Show all versions of rdsdata-jvm Show documentation
The AWS SDK for Kotlin client for RDS Data
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.rdsdata.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* The request parameters represent the input of a SQL statement over an array of data.
*/
public class BatchExecuteStatementRequest private constructor(builder: Builder) {
/**
* The name of the database.
*/
public val database: kotlin.String? = builder.database
/**
* The parameter set for the batch operation.
*
* The SQL statement is executed as many times as the number of parameter sets provided. To execute a SQL statement with no parameters, use one of the following options:
* + Specify one or more empty parameter sets.
* + Use the `ExecuteStatement` operation instead of the `BatchExecuteStatement` operation.
*
* Array parameters are not supported.
*/
public val parameterSets: List>? = builder.parameterSets
/**
* The Amazon Resource Name (ARN) of the Aurora Serverless DB cluster.
*/
public val resourceArn: kotlin.String = requireNotNull(builder.resourceArn) { "A non-null value must be provided for resourceArn" }
/**
* The name of the database schema.
*
* Currently, the `schema` parameter isn't supported.
*/
public val schema: kotlin.String? = builder.schema
/**
* The ARN of the secret that enables access to the DB cluster. Enter the database user name and password for the credentials in the secret.
*
* For information about creating the secret, see [Create a database secret](https://docs.aws.amazon.com/secretsmanager/latest/userguide/create_database_secret.html).
*/
public val secretArn: kotlin.String = requireNotNull(builder.secretArn) { "A non-null value must be provided for secretArn" }
/**
* The SQL statement to run. Don't include a semicolon (;) at the end of the SQL statement.
*/
public val sql: kotlin.String = requireNotNull(builder.sql) { "A non-null value must be provided for sql" }
/**
* The identifier of a transaction that was started by using the `BeginTransaction` operation. Specify the transaction ID of the transaction that you want to include the SQL statement in.
*
* If the SQL statement is not part of a transaction, don't set this parameter.
*/
public val transactionId: kotlin.String? = builder.transactionId
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.rdsdata.model.BatchExecuteStatementRequest = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("BatchExecuteStatementRequest(")
append("database=$database,")
append("parameterSets=$parameterSets,")
append("resourceArn=$resourceArn,")
append("schema=$schema,")
append("secretArn=$secretArn,")
append("sql=$sql,")
append("transactionId=$transactionId")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = database?.hashCode() ?: 0
result = 31 * result + (parameterSets?.hashCode() ?: 0)
result = 31 * result + (resourceArn.hashCode())
result = 31 * result + (schema?.hashCode() ?: 0)
result = 31 * result + (secretArn.hashCode())
result = 31 * result + (sql.hashCode())
result = 31 * result + (transactionId?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as BatchExecuteStatementRequest
if (database != other.database) return false
if (parameterSets != other.parameterSets) return false
if (resourceArn != other.resourceArn) return false
if (schema != other.schema) return false
if (secretArn != other.secretArn) return false
if (sql != other.sql) return false
if (transactionId != other.transactionId) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.rdsdata.model.BatchExecuteStatementRequest = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The name of the database.
*/
public var database: kotlin.String? = null
/**
* The parameter set for the batch operation.
*
* The SQL statement is executed as many times as the number of parameter sets provided. To execute a SQL statement with no parameters, use one of the following options:
* + Specify one or more empty parameter sets.
* + Use the `ExecuteStatement` operation instead of the `BatchExecuteStatement` operation.
*
* Array parameters are not supported.
*/
public var parameterSets: List>? = null
/**
* The Amazon Resource Name (ARN) of the Aurora Serverless DB cluster.
*/
public var resourceArn: kotlin.String? = null
/**
* The name of the database schema.
*
* Currently, the `schema` parameter isn't supported.
*/
public var schema: kotlin.String? = null
/**
* The ARN of the secret that enables access to the DB cluster. Enter the database user name and password for the credentials in the secret.
*
* For information about creating the secret, see [Create a database secret](https://docs.aws.amazon.com/secretsmanager/latest/userguide/create_database_secret.html).
*/
public var secretArn: kotlin.String? = null
/**
* The SQL statement to run. Don't include a semicolon (;) at the end of the SQL statement.
*/
public var sql: kotlin.String? = null
/**
* The identifier of a transaction that was started by using the `BeginTransaction` operation. Specify the transaction ID of the transaction that you want to include the SQL statement in.
*
* If the SQL statement is not part of a transaction, don't set this parameter.
*/
public var transactionId: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.rdsdata.model.BatchExecuteStatementRequest) : this() {
this.database = x.database
this.parameterSets = x.parameterSets
this.resourceArn = x.resourceArn
this.schema = x.schema
this.secretArn = x.secretArn
this.sql = x.sql
this.transactionId = x.transactionId
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.rdsdata.model.BatchExecuteStatementRequest = BatchExecuteStatementRequest(this)
internal fun correctErrors(): Builder {
if (resourceArn == null) resourceArn = ""
if (secretArn == null) secretArn = ""
if (sql == null) sql = ""
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy