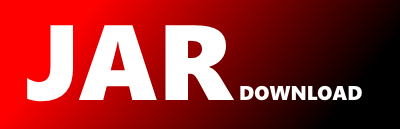
commonMain.aws.sdk.kotlin.services.route53recoverycontrolconfig.waiters.Waiters.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of route53recoverycontrolconfig-jvm Show documentation
Show all versions of route53recoverycontrolconfig-jvm Show documentation
The AWS Kotlin client for Route53 Recovery Control Config
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.route53recoverycontrolconfig.waiters
import aws.sdk.kotlin.services.route53recoverycontrolconfig.Route53RecoveryControlConfigClient
import aws.sdk.kotlin.services.route53recoverycontrolconfig.model.DescribeClusterRequest
import aws.sdk.kotlin.services.route53recoverycontrolconfig.model.DescribeClusterResponse
import aws.sdk.kotlin.services.route53recoverycontrolconfig.model.DescribeControlPanelRequest
import aws.sdk.kotlin.services.route53recoverycontrolconfig.model.DescribeControlPanelResponse
import aws.sdk.kotlin.services.route53recoverycontrolconfig.model.DescribeRoutingControlRequest
import aws.sdk.kotlin.services.route53recoverycontrolconfig.model.DescribeRoutingControlResponse
import aws.smithy.kotlin.runtime.retries.Outcome
import aws.smithy.kotlin.runtime.retries.StandardRetryStrategy
import aws.smithy.kotlin.runtime.retries.delay.InfiniteTokenBucket
import aws.smithy.kotlin.runtime.retries.policy.Acceptor
import aws.smithy.kotlin.runtime.retries.policy.AcceptorRetryPolicy
import aws.smithy.kotlin.runtime.retries.policy.ErrorTypeAcceptor
import aws.smithy.kotlin.runtime.retries.policy.OutputAcceptor
import aws.smithy.kotlin.runtime.retries.policy.RetryDirective
import aws.smithy.kotlin.runtime.retries.policy.RetryErrorType
import kotlin.time.Duration.Companion.milliseconds
/**
* Wait until a cluster is created
*/
public suspend fun Route53RecoveryControlConfigClient.waitUntilClusterCreated(request: DescribeClusterRequest): Outcome {
val strategy = StandardRetryStrategy {
maxAttempts = 20
tokenBucket = InfiniteTokenBucket
delayProvider {
initialDelay = 5_000.milliseconds
scaleFactor = 1.5
jitter = 1.0
maxBackoff = 120_000.milliseconds
}
}
val acceptors = listOf>(
OutputAcceptor(RetryDirective.TerminateAndSucceed) {
val cluster = it.cluster
val status = cluster?.status?.value
status == "DEPLOYED"
},
OutputAcceptor(RetryDirective.RetryError(RetryErrorType.ServerSide)) {
val cluster = it.cluster
val status = cluster?.status?.value
status == "PENDING"
},
ErrorTypeAcceptor(RetryDirective.RetryError(RetryErrorType.ServerSide), "InternalServerException"),
)
val policy = AcceptorRetryPolicy(request, acceptors)
return strategy.retry(policy) { describeCluster(request) }
}
/**
* Wait until a cluster is created
*/
public suspend fun Route53RecoveryControlConfigClient.waitUntilClusterCreated(block: DescribeClusterRequest.Builder.() -> Unit): Outcome =
waitUntilClusterCreated(DescribeClusterRequest.Builder().apply(block).build())
/**
* Wait for a cluster to be deleted
*/
public suspend fun Route53RecoveryControlConfigClient.waitUntilClusterDeleted(request: DescribeClusterRequest): Outcome {
val strategy = StandardRetryStrategy {
maxAttempts = 20
tokenBucket = InfiniteTokenBucket
delayProvider {
initialDelay = 5_000.milliseconds
scaleFactor = 1.5
jitter = 1.0
maxBackoff = 120_000.milliseconds
}
}
val acceptors = listOf>(
ErrorTypeAcceptor(RetryDirective.TerminateAndSucceed, "ResourceNotFoundException"),
OutputAcceptor(RetryDirective.RetryError(RetryErrorType.ServerSide)) {
val cluster = it.cluster
val status = cluster?.status?.value
status == "PENDING_DELETION"
},
ErrorTypeAcceptor(RetryDirective.RetryError(RetryErrorType.ServerSide), "InternalServerException"),
)
val policy = AcceptorRetryPolicy(request, acceptors)
return strategy.retry(policy) { describeCluster(request) }
}
/**
* Wait for a cluster to be deleted
*/
public suspend fun Route53RecoveryControlConfigClient.waitUntilClusterDeleted(block: DescribeClusterRequest.Builder.() -> Unit): Outcome =
waitUntilClusterDeleted(DescribeClusterRequest.Builder().apply(block).build())
/**
* Wait until a control panel is created
*/
public suspend fun Route53RecoveryControlConfigClient.waitUntilControlPanelCreated(request: DescribeControlPanelRequest): Outcome {
val strategy = StandardRetryStrategy {
maxAttempts = 20
tokenBucket = InfiniteTokenBucket
delayProvider {
initialDelay = 5_000.milliseconds
scaleFactor = 1.5
jitter = 1.0
maxBackoff = 120_000.milliseconds
}
}
val acceptors = listOf>(
OutputAcceptor(RetryDirective.TerminateAndSucceed) {
val controlPanel = it.controlPanel
val status = controlPanel?.status?.value
status == "DEPLOYED"
},
OutputAcceptor(RetryDirective.RetryError(RetryErrorType.ServerSide)) {
val controlPanel = it.controlPanel
val status = controlPanel?.status?.value
status == "PENDING"
},
ErrorTypeAcceptor(RetryDirective.RetryError(RetryErrorType.ServerSide), "InternalServerException"),
)
val policy = AcceptorRetryPolicy(request, acceptors)
return strategy.retry(policy) { describeControlPanel(request) }
}
/**
* Wait until a control panel is created
*/
public suspend fun Route53RecoveryControlConfigClient.waitUntilControlPanelCreated(block: DescribeControlPanelRequest.Builder.() -> Unit): Outcome =
waitUntilControlPanelCreated(DescribeControlPanelRequest.Builder().apply(block).build())
/**
* Wait until a control panel is deleted
*/
public suspend fun Route53RecoveryControlConfigClient.waitUntilControlPanelDeleted(request: DescribeControlPanelRequest): Outcome {
val strategy = StandardRetryStrategy {
maxAttempts = 20
tokenBucket = InfiniteTokenBucket
delayProvider {
initialDelay = 5_000.milliseconds
scaleFactor = 1.5
jitter = 1.0
maxBackoff = 120_000.milliseconds
}
}
val acceptors = listOf>(
ErrorTypeAcceptor(RetryDirective.TerminateAndSucceed, "ResourceNotFoundException"),
OutputAcceptor(RetryDirective.RetryError(RetryErrorType.ServerSide)) {
val controlPanel = it.controlPanel
val status = controlPanel?.status?.value
status == "PENDING_DELETION"
},
ErrorTypeAcceptor(RetryDirective.RetryError(RetryErrorType.ServerSide), "InternalServerException"),
)
val policy = AcceptorRetryPolicy(request, acceptors)
return strategy.retry(policy) { describeControlPanel(request) }
}
/**
* Wait until a control panel is deleted
*/
public suspend fun Route53RecoveryControlConfigClient.waitUntilControlPanelDeleted(block: DescribeControlPanelRequest.Builder.() -> Unit): Outcome =
waitUntilControlPanelDeleted(DescribeControlPanelRequest.Builder().apply(block).build())
/**
* Wait until a routing control is created
*/
public suspend fun Route53RecoveryControlConfigClient.waitUntilRoutingControlCreated(request: DescribeRoutingControlRequest): Outcome {
val strategy = StandardRetryStrategy {
maxAttempts = 20
tokenBucket = InfiniteTokenBucket
delayProvider {
initialDelay = 5_000.milliseconds
scaleFactor = 1.5
jitter = 1.0
maxBackoff = 120_000.milliseconds
}
}
val acceptors = listOf>(
OutputAcceptor(RetryDirective.TerminateAndSucceed) {
val routingControl = it.routingControl
val status = routingControl?.status?.value
status == "DEPLOYED"
},
OutputAcceptor(RetryDirective.RetryError(RetryErrorType.ServerSide)) {
val routingControl = it.routingControl
val status = routingControl?.status?.value
status == "PENDING"
},
ErrorTypeAcceptor(RetryDirective.RetryError(RetryErrorType.ServerSide), "InternalServerException"),
)
val policy = AcceptorRetryPolicy(request, acceptors)
return strategy.retry(policy) { describeRoutingControl(request) }
}
/**
* Wait until a routing control is created
*/
public suspend fun Route53RecoveryControlConfigClient.waitUntilRoutingControlCreated(block: DescribeRoutingControlRequest.Builder.() -> Unit): Outcome =
waitUntilRoutingControlCreated(DescribeRoutingControlRequest.Builder().apply(block).build())
/**
* Wait for a routing control to be deleted
*/
public suspend fun Route53RecoveryControlConfigClient.waitUntilRoutingControlDeleted(request: DescribeRoutingControlRequest): Outcome {
val strategy = StandardRetryStrategy {
maxAttempts = 20
tokenBucket = InfiniteTokenBucket
delayProvider {
initialDelay = 5_000.milliseconds
scaleFactor = 1.5
jitter = 1.0
maxBackoff = 120_000.milliseconds
}
}
val acceptors = listOf>(
ErrorTypeAcceptor(RetryDirective.TerminateAndSucceed, "ResourceNotFoundException"),
OutputAcceptor(RetryDirective.RetryError(RetryErrorType.ServerSide)) {
val routingControl = it.routingControl
val status = routingControl?.status?.value
status == "PENDING_DELETION"
},
ErrorTypeAcceptor(RetryDirective.RetryError(RetryErrorType.ServerSide), "InternalServerException"),
)
val policy = AcceptorRetryPolicy(request, acceptors)
return strategy.retry(policy) { describeRoutingControl(request) }
}
/**
* Wait for a routing control to be deleted
*/
public suspend fun Route53RecoveryControlConfigClient.waitUntilRoutingControlDeleted(block: DescribeRoutingControlRequest.Builder.() -> Unit): Outcome =
waitUntilRoutingControlDeleted(DescribeRoutingControlRequest.Builder().apply(block).build())
© 2015 - 2025 Weber Informatics LLC | Privacy Policy