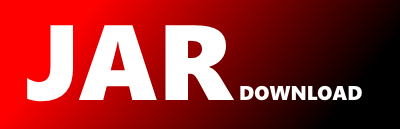
aws.sdk.kotlin.services.sagemaker.model.CreateAlgorithmRequest.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.sagemaker.model
class CreateAlgorithmRequest private constructor(builder: Builder) {
/**
* A description of the algorithm.
*/
val algorithmDescription: kotlin.String? = builder.algorithmDescription
/**
* The name of the algorithm.
*/
val algorithmName: kotlin.String? = builder.algorithmName
/**
* Whether to certify the algorithm so that it can be listed in Amazon Web Services Marketplace.
*/
val certifyForMarketplace: kotlin.Boolean = builder.certifyForMarketplace
/**
* Specifies details about inference jobs that the algorithm runs, including the
* following:
* The Amazon ECR paths of containers that contain the inference code and model
* artifacts.
* The instance types that the algorithm supports for transform jobs and
* real-time endpoints used for inference.
* The input and output content formats that the algorithm supports for
* inference.
*/
val inferenceSpecification: aws.sdk.kotlin.services.sagemaker.model.InferenceSpecification? = builder.inferenceSpecification
/**
* An array of key-value pairs. You can use tags to categorize your Amazon Web Services resources in
* different ways, for example, by purpose, owner, or environment. For more information,
* see Tagging Amazon Web Services
* Resources.
*/
val tags: List? = builder.tags
/**
* Specifies details about training jobs run by this algorithm, including the
* following:
* The Amazon ECR path of the container and the version digest of the
* algorithm.
* The hyperparameters that the algorithm supports.
* The instance types that the algorithm supports for training.
* Whether the algorithm supports distributed training.
* The metrics that the algorithm emits to Amazon CloudWatch.
* Which metrics that the algorithm emits can be used as the objective metric for
* hyperparameter tuning jobs.
* The input channels that the algorithm supports for training data. For example,
* an algorithm might support train, validation, and
* test channels.
*/
val trainingSpecification: aws.sdk.kotlin.services.sagemaker.model.TrainingSpecification? = builder.trainingSpecification
/**
* Specifies configurations for one or more training jobs and that Amazon SageMaker runs to test the
* algorithm's training code and, optionally, one or more batch transform jobs that Amazon SageMaker
* runs to test the algorithm's inference code.
*/
val validationSpecification: aws.sdk.kotlin.services.sagemaker.model.AlgorithmValidationSpecification? = builder.validationSpecification
companion object {
operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.sagemaker.model.CreateAlgorithmRequest = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("CreateAlgorithmRequest(")
append("algorithmDescription=$algorithmDescription,")
append("algorithmName=$algorithmName,")
append("certifyForMarketplace=$certifyForMarketplace,")
append("inferenceSpecification=$inferenceSpecification,")
append("tags=$tags,")
append("trainingSpecification=$trainingSpecification,")
append("validationSpecification=$validationSpecification)")
}
override fun hashCode(): kotlin.Int {
var result = algorithmDescription?.hashCode() ?: 0
result = 31 * result + (algorithmName?.hashCode() ?: 0)
result = 31 * result + (certifyForMarketplace.hashCode())
result = 31 * result + (inferenceSpecification?.hashCode() ?: 0)
result = 31 * result + (tags?.hashCode() ?: 0)
result = 31 * result + (trainingSpecification?.hashCode() ?: 0)
result = 31 * result + (validationSpecification?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as CreateAlgorithmRequest
if (algorithmDescription != other.algorithmDescription) return false
if (algorithmName != other.algorithmName) return false
if (certifyForMarketplace != other.certifyForMarketplace) return false
if (inferenceSpecification != other.inferenceSpecification) return false
if (tags != other.tags) return false
if (trainingSpecification != other.trainingSpecification) return false
if (validationSpecification != other.validationSpecification) return false
return true
}
inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.sagemaker.model.CreateAlgorithmRequest = Builder(this).apply(block).build()
class Builder {
/**
* A description of the algorithm.
*/
var algorithmDescription: kotlin.String? = null
/**
* The name of the algorithm.
*/
var algorithmName: kotlin.String? = null
/**
* Whether to certify the algorithm so that it can be listed in Amazon Web Services Marketplace.
*/
var certifyForMarketplace: kotlin.Boolean = false
/**
* Specifies details about inference jobs that the algorithm runs, including the
* following:
* The Amazon ECR paths of containers that contain the inference code and model
* artifacts.
* The instance types that the algorithm supports for transform jobs and
* real-time endpoints used for inference.
* The input and output content formats that the algorithm supports for
* inference.
*/
var inferenceSpecification: aws.sdk.kotlin.services.sagemaker.model.InferenceSpecification? = null
/**
* An array of key-value pairs. You can use tags to categorize your Amazon Web Services resources in
* different ways, for example, by purpose, owner, or environment. For more information,
* see Tagging Amazon Web Services
* Resources.
*/
var tags: List? = null
/**
* Specifies details about training jobs run by this algorithm, including the
* following:
* The Amazon ECR path of the container and the version digest of the
* algorithm.
* The hyperparameters that the algorithm supports.
* The instance types that the algorithm supports for training.
* Whether the algorithm supports distributed training.
* The metrics that the algorithm emits to Amazon CloudWatch.
* Which metrics that the algorithm emits can be used as the objective metric for
* hyperparameter tuning jobs.
* The input channels that the algorithm supports for training data. For example,
* an algorithm might support train, validation, and
* test channels.
*/
var trainingSpecification: aws.sdk.kotlin.services.sagemaker.model.TrainingSpecification? = null
/**
* Specifies configurations for one or more training jobs and that Amazon SageMaker runs to test the
* algorithm's training code and, optionally, one or more batch transform jobs that Amazon SageMaker
* runs to test the algorithm's inference code.
*/
var validationSpecification: aws.sdk.kotlin.services.sagemaker.model.AlgorithmValidationSpecification? = null
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.sagemaker.model.CreateAlgorithmRequest) : this() {
this.algorithmDescription = x.algorithmDescription
this.algorithmName = x.algorithmName
this.certifyForMarketplace = x.certifyForMarketplace
this.inferenceSpecification = x.inferenceSpecification
this.tags = x.tags
this.trainingSpecification = x.trainingSpecification
this.validationSpecification = x.validationSpecification
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.sagemaker.model.CreateAlgorithmRequest = CreateAlgorithmRequest(this)
/**
* construct an [aws.sdk.kotlin.services.sagemaker.model.InferenceSpecification] inside the given [block]
*/
fun inferenceSpecification(block: aws.sdk.kotlin.services.sagemaker.model.InferenceSpecification.Builder.() -> kotlin.Unit) {
this.inferenceSpecification = aws.sdk.kotlin.services.sagemaker.model.InferenceSpecification.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.sagemaker.model.TrainingSpecification] inside the given [block]
*/
fun trainingSpecification(block: aws.sdk.kotlin.services.sagemaker.model.TrainingSpecification.Builder.() -> kotlin.Unit) {
this.trainingSpecification = aws.sdk.kotlin.services.sagemaker.model.TrainingSpecification.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.sagemaker.model.AlgorithmValidationSpecification] inside the given [block]
*/
fun validationSpecification(block: aws.sdk.kotlin.services.sagemaker.model.AlgorithmValidationSpecification.Builder.() -> kotlin.Unit) {
this.validationSpecification = aws.sdk.kotlin.services.sagemaker.model.AlgorithmValidationSpecification.invoke(block)
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy