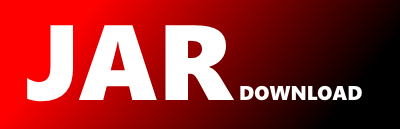
commonMain.aws.sdk.kotlin.services.redshift.model.CreateIntegrationRequest.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of redshift-jvm Show documentation
Show all versions of redshift-jvm Show documentation
The AWS SDK for Kotlin client for Redshift
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.redshift.model
import aws.smithy.kotlin.runtime.SdkDsl
public class CreateIntegrationRequest private constructor(builder: Builder) {
/**
* An optional set of non-secret key–value pairs that contains additional contextual information about the data. For more information, see [Encryption context](https://docs.aws.amazon.com/kms/latest/developerguide/concepts.html#encrypt_context) in the *Amazon Web Services Key Management Service Developer Guide*.
*
* You can only include this parameter if you specify the `KMSKeyId` parameter.
*/
public val additionalEncryptionContext: Map? = builder.additionalEncryptionContext
/**
* A description of the integration.
*/
public val description: kotlin.String? = builder.description
/**
* The name of the integration.
*/
public val integrationName: kotlin.String? = builder.integrationName
/**
* An Key Management Service (KMS) key identifier for the key to use to encrypt the integration. If you don't specify an encryption key, the default Amazon Web Services owned key is used.
*/
public val kmsKeyId: kotlin.String? = builder.kmsKeyId
/**
* The Amazon Resource Name (ARN) of the database to use as the source for replication.
*/
public val sourceArn: kotlin.String? = builder.sourceArn
/**
* A list of tags.
*/
public val tagList: List? = builder.tagList
/**
* The Amazon Resource Name (ARN) of the Amazon Redshift data warehouse to use as the target for replication.
*/
public val targetArn: kotlin.String? = builder.targetArn
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.redshift.model.CreateIntegrationRequest = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("CreateIntegrationRequest(")
append("additionalEncryptionContext=$additionalEncryptionContext,")
append("description=$description,")
append("integrationName=$integrationName,")
append("kmsKeyId=$kmsKeyId,")
append("sourceArn=$sourceArn,")
append("tagList=$tagList,")
append("targetArn=$targetArn")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = additionalEncryptionContext?.hashCode() ?: 0
result = 31 * result + (description?.hashCode() ?: 0)
result = 31 * result + (integrationName?.hashCode() ?: 0)
result = 31 * result + (kmsKeyId?.hashCode() ?: 0)
result = 31 * result + (sourceArn?.hashCode() ?: 0)
result = 31 * result + (tagList?.hashCode() ?: 0)
result = 31 * result + (targetArn?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as CreateIntegrationRequest
if (additionalEncryptionContext != other.additionalEncryptionContext) return false
if (description != other.description) return false
if (integrationName != other.integrationName) return false
if (kmsKeyId != other.kmsKeyId) return false
if (sourceArn != other.sourceArn) return false
if (tagList != other.tagList) return false
if (targetArn != other.targetArn) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.redshift.model.CreateIntegrationRequest = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* An optional set of non-secret key–value pairs that contains additional contextual information about the data. For more information, see [Encryption context](https://docs.aws.amazon.com/kms/latest/developerguide/concepts.html#encrypt_context) in the *Amazon Web Services Key Management Service Developer Guide*.
*
* You can only include this parameter if you specify the `KMSKeyId` parameter.
*/
public var additionalEncryptionContext: Map? = null
/**
* A description of the integration.
*/
public var description: kotlin.String? = null
/**
* The name of the integration.
*/
public var integrationName: kotlin.String? = null
/**
* An Key Management Service (KMS) key identifier for the key to use to encrypt the integration. If you don't specify an encryption key, the default Amazon Web Services owned key is used.
*/
public var kmsKeyId: kotlin.String? = null
/**
* The Amazon Resource Name (ARN) of the database to use as the source for replication.
*/
public var sourceArn: kotlin.String? = null
/**
* A list of tags.
*/
public var tagList: List? = null
/**
* The Amazon Resource Name (ARN) of the Amazon Redshift data warehouse to use as the target for replication.
*/
public var targetArn: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.redshift.model.CreateIntegrationRequest) : this() {
this.additionalEncryptionContext = x.additionalEncryptionContext
this.description = x.description
this.integrationName = x.integrationName
this.kmsKeyId = x.kmsKeyId
this.sourceArn = x.sourceArn
this.tagList = x.tagList
this.targetArn = x.targetArn
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.redshift.model.CreateIntegrationRequest = CreateIntegrationRequest(this)
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy