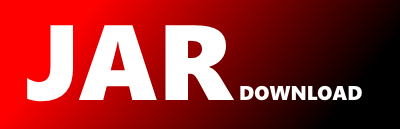
aws.sdk.kotlin.services.sagemaker.model.CreateDataQualityJobDefinitionRequest.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.sagemaker.model
class CreateDataQualityJobDefinitionRequest private constructor(builder: Builder) {
/**
* Specifies the container that runs the monitoring job.
*/
val dataQualityAppSpecification: aws.sdk.kotlin.services.sagemaker.model.DataQualityAppSpecification? = builder.dataQualityAppSpecification
/**
* Configures the constraints and baselines for the monitoring job.
*/
val dataQualityBaselineConfig: aws.sdk.kotlin.services.sagemaker.model.DataQualityBaselineConfig? = builder.dataQualityBaselineConfig
/**
* A list of inputs for the monitoring job. Currently endpoints are supported as monitoring
* inputs.
*/
val dataQualityJobInput: aws.sdk.kotlin.services.sagemaker.model.DataQualityJobInput? = builder.dataQualityJobInput
/**
* The output configuration for monitoring jobs.
*/
val dataQualityJobOutputConfig: aws.sdk.kotlin.services.sagemaker.model.MonitoringOutputConfig? = builder.dataQualityJobOutputConfig
/**
* The name for the monitoring job definition.
*/
val jobDefinitionName: kotlin.String? = builder.jobDefinitionName
/**
* Identifies the resources to deploy for a monitoring job.
*/
val jobResources: aws.sdk.kotlin.services.sagemaker.model.MonitoringResources? = builder.jobResources
/**
* Specifies networking configuration for the monitoring job.
*/
val networkConfig: aws.sdk.kotlin.services.sagemaker.model.MonitoringNetworkConfig? = builder.networkConfig
/**
* The Amazon Resource Name (ARN) of an IAM role that Amazon SageMaker can assume to
* perform tasks on your behalf.
*/
val roleArn: kotlin.String? = builder.roleArn
/**
* A time limit for how long the monitoring job is allowed to run before stopping.
*/
val stoppingCondition: aws.sdk.kotlin.services.sagemaker.model.MonitoringStoppingCondition? = builder.stoppingCondition
/**
* (Optional) An array of key-value pairs. For more information, see Using Cost Allocation Tags in the Amazon Web Services Billing and Cost Management
* User Guide.
*/
val tags: List? = builder.tags
companion object {
operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.sagemaker.model.CreateDataQualityJobDefinitionRequest = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("CreateDataQualityJobDefinitionRequest(")
append("dataQualityAppSpecification=$dataQualityAppSpecification,")
append("dataQualityBaselineConfig=$dataQualityBaselineConfig,")
append("dataQualityJobInput=$dataQualityJobInput,")
append("dataQualityJobOutputConfig=$dataQualityJobOutputConfig,")
append("jobDefinitionName=$jobDefinitionName,")
append("jobResources=$jobResources,")
append("networkConfig=$networkConfig,")
append("roleArn=$roleArn,")
append("stoppingCondition=$stoppingCondition,")
append("tags=$tags)")
}
override fun hashCode(): kotlin.Int {
var result = dataQualityAppSpecification?.hashCode() ?: 0
result = 31 * result + (dataQualityBaselineConfig?.hashCode() ?: 0)
result = 31 * result + (dataQualityJobInput?.hashCode() ?: 0)
result = 31 * result + (dataQualityJobOutputConfig?.hashCode() ?: 0)
result = 31 * result + (jobDefinitionName?.hashCode() ?: 0)
result = 31 * result + (jobResources?.hashCode() ?: 0)
result = 31 * result + (networkConfig?.hashCode() ?: 0)
result = 31 * result + (roleArn?.hashCode() ?: 0)
result = 31 * result + (stoppingCondition?.hashCode() ?: 0)
result = 31 * result + (tags?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as CreateDataQualityJobDefinitionRequest
if (dataQualityAppSpecification != other.dataQualityAppSpecification) return false
if (dataQualityBaselineConfig != other.dataQualityBaselineConfig) return false
if (dataQualityJobInput != other.dataQualityJobInput) return false
if (dataQualityJobOutputConfig != other.dataQualityJobOutputConfig) return false
if (jobDefinitionName != other.jobDefinitionName) return false
if (jobResources != other.jobResources) return false
if (networkConfig != other.networkConfig) return false
if (roleArn != other.roleArn) return false
if (stoppingCondition != other.stoppingCondition) return false
if (tags != other.tags) return false
return true
}
inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.sagemaker.model.CreateDataQualityJobDefinitionRequest = Builder(this).apply(block).build()
class Builder {
/**
* Specifies the container that runs the monitoring job.
*/
var dataQualityAppSpecification: aws.sdk.kotlin.services.sagemaker.model.DataQualityAppSpecification? = null
/**
* Configures the constraints and baselines for the monitoring job.
*/
var dataQualityBaselineConfig: aws.sdk.kotlin.services.sagemaker.model.DataQualityBaselineConfig? = null
/**
* A list of inputs for the monitoring job. Currently endpoints are supported as monitoring
* inputs.
*/
var dataQualityJobInput: aws.sdk.kotlin.services.sagemaker.model.DataQualityJobInput? = null
/**
* The output configuration for monitoring jobs.
*/
var dataQualityJobOutputConfig: aws.sdk.kotlin.services.sagemaker.model.MonitoringOutputConfig? = null
/**
* The name for the monitoring job definition.
*/
var jobDefinitionName: kotlin.String? = null
/**
* Identifies the resources to deploy for a monitoring job.
*/
var jobResources: aws.sdk.kotlin.services.sagemaker.model.MonitoringResources? = null
/**
* Specifies networking configuration for the monitoring job.
*/
var networkConfig: aws.sdk.kotlin.services.sagemaker.model.MonitoringNetworkConfig? = null
/**
* The Amazon Resource Name (ARN) of an IAM role that Amazon SageMaker can assume to
* perform tasks on your behalf.
*/
var roleArn: kotlin.String? = null
/**
* A time limit for how long the monitoring job is allowed to run before stopping.
*/
var stoppingCondition: aws.sdk.kotlin.services.sagemaker.model.MonitoringStoppingCondition? = null
/**
* (Optional) An array of key-value pairs. For more information, see Using Cost Allocation Tags in the Amazon Web Services Billing and Cost Management
* User Guide.
*/
var tags: List? = null
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.sagemaker.model.CreateDataQualityJobDefinitionRequest) : this() {
this.dataQualityAppSpecification = x.dataQualityAppSpecification
this.dataQualityBaselineConfig = x.dataQualityBaselineConfig
this.dataQualityJobInput = x.dataQualityJobInput
this.dataQualityJobOutputConfig = x.dataQualityJobOutputConfig
this.jobDefinitionName = x.jobDefinitionName
this.jobResources = x.jobResources
this.networkConfig = x.networkConfig
this.roleArn = x.roleArn
this.stoppingCondition = x.stoppingCondition
this.tags = x.tags
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.sagemaker.model.CreateDataQualityJobDefinitionRequest = CreateDataQualityJobDefinitionRequest(this)
/**
* construct an [aws.sdk.kotlin.services.sagemaker.model.DataQualityAppSpecification] inside the given [block]
*/
fun dataQualityAppSpecification(block: aws.sdk.kotlin.services.sagemaker.model.DataQualityAppSpecification.Builder.() -> kotlin.Unit) {
this.dataQualityAppSpecification = aws.sdk.kotlin.services.sagemaker.model.DataQualityAppSpecification.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.sagemaker.model.DataQualityBaselineConfig] inside the given [block]
*/
fun dataQualityBaselineConfig(block: aws.sdk.kotlin.services.sagemaker.model.DataQualityBaselineConfig.Builder.() -> kotlin.Unit) {
this.dataQualityBaselineConfig = aws.sdk.kotlin.services.sagemaker.model.DataQualityBaselineConfig.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.sagemaker.model.DataQualityJobInput] inside the given [block]
*/
fun dataQualityJobInput(block: aws.sdk.kotlin.services.sagemaker.model.DataQualityJobInput.Builder.() -> kotlin.Unit) {
this.dataQualityJobInput = aws.sdk.kotlin.services.sagemaker.model.DataQualityJobInput.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.sagemaker.model.MonitoringOutputConfig] inside the given [block]
*/
fun dataQualityJobOutputConfig(block: aws.sdk.kotlin.services.sagemaker.model.MonitoringOutputConfig.Builder.() -> kotlin.Unit) {
this.dataQualityJobOutputConfig = aws.sdk.kotlin.services.sagemaker.model.MonitoringOutputConfig.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.sagemaker.model.MonitoringResources] inside the given [block]
*/
fun jobResources(block: aws.sdk.kotlin.services.sagemaker.model.MonitoringResources.Builder.() -> kotlin.Unit) {
this.jobResources = aws.sdk.kotlin.services.sagemaker.model.MonitoringResources.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.sagemaker.model.MonitoringNetworkConfig] inside the given [block]
*/
fun networkConfig(block: aws.sdk.kotlin.services.sagemaker.model.MonitoringNetworkConfig.Builder.() -> kotlin.Unit) {
this.networkConfig = aws.sdk.kotlin.services.sagemaker.model.MonitoringNetworkConfig.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.sagemaker.model.MonitoringStoppingCondition] inside the given [block]
*/
fun stoppingCondition(block: aws.sdk.kotlin.services.sagemaker.model.MonitoringStoppingCondition.Builder.() -> kotlin.Unit) {
this.stoppingCondition = aws.sdk.kotlin.services.sagemaker.model.MonitoringStoppingCondition.invoke(block)
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy