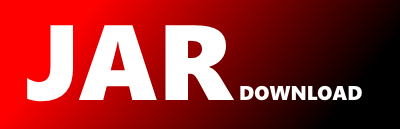
commonMain.aws.sdk.kotlin.services.resourcegroups.DefaultResourceGroupsClient.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of resourcegroups-jvm Show documentation
Show all versions of resourcegroups-jvm Show documentation
The AWS SDK for Kotlin client for Resource Groups
The newest version!
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.resourcegroups
import aws.sdk.kotlin.runtime.http.ApiMetadata
import aws.sdk.kotlin.runtime.http.AwsUserAgentMetadata
import aws.sdk.kotlin.runtime.http.interceptors.AwsSpanInterceptor
import aws.sdk.kotlin.runtime.http.interceptors.BusinessMetricsInterceptor
import aws.sdk.kotlin.runtime.http.middleware.AwsRetryHeaderMiddleware
import aws.sdk.kotlin.runtime.http.middleware.RecursionDetection
import aws.sdk.kotlin.runtime.http.middleware.UserAgent
import aws.sdk.kotlin.services.resourcegroups.auth.ResourceGroupsAuthSchemeProviderAdapter
import aws.sdk.kotlin.services.resourcegroups.auth.ResourceGroupsIdentityProviderConfigAdapter
import aws.sdk.kotlin.services.resourcegroups.endpoints.internal.EndpointResolverAdapter
import aws.sdk.kotlin.services.resourcegroups.model.*
import aws.sdk.kotlin.services.resourcegroups.serde.*
import aws.smithy.kotlin.runtime.auth.AuthSchemeId
import aws.smithy.kotlin.runtime.auth.awssigning.AwsSigningAttributes
import aws.smithy.kotlin.runtime.auth.awssigning.DefaultAwsSigner
import aws.smithy.kotlin.runtime.awsprotocol.AwsAttributes
import aws.smithy.kotlin.runtime.client.SdkClientOption
import aws.smithy.kotlin.runtime.collections.attributesOf
import aws.smithy.kotlin.runtime.collections.putIfAbsent
import aws.smithy.kotlin.runtime.collections.putIfAbsentNotNull
import aws.smithy.kotlin.runtime.http.SdkHttpClient
import aws.smithy.kotlin.runtime.http.auth.AuthScheme
import aws.smithy.kotlin.runtime.http.auth.SigV4AuthScheme
import aws.smithy.kotlin.runtime.http.operation.OperationAuthConfig
import aws.smithy.kotlin.runtime.http.operation.OperationMetrics
import aws.smithy.kotlin.runtime.http.operation.SdkHttpOperation
import aws.smithy.kotlin.runtime.http.operation.context
import aws.smithy.kotlin.runtime.http.operation.roundTrip
import aws.smithy.kotlin.runtime.http.operation.telemetry
import aws.smithy.kotlin.runtime.io.SdkManagedGroup
import aws.smithy.kotlin.runtime.io.addIfManaged
import aws.smithy.kotlin.runtime.operation.ExecutionContext
internal class DefaultResourceGroupsClient(override val config: ResourceGroupsClient.Config) : ResourceGroupsClient {
private val managedResources = SdkManagedGroup()
private val client = SdkHttpClient(config.httpClient)
private val identityProviderConfig = ResourceGroupsIdentityProviderConfigAdapter(config)
private val configuredAuthSchemes = with(config.authSchemes.associateBy(AuthScheme::schemeId).toMutableMap()){
getOrPut(AuthSchemeId.AwsSigV4){
SigV4AuthScheme(DefaultAwsSigner, "resource-groups")
}
toMap()
}
private val authSchemeAdapter = ResourceGroupsAuthSchemeProviderAdapter(config)
private val telemetryScope = "aws.sdk.kotlin.services.resourcegroups"
private val opMetrics = OperationMetrics(telemetryScope, config.telemetryProvider)
init {
managedResources.addIfManaged(config.httpClient)
managedResources.addIfManaged(config.credentialsProvider)
}
private val awsUserAgentMetadata = AwsUserAgentMetadata.fromEnvironment(ApiMetadata(ServiceId, SdkVersion), config.applicationId)
/**
* Cancels the specified tag-sync task.
*
* **Minimum permissions**
*
* To run this command, you must have the following permissions:
* + `resource-groups:CancelTagSyncTask` on the application group
* + `resource-groups:DeleteGroup`
*/
override suspend fun cancelTagSyncTask(input: CancelTagSyncTaskRequest): CancelTagSyncTaskResponse {
val op = SdkHttpOperation.build {
serializeWith = CancelTagSyncTaskOperationSerializer()
deserializeWith = CancelTagSyncTaskOperationDeserializer()
operationName = "CancelTagSyncTask"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Creates a resource group with the specified name and description. You can optionally include either a resource query or a service configuration. For more information about constructing a resource query, see [Build queries and groups in Resource Groups](https://docs.aws.amazon.com/ARG/latest/userguide/getting_started-query.html) in the *Resource Groups User Guide*. For more information about service-linked groups and service configurations, see [Service configurations for Resource Groups](https://docs.aws.amazon.com/ARG/latest/APIReference/about-slg.html).
*
* **Minimum permissions**
*
* To run this command, you must have the following permissions:
* + `resource-groups:CreateGroup`
*/
override suspend fun createGroup(input: CreateGroupRequest): CreateGroupResponse {
val op = SdkHttpOperation.build {
serializeWith = CreateGroupOperationSerializer()
deserializeWith = CreateGroupOperationDeserializer()
operationName = "CreateGroup"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Deletes the specified resource group. Deleting a resource group does not delete any resources that are members of the group; it only deletes the group structure.
*
* **Minimum permissions**
*
* To run this command, you must have the following permissions:
* + `resource-groups:DeleteGroup`
*/
override suspend fun deleteGroup(input: DeleteGroupRequest): DeleteGroupResponse {
val op = SdkHttpOperation.build {
serializeWith = DeleteGroupOperationSerializer()
deserializeWith = DeleteGroupOperationDeserializer()
operationName = "DeleteGroup"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Retrieves the current status of optional features in Resource Groups.
*/
override suspend fun getAccountSettings(input: GetAccountSettingsRequest): GetAccountSettingsResponse {
val op = SdkHttpOperation.build {
serializeWith = GetAccountSettingsOperationSerializer()
deserializeWith = GetAccountSettingsOperationDeserializer()
operationName = "GetAccountSettings"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Returns information about a specified resource group.
*
* **Minimum permissions**
*
* To run this command, you must have the following permissions:
* + `resource-groups:GetGroup`
*/
override suspend fun getGroup(input: GetGroupRequest): GetGroupResponse {
val op = SdkHttpOperation.build {
serializeWith = GetGroupOperationSerializer()
deserializeWith = GetGroupOperationDeserializer()
operationName = "GetGroup"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Retrieves the service configuration associated with the specified resource group. For details about the service configuration syntax, see [Service configurations for Resource Groups](https://docs.aws.amazon.com/ARG/latest/APIReference/about-slg.html).
*
* **Minimum permissions**
*
* To run this command, you must have the following permissions:
* + `resource-groups:GetGroupConfiguration`
*/
override suspend fun getGroupConfiguration(input: GetGroupConfigurationRequest): GetGroupConfigurationResponse {
val op = SdkHttpOperation.build {
serializeWith = GetGroupConfigurationOperationSerializer()
deserializeWith = GetGroupConfigurationOperationDeserializer()
operationName = "GetGroupConfiguration"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Retrieves the resource query associated with the specified resource group. For more information about resource queries, see [Create a tag-based group in Resource Groups](https://docs.aws.amazon.com/ARG/latest/userguide/gettingstarted-query.html#gettingstarted-query-cli-tag).
*
* **Minimum permissions**
*
* To run this command, you must have the following permissions:
* + `resource-groups:GetGroupQuery`
*/
override suspend fun getGroupQuery(input: GetGroupQueryRequest): GetGroupQueryResponse {
val op = SdkHttpOperation.build {
serializeWith = GetGroupQueryOperationSerializer()
deserializeWith = GetGroupQueryOperationDeserializer()
operationName = "GetGroupQuery"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Returns information about a specified tag-sync task.
*
* **Minimum permissions**
*
* To run this command, you must have the following permissions:
* + `resource-groups:GetTagSyncTask` on the application group
*/
override suspend fun getTagSyncTask(input: GetTagSyncTaskRequest): GetTagSyncTaskResponse {
val op = SdkHttpOperation.build {
serializeWith = GetTagSyncTaskOperationSerializer()
deserializeWith = GetTagSyncTaskOperationDeserializer()
operationName = "GetTagSyncTask"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Returns a list of tags that are associated with a resource group, specified by an Amazon resource name (ARN).
*
* **Minimum permissions**
*
* To run this command, you must have the following permissions:
* + `resource-groups:GetTags`
*/
override suspend fun getTags(input: GetTagsRequest): GetTagsResponse {
val op = SdkHttpOperation.build {
serializeWith = GetTagsOperationSerializer()
deserializeWith = GetTagsOperationDeserializer()
operationName = "GetTags"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Adds the specified resources to the specified group.
*
* You can only use this operation with the following groups:
* + `AWS::EC2::HostManagement`
* + `AWS::EC2::CapacityReservationPool`
* + `AWS::ResourceGroups::ApplicationGroup`
* Other resource group types and resource types are not currently supported by this operation.
*
* **Minimum permissions**
*
* To run this command, you must have the following permissions:
* + `resource-groups:GroupResources`
*/
override suspend fun groupResources(input: GroupResourcesRequest): GroupResourcesResponse {
val op = SdkHttpOperation.build {
serializeWith = GroupResourcesOperationSerializer()
deserializeWith = GroupResourcesOperationDeserializer()
operationName = "GroupResources"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Returns a list of Amazon resource names (ARNs) of the resources that are members of a specified resource group.
*
* **Minimum permissions**
*
* To run this command, you must have the following permissions:
* + `resource-groups:ListGroupResources`
* + `cloudformation:DescribeStacks`
* + `cloudformation:ListStackResources`
* + `tag:GetResources`
*/
override suspend fun listGroupResources(input: ListGroupResourcesRequest): ListGroupResourcesResponse {
val op = SdkHttpOperation.build {
serializeWith = ListGroupResourcesOperationSerializer()
deserializeWith = ListGroupResourcesOperationDeserializer()
operationName = "ListGroupResources"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Returns the status of the last grouping or ungrouping action for each resource in the specified application group.
*/
override suspend fun listGroupingStatuses(input: ListGroupingStatusesRequest): ListGroupingStatusesResponse {
val op = SdkHttpOperation.build {
serializeWith = ListGroupingStatusesOperationSerializer()
deserializeWith = ListGroupingStatusesOperationDeserializer()
operationName = "ListGroupingStatuses"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Returns a list of existing Resource Groups in your account.
*
* **Minimum permissions**
*
* To run this command, you must have the following permissions:
* + `resource-groups:ListGroups`
*/
override suspend fun listGroups(input: ListGroupsRequest): ListGroupsResponse {
val op = SdkHttpOperation.build {
serializeWith = ListGroupsOperationSerializer()
deserializeWith = ListGroupsOperationDeserializer()
operationName = "ListGroups"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Returns a list of tag-sync tasks.
*
* **Minimum permissions**
*
* To run this command, you must have the following permissions:
* + `resource-groups:ListTagSyncTasks` with the group passed in the filters as the resource or * if using no filters
*/
override suspend fun listTagSyncTasks(input: ListTagSyncTasksRequest): ListTagSyncTasksResponse {
val op = SdkHttpOperation.build {
serializeWith = ListTagSyncTasksOperationSerializer()
deserializeWith = ListTagSyncTasksOperationDeserializer()
operationName = "ListTagSyncTasks"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Attaches a service configuration to the specified group. This occurs asynchronously, and can take time to complete. You can use GetGroupConfiguration to check the status of the update.
*
* **Minimum permissions**
*
* To run this command, you must have the following permissions:
* + `resource-groups:PutGroupConfiguration`
*/
override suspend fun putGroupConfiguration(input: PutGroupConfigurationRequest): PutGroupConfigurationResponse {
val op = SdkHttpOperation.build {
serializeWith = PutGroupConfigurationOperationSerializer()
deserializeWith = PutGroupConfigurationOperationDeserializer()
operationName = "PutGroupConfiguration"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Returns a list of Amazon Web Services resource identifiers that matches the specified query. The query uses the same format as a resource query in a CreateGroup or UpdateGroupQuery operation.
*
* **Minimum permissions**
*
* To run this command, you must have the following permissions:
* + `resource-groups:SearchResources`
* + `cloudformation:DescribeStacks`
* + `cloudformation:ListStackResources`
* + `tag:GetResources`
*/
override suspend fun searchResources(input: SearchResourcesRequest): SearchResourcesResponse {
val op = SdkHttpOperation.build {
serializeWith = SearchResourcesOperationSerializer()
deserializeWith = SearchResourcesOperationDeserializer()
operationName = "SearchResources"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Creates a new tag-sync task to onboard and sync resources tagged with a specific tag key-value pair to an application.
*
* **Minimum permissions**
*
* To run this command, you must have the following permissions:
* + `resource-groups:StartTagSyncTask` on the application group
* + `resource-groups:CreateGroup`
* + `iam:PassRole` on the role provided in the request
*/
override suspend fun startTagSyncTask(input: StartTagSyncTaskRequest): StartTagSyncTaskResponse {
val op = SdkHttpOperation.build {
serializeWith = StartTagSyncTaskOperationSerializer()
deserializeWith = StartTagSyncTaskOperationDeserializer()
operationName = "StartTagSyncTask"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Adds tags to a resource group with the specified Amazon resource name (ARN). Existing tags on a resource group are not changed if they are not specified in the request parameters.
*
* Do not store personally identifiable information (PII) or other confidential or sensitive information in tags. We use tags to provide you with billing and administration services. Tags are not intended to be used for private or sensitive data.
*
* **Minimum permissions**
*
* To run this command, you must have the following permissions:
* + `resource-groups:Tag`
*/
override suspend fun tag(input: TagRequest): TagResponse {
val op = SdkHttpOperation.build {
serializeWith = TagOperationSerializer()
deserializeWith = TagOperationDeserializer()
operationName = "Tag"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Removes the specified resources from the specified group. This operation works only with static groups that you populated using the GroupResources operation. It doesn't work with any resource groups that are automatically populated by tag-based or CloudFormation stack-based queries.
*
* **Minimum permissions**
*
* To run this command, you must have the following permissions:
* + `resource-groups:UngroupResources`
*/
override suspend fun ungroupResources(input: UngroupResourcesRequest): UngroupResourcesResponse {
val op = SdkHttpOperation.build {
serializeWith = UngroupResourcesOperationSerializer()
deserializeWith = UngroupResourcesOperationDeserializer()
operationName = "UngroupResources"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Deletes tags from a specified resource group.
*
* **Minimum permissions**
*
* To run this command, you must have the following permissions:
* + `resource-groups:Untag`
*/
override suspend fun untag(input: UntagRequest): UntagResponse {
val op = SdkHttpOperation.build {
serializeWith = UntagOperationSerializer()
deserializeWith = UntagOperationDeserializer()
operationName = "Untag"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Turns on or turns off optional features in Resource Groups.
*
* The preceding example shows that the request to turn on group lifecycle events is `IN_PROGRESS`. You can call the GetAccountSettings operation to check for completion by looking for `GroupLifecycleEventsStatus` to change to `ACTIVE`.
*/
override suspend fun updateAccountSettings(input: UpdateAccountSettingsRequest): UpdateAccountSettingsResponse {
val op = SdkHttpOperation.build {
serializeWith = UpdateAccountSettingsOperationSerializer()
deserializeWith = UpdateAccountSettingsOperationDeserializer()
operationName = "UpdateAccountSettings"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Updates the description for an existing group. You cannot update the name of a resource group.
*
* **Minimum permissions**
*
* To run this command, you must have the following permissions:
* + `resource-groups:UpdateGroup`
*/
override suspend fun updateGroup(input: UpdateGroupRequest): UpdateGroupResponse {
val op = SdkHttpOperation.build {
serializeWith = UpdateGroupOperationSerializer()
deserializeWith = UpdateGroupOperationDeserializer()
operationName = "UpdateGroup"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Updates the resource query of a group. For more information about resource queries, see [Create a tag-based group in Resource Groups](https://docs.aws.amazon.com/ARG/latest/userguide/gettingstarted-query.html#gettingstarted-query-cli-tag).
*
* **Minimum permissions**
*
* To run this command, you must have the following permissions:
* + `resource-groups:UpdateGroupQuery`
*/
override suspend fun updateGroupQuery(input: UpdateGroupQueryRequest): UpdateGroupQueryResponse {
val op = SdkHttpOperation.build {
serializeWith = UpdateGroupQueryOperationSerializer()
deserializeWith = UpdateGroupQueryOperationDeserializer()
operationName = "UpdateGroupQuery"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
override fun close() {
managedResources.unshareAll()
}
/**
* merge the defaults configured for the service into the execution context before firing off a request
*/
private fun mergeServiceDefaults(ctx: ExecutionContext) {
ctx.putIfAbsent(SdkClientOption.ClientName, config.clientName)
ctx.putIfAbsent(SdkClientOption.LogMode, config.logMode)
ctx.putIfAbsentNotNull(AwsAttributes.Region, config.region)
ctx.putIfAbsentNotNull(AwsSigningAttributes.SigningRegion, config.region)
ctx.putIfAbsent(AwsSigningAttributes.SigningService, "resource-groups")
ctx.putIfAbsent(AwsSigningAttributes.CredentialsProvider, config.credentialsProvider)
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy