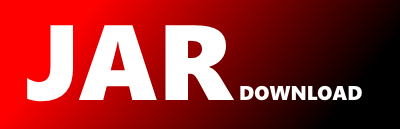
commonMain.aws.sdk.kotlin.services.resourcegroups.model.Group.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of resourcegroups-jvm Show documentation
Show all versions of resourcegroups-jvm Show documentation
The AWS SDK for Kotlin client for Resource Groups
The newest version!
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.resourcegroups.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* A resource group that contains Amazon Web Services resources. You can assign resources to the group by associating either of the following elements with the group:
* + ResourceQuery - Use a resource query to specify a set of tag keys and values. All resources in the same Amazon Web Services Region and Amazon Web Services account that have those keys with the same values are included in the group. You can add a resource query when you create the group, or later by using the PutGroupConfiguration operation.
* + GroupConfiguration - Use a service configuration to associate the group with an Amazon Web Services service. The configuration specifies which resource types can be included in the group.
*/
public class Group private constructor(builder: Builder) {
/**
* A tag that defines the application group membership. This tag is only supported for application groups.
*/
public val applicationTag: Map? = builder.applicationTag
/**
* The critical rank of the application group on a scale of 1 to 10, with a rank of 1 being the most critical, and a rank of 10 being least critical.
*/
public val criticality: kotlin.Int? = builder.criticality
/**
* The description of the resource group.
*/
public val description: kotlin.String? = builder.description
/**
* The name of the application group, which you can change at any time.
*/
public val displayName: kotlin.String? = builder.displayName
/**
* The Amazon resource name (ARN) of the resource group.
*/
public val groupArn: kotlin.String = requireNotNull(builder.groupArn) { "A non-null value must be provided for groupArn" }
/**
* The name of the resource group.
*/
public val name: kotlin.String = requireNotNull(builder.name) { "A non-null value must be provided for name" }
/**
* A name, email address or other identifier for the person or group who is considered as the owner of this application group within your organization.
*/
public val owner: kotlin.String? = builder.owner
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.resourcegroups.model.Group = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("Group(")
append("applicationTag=$applicationTag,")
append("criticality=$criticality,")
append("description=$description,")
append("displayName=$displayName,")
append("groupArn=$groupArn,")
append("name=$name,")
append("owner=$owner")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = applicationTag?.hashCode() ?: 0
result = 31 * result + (criticality ?: 0)
result = 31 * result + (description?.hashCode() ?: 0)
result = 31 * result + (displayName?.hashCode() ?: 0)
result = 31 * result + (groupArn.hashCode())
result = 31 * result + (name.hashCode())
result = 31 * result + (owner?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as Group
if (applicationTag != other.applicationTag) return false
if (criticality != other.criticality) return false
if (description != other.description) return false
if (displayName != other.displayName) return false
if (groupArn != other.groupArn) return false
if (name != other.name) return false
if (owner != other.owner) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.resourcegroups.model.Group = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* A tag that defines the application group membership. This tag is only supported for application groups.
*/
public var applicationTag: Map? = null
/**
* The critical rank of the application group on a scale of 1 to 10, with a rank of 1 being the most critical, and a rank of 10 being least critical.
*/
public var criticality: kotlin.Int? = null
/**
* The description of the resource group.
*/
public var description: kotlin.String? = null
/**
* The name of the application group, which you can change at any time.
*/
public var displayName: kotlin.String? = null
/**
* The Amazon resource name (ARN) of the resource group.
*/
public var groupArn: kotlin.String? = null
/**
* The name of the resource group.
*/
public var name: kotlin.String? = null
/**
* A name, email address or other identifier for the person or group who is considered as the owner of this application group within your organization.
*/
public var owner: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.resourcegroups.model.Group) : this() {
this.applicationTag = x.applicationTag
this.criticality = x.criticality
this.description = x.description
this.displayName = x.displayName
this.groupArn = x.groupArn
this.name = x.name
this.owner = x.owner
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.resourcegroups.model.Group = Group(this)
internal fun correctErrors(): Builder {
if (groupArn == null) groupArn = ""
if (name == null) name = ""
return this
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy