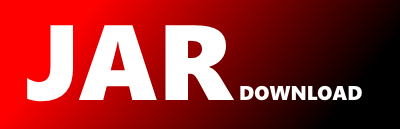
commonMain.aws.sdk.kotlin.services.redshiftdata.model.DescribeStatementResponse.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of redshiftdata Show documentation
Show all versions of redshiftdata Show documentation
The AWS Kotlin client for Redshift Data
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.redshiftdata.model
import aws.smithy.kotlin.runtime.time.Instant
public class DescribeStatementResponse private constructor(builder: Builder) {
/**
* The cluster identifier.
*/
public val clusterIdentifier: kotlin.String? = builder.clusterIdentifier
/**
* The date and time (UTC) when the SQL statement was submitted to run.
*/
public val createdAt: aws.smithy.kotlin.runtime.time.Instant? = builder.createdAt
/**
* The name of the database.
*/
public val database: kotlin.String? = builder.database
/**
* The database user name.
*/
public val dbUser: kotlin.String? = builder.dbUser
/**
* The amount of time in nanoseconds that the statement ran.
*/
public val duration: kotlin.Long = builder.duration
/**
* The error message from the cluster if the SQL statement encountered an error while running.
*/
public val error: kotlin.String? = builder.error
/**
* A value that indicates whether the statement has a result set. The result set can be empty. The value is true for an empty result set. The value is true if any substatement returns a result set.
*/
public val hasResultSet: kotlin.Boolean? = builder.hasResultSet
/**
* The identifier of the SQL statement described. This value is a universally unique identifier (UUID) generated by Amazon Redshift Data API.
*/
public val id: kotlin.String? = builder.id
/**
* The parameters for the SQL statement.
*/
public val queryParameters: List? = builder.queryParameters
/**
* The SQL statement text.
*/
public val queryString: kotlin.String? = builder.queryString
/**
* The process identifier from Amazon Redshift.
*/
public val redshiftPid: kotlin.Long = builder.redshiftPid
/**
* The identifier of the query generated by Amazon Redshift. These identifiers are also available in the `query` column of the `STL_QUERY` system view.
*/
public val redshiftQueryId: kotlin.Long = builder.redshiftQueryId
/**
* Either the number of rows returned from the SQL statement or the number of rows affected. If result size is greater than zero, the result rows can be the number of rows affected by SQL statements such as INSERT, UPDATE, DELETE, COPY, and others. A `-1` indicates the value is null.
*/
public val resultRows: kotlin.Long = builder.resultRows
/**
* The size in bytes of the returned results. A `-1` indicates the value is null.
*/
public val resultSize: kotlin.Long = builder.resultSize
/**
* The name or Amazon Resource Name (ARN) of the secret that enables access to the database.
*/
public val secretArn: kotlin.String? = builder.secretArn
/**
* The status of the SQL statement being described. Status values are defined as follows:
* + ABORTED - The query run was stopped by the user.
* + ALL - A status value that includes all query statuses. This value can be used to filter results.
* + FAILED - The query run failed.
* + FINISHED - The query has finished running.
* + PICKED - The query has been chosen to be run.
* + STARTED - The query run has started.
* + SUBMITTED - The query was submitted, but not yet processed.
*/
public val status: aws.sdk.kotlin.services.redshiftdata.model.StatusString? = builder.status
/**
* The SQL statements from a multiple statement run.
*/
public val subStatements: List? = builder.subStatements
/**
* The date and time (UTC) that the metadata for the SQL statement was last updated. An example is the time the status last changed.
*/
public val updatedAt: aws.smithy.kotlin.runtime.time.Instant? = builder.updatedAt
/**
* The serverless workgroup name.
*/
public val workgroupName: kotlin.String? = builder.workgroupName
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.redshiftdata.model.DescribeStatementResponse = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("DescribeStatementResponse(")
append("clusterIdentifier=$clusterIdentifier,")
append("createdAt=$createdAt,")
append("database=$database,")
append("dbUser=$dbUser,")
append("duration=$duration,")
append("error=$error,")
append("hasResultSet=$hasResultSet,")
append("id=$id,")
append("queryParameters=$queryParameters,")
append("queryString=$queryString,")
append("redshiftPid=$redshiftPid,")
append("redshiftQueryId=$redshiftQueryId,")
append("resultRows=$resultRows,")
append("resultSize=$resultSize,")
append("secretArn=$secretArn,")
append("status=$status,")
append("subStatements=$subStatements,")
append("updatedAt=$updatedAt,")
append("workgroupName=$workgroupName)")
}
override fun hashCode(): kotlin.Int {
var result = clusterIdentifier?.hashCode() ?: 0
result = 31 * result + (createdAt?.hashCode() ?: 0)
result = 31 * result + (database?.hashCode() ?: 0)
result = 31 * result + (dbUser?.hashCode() ?: 0)
result = 31 * result + (duration.hashCode())
result = 31 * result + (error?.hashCode() ?: 0)
result = 31 * result + (hasResultSet?.hashCode() ?: 0)
result = 31 * result + (id?.hashCode() ?: 0)
result = 31 * result + (queryParameters?.hashCode() ?: 0)
result = 31 * result + (queryString?.hashCode() ?: 0)
result = 31 * result + (redshiftPid.hashCode())
result = 31 * result + (redshiftQueryId.hashCode())
result = 31 * result + (resultRows.hashCode())
result = 31 * result + (resultSize.hashCode())
result = 31 * result + (secretArn?.hashCode() ?: 0)
result = 31 * result + (status?.hashCode() ?: 0)
result = 31 * result + (subStatements?.hashCode() ?: 0)
result = 31 * result + (updatedAt?.hashCode() ?: 0)
result = 31 * result + (workgroupName?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as DescribeStatementResponse
if (clusterIdentifier != other.clusterIdentifier) return false
if (createdAt != other.createdAt) return false
if (database != other.database) return false
if (dbUser != other.dbUser) return false
if (duration != other.duration) return false
if (error != other.error) return false
if (hasResultSet != other.hasResultSet) return false
if (id != other.id) return false
if (queryParameters != other.queryParameters) return false
if (queryString != other.queryString) return false
if (redshiftPid != other.redshiftPid) return false
if (redshiftQueryId != other.redshiftQueryId) return false
if (resultRows != other.resultRows) return false
if (resultSize != other.resultSize) return false
if (secretArn != other.secretArn) return false
if (status != other.status) return false
if (subStatements != other.subStatements) return false
if (updatedAt != other.updatedAt) return false
if (workgroupName != other.workgroupName) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.redshiftdata.model.DescribeStatementResponse = Builder(this).apply(block).build()
public class Builder {
/**
* The cluster identifier.
*/
public var clusterIdentifier: kotlin.String? = null
/**
* The date and time (UTC) when the SQL statement was submitted to run.
*/
public var createdAt: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The name of the database.
*/
public var database: kotlin.String? = null
/**
* The database user name.
*/
public var dbUser: kotlin.String? = null
/**
* The amount of time in nanoseconds that the statement ran.
*/
public var duration: kotlin.Long = 0L
/**
* The error message from the cluster if the SQL statement encountered an error while running.
*/
public var error: kotlin.String? = null
/**
* A value that indicates whether the statement has a result set. The result set can be empty. The value is true for an empty result set. The value is true if any substatement returns a result set.
*/
public var hasResultSet: kotlin.Boolean? = null
/**
* The identifier of the SQL statement described. This value is a universally unique identifier (UUID) generated by Amazon Redshift Data API.
*/
public var id: kotlin.String? = null
/**
* The parameters for the SQL statement.
*/
public var queryParameters: List? = null
/**
* The SQL statement text.
*/
public var queryString: kotlin.String? = null
/**
* The process identifier from Amazon Redshift.
*/
public var redshiftPid: kotlin.Long = 0L
/**
* The identifier of the query generated by Amazon Redshift. These identifiers are also available in the `query` column of the `STL_QUERY` system view.
*/
public var redshiftQueryId: kotlin.Long = 0L
/**
* Either the number of rows returned from the SQL statement or the number of rows affected. If result size is greater than zero, the result rows can be the number of rows affected by SQL statements such as INSERT, UPDATE, DELETE, COPY, and others. A `-1` indicates the value is null.
*/
public var resultRows: kotlin.Long = 0L
/**
* The size in bytes of the returned results. A `-1` indicates the value is null.
*/
public var resultSize: kotlin.Long = 0L
/**
* The name or Amazon Resource Name (ARN) of the secret that enables access to the database.
*/
public var secretArn: kotlin.String? = null
/**
* The status of the SQL statement being described. Status values are defined as follows:
* + ABORTED - The query run was stopped by the user.
* + ALL - A status value that includes all query statuses. This value can be used to filter results.
* + FAILED - The query run failed.
* + FINISHED - The query has finished running.
* + PICKED - The query has been chosen to be run.
* + STARTED - The query run has started.
* + SUBMITTED - The query was submitted, but not yet processed.
*/
public var status: aws.sdk.kotlin.services.redshiftdata.model.StatusString? = null
/**
* The SQL statements from a multiple statement run.
*/
public var subStatements: List? = null
/**
* The date and time (UTC) that the metadata for the SQL statement was last updated. An example is the time the status last changed.
*/
public var updatedAt: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The serverless workgroup name.
*/
public var workgroupName: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.redshiftdata.model.DescribeStatementResponse) : this() {
this.clusterIdentifier = x.clusterIdentifier
this.createdAt = x.createdAt
this.database = x.database
this.dbUser = x.dbUser
this.duration = x.duration
this.error = x.error
this.hasResultSet = x.hasResultSet
this.id = x.id
this.queryParameters = x.queryParameters
this.queryString = x.queryString
this.redshiftPid = x.redshiftPid
this.redshiftQueryId = x.redshiftQueryId
this.resultRows = x.resultRows
this.resultSize = x.resultSize
this.secretArn = x.secretArn
this.status = x.status
this.subStatements = x.subStatements
this.updatedAt = x.updatedAt
this.workgroupName = x.workgroupName
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.redshiftdata.model.DescribeStatementResponse = DescribeStatementResponse(this)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy