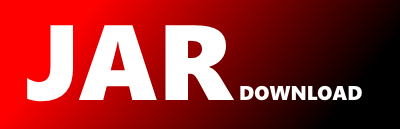
commonMain.aws.sdk.kotlin.services.s3outposts.model.Endpoint.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.s3outposts.model
import aws.smithy.kotlin.runtime.time.Instant
/**
* Amazon S3 on Outposts Access Points simplify managing data access at scale for shared datasets in S3 on Outposts. S3 on Outposts uses endpoints to connect to Outposts buckets so that you can perform actions within your virtual private cloud (VPC). For more information, see [ Accessing S3 on Outposts using VPC-only access points](https://docs.aws.amazon.com/AmazonS3/latest/userguide/WorkingWithS3Outposts.html) in the *Amazon Simple Storage Service User Guide*.
*/
class Endpoint private constructor(builder: Builder) {
/**
* The type of connectivity used to access the Amazon S3 on Outposts endpoint.
*/
val accessType: aws.sdk.kotlin.services.s3outposts.model.EndpointAccessType? = builder.accessType
/**
* The VPC CIDR committed by this endpoint.
*/
val cidrBlock: kotlin.String? = builder.cidrBlock
/**
* The time the endpoint was created.
*/
val creationTime: aws.smithy.kotlin.runtime.time.Instant? = builder.creationTime
/**
* The ID of the customer-owned IPv4 address pool used for the endpoint.
*/
val customerOwnedIpv4Pool: kotlin.String? = builder.customerOwnedIpv4Pool
/**
* The Amazon Resource Name (ARN) of the endpoint.
*/
val endpointArn: kotlin.String? = builder.endpointArn
/**
* The network interface of the endpoint.
*/
val networkInterfaces: List? = builder.networkInterfaces
/**
* The ID of the Outposts.
*/
val outpostsId: kotlin.String? = builder.outpostsId
/**
* The ID of the security group used for the endpoint.
*/
val securityGroupId: kotlin.String? = builder.securityGroupId
/**
* The status of the endpoint.
*/
val status: aws.sdk.kotlin.services.s3outposts.model.EndpointStatus? = builder.status
/**
* The ID of the subnet used for the endpoint.
*/
val subnetId: kotlin.String? = builder.subnetId
/**
* The ID of the VPC used for the endpoint.
*/
val vpcId: kotlin.String? = builder.vpcId
companion object {
operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.s3outposts.model.Endpoint = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("Endpoint(")
append("accessType=$accessType,")
append("cidrBlock=$cidrBlock,")
append("creationTime=$creationTime,")
append("customerOwnedIpv4Pool=$customerOwnedIpv4Pool,")
append("endpointArn=$endpointArn,")
append("networkInterfaces=$networkInterfaces,")
append("outpostsId=$outpostsId,")
append("securityGroupId=$securityGroupId,")
append("status=$status,")
append("subnetId=$subnetId,")
append("vpcId=$vpcId)")
}
override fun hashCode(): kotlin.Int {
var result = accessType?.hashCode() ?: 0
result = 31 * result + (cidrBlock?.hashCode() ?: 0)
result = 31 * result + (creationTime?.hashCode() ?: 0)
result = 31 * result + (customerOwnedIpv4Pool?.hashCode() ?: 0)
result = 31 * result + (endpointArn?.hashCode() ?: 0)
result = 31 * result + (networkInterfaces?.hashCode() ?: 0)
result = 31 * result + (outpostsId?.hashCode() ?: 0)
result = 31 * result + (securityGroupId?.hashCode() ?: 0)
result = 31 * result + (status?.hashCode() ?: 0)
result = 31 * result + (subnetId?.hashCode() ?: 0)
result = 31 * result + (vpcId?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as Endpoint
if (accessType != other.accessType) return false
if (cidrBlock != other.cidrBlock) return false
if (creationTime != other.creationTime) return false
if (customerOwnedIpv4Pool != other.customerOwnedIpv4Pool) return false
if (endpointArn != other.endpointArn) return false
if (networkInterfaces != other.networkInterfaces) return false
if (outpostsId != other.outpostsId) return false
if (securityGroupId != other.securityGroupId) return false
if (status != other.status) return false
if (subnetId != other.subnetId) return false
if (vpcId != other.vpcId) return false
return true
}
inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.s3outposts.model.Endpoint = Builder(this).apply(block).build()
class Builder {
/**
* The type of connectivity used to access the Amazon S3 on Outposts endpoint.
*/
var accessType: aws.sdk.kotlin.services.s3outposts.model.EndpointAccessType? = null
/**
* The VPC CIDR committed by this endpoint.
*/
var cidrBlock: kotlin.String? = null
/**
* The time the endpoint was created.
*/
var creationTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The ID of the customer-owned IPv4 address pool used for the endpoint.
*/
var customerOwnedIpv4Pool: kotlin.String? = null
/**
* The Amazon Resource Name (ARN) of the endpoint.
*/
var endpointArn: kotlin.String? = null
/**
* The network interface of the endpoint.
*/
var networkInterfaces: List? = null
/**
* The ID of the Outposts.
*/
var outpostsId: kotlin.String? = null
/**
* The ID of the security group used for the endpoint.
*/
var securityGroupId: kotlin.String? = null
/**
* The status of the endpoint.
*/
var status: aws.sdk.kotlin.services.s3outposts.model.EndpointStatus? = null
/**
* The ID of the subnet used for the endpoint.
*/
var subnetId: kotlin.String? = null
/**
* The ID of the VPC used for the endpoint.
*/
var vpcId: kotlin.String? = null
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.s3outposts.model.Endpoint) : this() {
this.accessType = x.accessType
this.cidrBlock = x.cidrBlock
this.creationTime = x.creationTime
this.customerOwnedIpv4Pool = x.customerOwnedIpv4Pool
this.endpointArn = x.endpointArn
this.networkInterfaces = x.networkInterfaces
this.outpostsId = x.outpostsId
this.securityGroupId = x.securityGroupId
this.status = x.status
this.subnetId = x.subnetId
this.vpcId = x.vpcId
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.s3outposts.model.Endpoint = Endpoint(this)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy