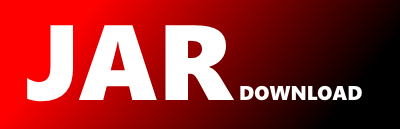
commonMain.aws.sdk.kotlin.services.s3outposts.S3OutpostsClient.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of s3outposts-jvm Show documentation
Show all versions of s3outposts-jvm Show documentation
The AWS SDK for Kotlin client for S3Outposts
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.s3outposts
import aws.sdk.kotlin.runtime.auth.credentials.DefaultChainCredentialsProvider
import aws.sdk.kotlin.runtime.auth.credentials.internal.borrow
import aws.sdk.kotlin.runtime.endpoint.AwsEndpointResolver
import aws.sdk.kotlin.runtime.region.resolveRegion
import aws.sdk.kotlin.services.s3outposts.internal.DefaultEndpointResolver
import aws.sdk.kotlin.services.s3outposts.model.*
import aws.smithy.kotlin.runtime.SdkClient
import aws.smithy.kotlin.runtime.auth.awscredentials.CredentialsProvider
import aws.smithy.kotlin.runtime.auth.awssigning.AwsSigner
import aws.smithy.kotlin.runtime.auth.awssigning.crt.CrtAwsSigner
import aws.smithy.kotlin.runtime.client.SdkLogMode
import aws.smithy.kotlin.runtime.config.SdkClientConfig
import aws.smithy.kotlin.runtime.http.config.HttpClientConfig
import aws.smithy.kotlin.runtime.http.endpoints.EndpointResolver
import aws.smithy.kotlin.runtime.http.engine.HttpClientEngine
import aws.smithy.kotlin.runtime.retries.RetryStrategy
import aws.smithy.kotlin.runtime.retries.StandardRetryStrategy
/**
* Amazon S3 on Outposts provides access to S3 on Outposts operations.
*/
interface S3OutpostsClient : SdkClient {
override val serviceName: String
get() = "S3Outposts"
/**
* S3OutpostsClient's configuration
*/
val config: Config
companion object {
operator fun invoke(block: Config.Builder.() -> Unit): S3OutpostsClient {
val config = Config.Builder().apply(block).build()
return DefaultS3OutpostsClient(config)
}
operator fun invoke(config: Config): S3OutpostsClient = DefaultS3OutpostsClient(config)
/**
* Construct a [S3OutpostsClient] by resolving the configuration from the current environment.
*/
suspend fun fromEnvironment(block: (Config.Builder.() -> Unit)? = null): S3OutpostsClient {
val builder = Config.Builder()
if (block != null) builder.apply(block)
builder.region = builder.region ?: resolveRegion()
return DefaultS3OutpostsClient(builder.build())
}
}
class Config private constructor(builder: Builder): HttpClientConfig, SdkClientConfig {
val credentialsProvider: CredentialsProvider = builder.credentialsProvider?.borrow() ?: DefaultChainCredentialsProvider()
val endpointResolver: AwsEndpointResolver = builder.endpointResolver ?: DefaultEndpointResolver()
override val httpClientEngine: HttpClientEngine? = builder.httpClientEngine
val region: String = requireNotNull(builder.region) { "region is a required configuration property" }
val retryStrategy: RetryStrategy = StandardRetryStrategy()
override val sdkLogMode: SdkLogMode = builder.sdkLogMode
val signer: AwsSigner = builder.signer ?: CrtAwsSigner
companion object {
inline operator fun invoke(block: Builder.() -> kotlin.Unit): Config = Builder().apply(block).build()
}
class Builder {
/**
* The AWS credentials provider to use for authenticating requests. If not provided a
* [aws.sdk.kotlin.runtime.auth.credentials.DefaultChainCredentialsProvider] instance will be used.
* NOTE: The caller is responsible for managing the lifetime of the provider when set. The SDK
* client will not close it when the client is closed.
*/
var credentialsProvider: CredentialsProvider? = null
/**
* Determines the endpoint (hostname) to make requests to. When not provided a default
* resolver is configured automatically. This is an advanced client option.
*/
var endpointResolver: AwsEndpointResolver? = null
/**
* Override the default HTTP client engine used to make SDK requests (e.g. configure proxy behavior, timeouts, concurrency, etc).
* NOTE: The caller is responsible for managing the lifetime of the engine when set. The SDK
* client will not close it when the client is closed.
*/
var httpClientEngine: HttpClientEngine? = null
/**
* AWS region to make requests to
*/
var region: String? = null
/**
* Configure events that will be logged. By default clients will not output
* raw requests or responses. Use this setting to opt-in to additional debug logging.
*
* This can be used to configure logging of requests, responses, retries, etc of SDK clients.
*
* **NOTE**: Logging of raw requests or responses may leak sensitive information! It may also have
* performance considerations when dumping the request/response body. This is primarily a tool for
* debug purposes.
*/
var sdkLogMode: SdkLogMode = SdkLogMode.Default
/**
* The implementation of AWS signer to use for signing requests
*/
var signer: AwsSigner? = null
@PublishedApi
internal fun build(): Config = Config(this)
}
}
/**
* Creates an endpoint and associates it with the specified Outpost.
*
* It can take up to 5 minutes for this action to finish.
*
* Related actions include:
* + [DeleteEndpoint](https://docs.aws.amazon.com/AmazonS3/latest/API/API_s3outposts_DeleteEndpoint.html)
* + [ListEndpoints](https://docs.aws.amazon.com/AmazonS3/latest/API/API_s3outposts_ListEndpoints.html)
*/
suspend fun createEndpoint(input: CreateEndpointRequest): CreateEndpointResponse
/**
* Creates an endpoint and associates it with the specified Outpost.
*
* It can take up to 5 minutes for this action to finish.
*
* Related actions include:
* + [DeleteEndpoint](https://docs.aws.amazon.com/AmazonS3/latest/API/API_s3outposts_DeleteEndpoint.html)
* + [ListEndpoints](https://docs.aws.amazon.com/AmazonS3/latest/API/API_s3outposts_ListEndpoints.html)
*/
suspend fun createEndpoint(block: CreateEndpointRequest.Builder.() -> Unit) = createEndpoint(CreateEndpointRequest.Builder().apply(block).build())
/**
* Deletes an endpoint.
*
* It can take up to 5 minutes for this action to finish.
*
* Related actions include:
* + [CreateEndpoint](https://docs.aws.amazon.com/AmazonS3/latest/API/API_s3outposts_CreateEndpoint.html)
* + [ListEndpoints](https://docs.aws.amazon.com/AmazonS3/latest/API/API_s3outposts_ListEndpoints.html)
*/
suspend fun deleteEndpoint(input: DeleteEndpointRequest): DeleteEndpointResponse
/**
* Deletes an endpoint.
*
* It can take up to 5 minutes for this action to finish.
*
* Related actions include:
* + [CreateEndpoint](https://docs.aws.amazon.com/AmazonS3/latest/API/API_s3outposts_CreateEndpoint.html)
* + [ListEndpoints](https://docs.aws.amazon.com/AmazonS3/latest/API/API_s3outposts_ListEndpoints.html)
*/
suspend fun deleteEndpoint(block: DeleteEndpointRequest.Builder.() -> Unit) = deleteEndpoint(DeleteEndpointRequest.Builder().apply(block).build())
/**
* Lists endpoints associated with the specified Outpost.
*
* Related actions include:
* + [CreateEndpoint](https://docs.aws.amazon.com/AmazonS3/latest/API/API_s3outposts_CreateEndpoint.html)
* + [DeleteEndpoint](https://docs.aws.amazon.com/AmazonS3/latest/API/API_s3outposts_DeleteEndpoint.html)
*/
suspend fun listEndpoints(input: ListEndpointsRequest = ListEndpointsRequest {}): ListEndpointsResponse
/**
* Lists endpoints associated with the specified Outpost.
*
* Related actions include:
* + [CreateEndpoint](https://docs.aws.amazon.com/AmazonS3/latest/API/API_s3outposts_CreateEndpoint.html)
* + [DeleteEndpoint](https://docs.aws.amazon.com/AmazonS3/latest/API/API_s3outposts_DeleteEndpoint.html)
*/
suspend fun listEndpoints(block: ListEndpointsRequest.Builder.() -> Unit) = listEndpoints(ListEndpointsRequest.Builder().apply(block).build())
/**
* Lists all endpoints associated with an Outpost that has been shared by Amazon Web Services Resource Access Manager (RAM).
*
* Related actions include:
* + [CreateEndpoint](https://docs.aws.amazon.com/AmazonS3/latest/API/API_s3outposts_CreateEndpoint.html)
* + [DeleteEndpoint](https://docs.aws.amazon.com/AmazonS3/latest/API/API_s3outposts_DeleteEndpoint.html)
*/
suspend fun listSharedEndpoints(input: ListSharedEndpointsRequest): ListSharedEndpointsResponse
/**
* Lists all endpoints associated with an Outpost that has been shared by Amazon Web Services Resource Access Manager (RAM).
*
* Related actions include:
* + [CreateEndpoint](https://docs.aws.amazon.com/AmazonS3/latest/API/API_s3outposts_CreateEndpoint.html)
* + [DeleteEndpoint](https://docs.aws.amazon.com/AmazonS3/latest/API/API_s3outposts_DeleteEndpoint.html)
*/
suspend fun listSharedEndpoints(block: ListSharedEndpointsRequest.Builder.() -> Unit) = listSharedEndpoints(ListSharedEndpointsRequest.Builder().apply(block).build())
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy