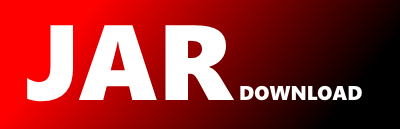
aws.sdk.kotlin.services.sagemaker.model.CreateCompilationJobRequest.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.sagemaker.model
class CreateCompilationJobRequest private constructor(builder: Builder) {
/**
* A name for the model compilation job. The name must be unique within the Amazon Web Services Region
* and within your Amazon Web Services account.
*/
val compilationJobName: kotlin.String? = builder.compilationJobName
/**
* Provides information about the location of input model artifacts, the name and shape
* of the expected data inputs, and the framework in which the model was trained.
*/
val inputConfig: aws.sdk.kotlin.services.sagemaker.model.InputConfig? = builder.inputConfig
/**
* The Amazon Resource Name (ARN) of a versioned model package. Provide either a
* ModelPackageVersionArn or an InputConfig object in the
* request syntax. The presence of both objects in the CreateCompilationJob
* request will return an exception.
*/
val modelPackageVersionArn: kotlin.String? = builder.modelPackageVersionArn
/**
* Provides information about the output location for the compiled model and the target
* device the model runs on.
*/
val outputConfig: aws.sdk.kotlin.services.sagemaker.model.OutputConfig? = builder.outputConfig
/**
* The Amazon Resource Name (ARN) of an IAM role that enables Amazon SageMaker to perform tasks on
* your behalf.
* During model compilation, Amazon SageMaker needs your permission to:
* Read input data from an S3 bucket
* Write model artifacts to an S3 bucket
* Write logs to Amazon CloudWatch Logs
* Publish metrics to Amazon CloudWatch
* You grant permissions for all of these tasks to an IAM role. To pass this role to
* Amazon SageMaker, the caller of this API must have the iam:PassRole permission. For
* more information, see Amazon SageMaker
* Roles.
*/
val roleArn: kotlin.String? = builder.roleArn
/**
* Specifies a limit to how long a model compilation job can run. When the job reaches
* the time limit, Amazon SageMaker ends the compilation job. Use this API to cap model training
* costs.
*/
val stoppingCondition: aws.sdk.kotlin.services.sagemaker.model.StoppingCondition? = builder.stoppingCondition
/**
* An array of key-value pairs. You can use tags to categorize your Amazon Web Services resources in
* different ways, for example, by purpose, owner, or environment. For more information,
* see Tagging Amazon Web Services
* Resources.
*/
val tags: List? = builder.tags
/**
* A VpcConfig object that specifies the VPC that you want your
* compilation job to connect to. Control access to your models by
* configuring the VPC. For more information, see Protect Compilation Jobs by Using an Amazon
* Virtual Private Cloud.
*/
val vpcConfig: aws.sdk.kotlin.services.sagemaker.model.NeoVpcConfig? = builder.vpcConfig
companion object {
operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.sagemaker.model.CreateCompilationJobRequest = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("CreateCompilationJobRequest(")
append("compilationJobName=$compilationJobName,")
append("inputConfig=$inputConfig,")
append("modelPackageVersionArn=$modelPackageVersionArn,")
append("outputConfig=$outputConfig,")
append("roleArn=$roleArn,")
append("stoppingCondition=$stoppingCondition,")
append("tags=$tags,")
append("vpcConfig=$vpcConfig)")
}
override fun hashCode(): kotlin.Int {
var result = compilationJobName?.hashCode() ?: 0
result = 31 * result + (inputConfig?.hashCode() ?: 0)
result = 31 * result + (modelPackageVersionArn?.hashCode() ?: 0)
result = 31 * result + (outputConfig?.hashCode() ?: 0)
result = 31 * result + (roleArn?.hashCode() ?: 0)
result = 31 * result + (stoppingCondition?.hashCode() ?: 0)
result = 31 * result + (tags?.hashCode() ?: 0)
result = 31 * result + (vpcConfig?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as CreateCompilationJobRequest
if (compilationJobName != other.compilationJobName) return false
if (inputConfig != other.inputConfig) return false
if (modelPackageVersionArn != other.modelPackageVersionArn) return false
if (outputConfig != other.outputConfig) return false
if (roleArn != other.roleArn) return false
if (stoppingCondition != other.stoppingCondition) return false
if (tags != other.tags) return false
if (vpcConfig != other.vpcConfig) return false
return true
}
inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.sagemaker.model.CreateCompilationJobRequest = Builder(this).apply(block).build()
class Builder {
/**
* A name for the model compilation job. The name must be unique within the Amazon Web Services Region
* and within your Amazon Web Services account.
*/
var compilationJobName: kotlin.String? = null
/**
* Provides information about the location of input model artifacts, the name and shape
* of the expected data inputs, and the framework in which the model was trained.
*/
var inputConfig: aws.sdk.kotlin.services.sagemaker.model.InputConfig? = null
/**
* The Amazon Resource Name (ARN) of a versioned model package. Provide either a
* ModelPackageVersionArn or an InputConfig object in the
* request syntax. The presence of both objects in the CreateCompilationJob
* request will return an exception.
*/
var modelPackageVersionArn: kotlin.String? = null
/**
* Provides information about the output location for the compiled model and the target
* device the model runs on.
*/
var outputConfig: aws.sdk.kotlin.services.sagemaker.model.OutputConfig? = null
/**
* The Amazon Resource Name (ARN) of an IAM role that enables Amazon SageMaker to perform tasks on
* your behalf.
* During model compilation, Amazon SageMaker needs your permission to:
* Read input data from an S3 bucket
* Write model artifacts to an S3 bucket
* Write logs to Amazon CloudWatch Logs
* Publish metrics to Amazon CloudWatch
* You grant permissions for all of these tasks to an IAM role. To pass this role to
* Amazon SageMaker, the caller of this API must have the iam:PassRole permission. For
* more information, see Amazon SageMaker
* Roles.
*/
var roleArn: kotlin.String? = null
/**
* Specifies a limit to how long a model compilation job can run. When the job reaches
* the time limit, Amazon SageMaker ends the compilation job. Use this API to cap model training
* costs.
*/
var stoppingCondition: aws.sdk.kotlin.services.sagemaker.model.StoppingCondition? = null
/**
* An array of key-value pairs. You can use tags to categorize your Amazon Web Services resources in
* different ways, for example, by purpose, owner, or environment. For more information,
* see Tagging Amazon Web Services
* Resources.
*/
var tags: List? = null
/**
* A VpcConfig object that specifies the VPC that you want your
* compilation job to connect to. Control access to your models by
* configuring the VPC. For more information, see Protect Compilation Jobs by Using an Amazon
* Virtual Private Cloud.
*/
var vpcConfig: aws.sdk.kotlin.services.sagemaker.model.NeoVpcConfig? = null
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.sagemaker.model.CreateCompilationJobRequest) : this() {
this.compilationJobName = x.compilationJobName
this.inputConfig = x.inputConfig
this.modelPackageVersionArn = x.modelPackageVersionArn
this.outputConfig = x.outputConfig
this.roleArn = x.roleArn
this.stoppingCondition = x.stoppingCondition
this.tags = x.tags
this.vpcConfig = x.vpcConfig
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.sagemaker.model.CreateCompilationJobRequest = CreateCompilationJobRequest(this)
/**
* construct an [aws.sdk.kotlin.services.sagemaker.model.InputConfig] inside the given [block]
*/
fun inputConfig(block: aws.sdk.kotlin.services.sagemaker.model.InputConfig.Builder.() -> kotlin.Unit) {
this.inputConfig = aws.sdk.kotlin.services.sagemaker.model.InputConfig.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.sagemaker.model.OutputConfig] inside the given [block]
*/
fun outputConfig(block: aws.sdk.kotlin.services.sagemaker.model.OutputConfig.Builder.() -> kotlin.Unit) {
this.outputConfig = aws.sdk.kotlin.services.sagemaker.model.OutputConfig.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.sagemaker.model.StoppingCondition] inside the given [block]
*/
fun stoppingCondition(block: aws.sdk.kotlin.services.sagemaker.model.StoppingCondition.Builder.() -> kotlin.Unit) {
this.stoppingCondition = aws.sdk.kotlin.services.sagemaker.model.StoppingCondition.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.sagemaker.model.NeoVpcConfig] inside the given [block]
*/
fun vpcConfig(block: aws.sdk.kotlin.services.sagemaker.model.NeoVpcConfig.Builder.() -> kotlin.Unit) {
this.vpcConfig = aws.sdk.kotlin.services.sagemaker.model.NeoVpcConfig.invoke(block)
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy