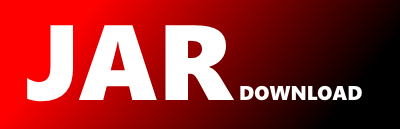
commonMain.aws.sdk.kotlin.services.redshiftdata.model.StatementData.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of redshiftdata Show documentation
Show all versions of redshiftdata Show documentation
The AWS Kotlin client for Redshift Data
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.redshiftdata.model
import aws.smithy.kotlin.runtime.time.Instant
/**
* The SQL statement to run.
*/
public class StatementData private constructor(builder: Builder) {
/**
* The date and time (UTC) the statement was created.
*/
public val createdAt: aws.smithy.kotlin.runtime.time.Instant? = builder.createdAt
/**
* The SQL statement identifier. This value is a universally unique identifier (UUID) generated by Amazon Redshift Data API.
*/
public val id: kotlin.String? = builder.id
/**
* A value that indicates whether the statement is a batch query request.
*/
public val isBatchStatement: kotlin.Boolean? = builder.isBatchStatement
/**
* The parameters used in a SQL statement.
*/
public val queryParameters: List? = builder.queryParameters
/**
* The SQL statement.
*/
public val queryString: kotlin.String? = builder.queryString
/**
* One or more SQL statements. Each query string in the array corresponds to one of the queries in a batch query request.
*/
public val queryStrings: List? = builder.queryStrings
/**
* The name or Amazon Resource Name (ARN) of the secret that enables access to the database.
*/
public val secretArn: kotlin.String? = builder.secretArn
/**
* The name of the SQL statement.
*/
public val statementName: kotlin.String? = builder.statementName
/**
* The status of the SQL statement. An example is the that the SQL statement finished.
*/
public val status: aws.sdk.kotlin.services.redshiftdata.model.StatusString? = builder.status
/**
* The date and time (UTC) that the statement metadata was last updated.
*/
public val updatedAt: aws.smithy.kotlin.runtime.time.Instant? = builder.updatedAt
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.redshiftdata.model.StatementData = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("StatementData(")
append("createdAt=$createdAt,")
append("id=$id,")
append("isBatchStatement=$isBatchStatement,")
append("queryParameters=$queryParameters,")
append("queryString=$queryString,")
append("queryStrings=$queryStrings,")
append("secretArn=$secretArn,")
append("statementName=$statementName,")
append("status=$status,")
append("updatedAt=$updatedAt)")
}
override fun hashCode(): kotlin.Int {
var result = createdAt?.hashCode() ?: 0
result = 31 * result + (id?.hashCode() ?: 0)
result = 31 * result + (isBatchStatement?.hashCode() ?: 0)
result = 31 * result + (queryParameters?.hashCode() ?: 0)
result = 31 * result + (queryString?.hashCode() ?: 0)
result = 31 * result + (queryStrings?.hashCode() ?: 0)
result = 31 * result + (secretArn?.hashCode() ?: 0)
result = 31 * result + (statementName?.hashCode() ?: 0)
result = 31 * result + (status?.hashCode() ?: 0)
result = 31 * result + (updatedAt?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as StatementData
if (createdAt != other.createdAt) return false
if (id != other.id) return false
if (isBatchStatement != other.isBatchStatement) return false
if (queryParameters != other.queryParameters) return false
if (queryString != other.queryString) return false
if (queryStrings != other.queryStrings) return false
if (secretArn != other.secretArn) return false
if (statementName != other.statementName) return false
if (status != other.status) return false
if (updatedAt != other.updatedAt) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.redshiftdata.model.StatementData = Builder(this).apply(block).build()
public class Builder {
/**
* The date and time (UTC) the statement was created.
*/
public var createdAt: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The SQL statement identifier. This value is a universally unique identifier (UUID) generated by Amazon Redshift Data API.
*/
public var id: kotlin.String? = null
/**
* A value that indicates whether the statement is a batch query request.
*/
public var isBatchStatement: kotlin.Boolean? = null
/**
* The parameters used in a SQL statement.
*/
public var queryParameters: List? = null
/**
* The SQL statement.
*/
public var queryString: kotlin.String? = null
/**
* One or more SQL statements. Each query string in the array corresponds to one of the queries in a batch query request.
*/
public var queryStrings: List? = null
/**
* The name or Amazon Resource Name (ARN) of the secret that enables access to the database.
*/
public var secretArn: kotlin.String? = null
/**
* The name of the SQL statement.
*/
public var statementName: kotlin.String? = null
/**
* The status of the SQL statement. An example is the that the SQL statement finished.
*/
public var status: aws.sdk.kotlin.services.redshiftdata.model.StatusString? = null
/**
* The date and time (UTC) that the statement metadata was last updated.
*/
public var updatedAt: aws.smithy.kotlin.runtime.time.Instant? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.redshiftdata.model.StatementData) : this() {
this.createdAt = x.createdAt
this.id = x.id
this.isBatchStatement = x.isBatchStatement
this.queryParameters = x.queryParameters
this.queryString = x.queryString
this.queryStrings = x.queryStrings
this.secretArn = x.secretArn
this.statementName = x.statementName
this.status = x.status
this.updatedAt = x.updatedAt
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.redshiftdata.model.StatementData = StatementData(this)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy