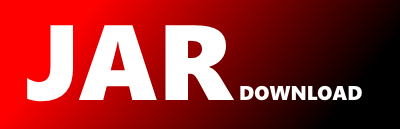
commonMain.aws.sdk.kotlin.services.route53recoverycontrolconfig.model.Cluster.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of route53recoverycontrolconfig-jvm Show documentation
Show all versions of route53recoverycontrolconfig-jvm Show documentation
The AWS Kotlin client for Route53 Recovery Control Config
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.route53recoverycontrolconfig.model
/**
* A set of five redundant Regional endpoints against which you can execute API calls to update or get the state of routing controls. You can host multiple control panels and routing controls on one cluster.
*/
public class Cluster private constructor(builder: Builder) {
/**
* The Amazon Resource Name (ARN) of the cluster.
*/
public val clusterArn: kotlin.String? = builder.clusterArn
/**
* Endpoints for a cluster. Specify one of these endpoints when you want to set or retrieve a routing control state in the cluster.
*
* To get or update the routing control state, see the Amazon Route 53 Application Recovery Controller Routing Control Actions.
*/
public val clusterEndpoints: List? = builder.clusterEndpoints
/**
* The name of the cluster.
*/
public val name: kotlin.String? = builder.name
/**
* The Amazon Web Services account ID of the cluster owner.
*/
public val owner: kotlin.String? = builder.owner
/**
* Deployment status of a resource. Status can be one of the following: PENDING, DEPLOYED, PENDING_DELETION.
*/
public val status: aws.sdk.kotlin.services.route53recoverycontrolconfig.model.Status? = builder.status
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.route53recoverycontrolconfig.model.Cluster = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("Cluster(")
append("clusterArn=$clusterArn,")
append("clusterEndpoints=$clusterEndpoints,")
append("name=$name,")
append("owner=$owner,")
append("status=$status")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = clusterArn?.hashCode() ?: 0
result = 31 * result + (clusterEndpoints?.hashCode() ?: 0)
result = 31 * result + (name?.hashCode() ?: 0)
result = 31 * result + (owner?.hashCode() ?: 0)
result = 31 * result + (status?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as Cluster
if (clusterArn != other.clusterArn) return false
if (clusterEndpoints != other.clusterEndpoints) return false
if (name != other.name) return false
if (owner != other.owner) return false
if (status != other.status) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.route53recoverycontrolconfig.model.Cluster = Builder(this).apply(block).build()
public class Builder {
/**
* The Amazon Resource Name (ARN) of the cluster.
*/
public var clusterArn: kotlin.String? = null
/**
* Endpoints for a cluster. Specify one of these endpoints when you want to set or retrieve a routing control state in the cluster.
*
* To get or update the routing control state, see the Amazon Route 53 Application Recovery Controller Routing Control Actions.
*/
public var clusterEndpoints: List? = null
/**
* The name of the cluster.
*/
public var name: kotlin.String? = null
/**
* The Amazon Web Services account ID of the cluster owner.
*/
public var owner: kotlin.String? = null
/**
* Deployment status of a resource. Status can be one of the following: PENDING, DEPLOYED, PENDING_DELETION.
*/
public var status: aws.sdk.kotlin.services.route53recoverycontrolconfig.model.Status? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.route53recoverycontrolconfig.model.Cluster) : this() {
this.clusterArn = x.clusterArn
this.clusterEndpoints = x.clusterEndpoints
this.name = x.name
this.owner = x.owner
this.status = x.status
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.route53recoverycontrolconfig.model.Cluster = Cluster(this)
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy