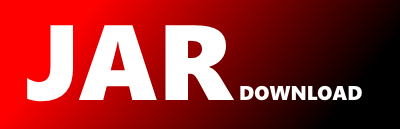
aws.sdk.kotlin.services.sagemaker.model.DescribeAppResponse.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.sagemaker.model
import aws.smithy.kotlin.runtime.time.Instant
class DescribeAppResponse private constructor(builder: Builder) {
/**
* The Amazon Resource Name (ARN) of the app.
*/
val appArn: kotlin.String? = builder.appArn
/**
* The name of the app.
*/
val appName: kotlin.String? = builder.appName
/**
* The type of app.
*/
val appType: aws.sdk.kotlin.services.sagemaker.model.AppType? = builder.appType
/**
* The creation time.
*/
val creationTime: aws.smithy.kotlin.runtime.time.Instant? = builder.creationTime
/**
* The domain ID.
*/
val domainId: kotlin.String? = builder.domainId
/**
* The failure reason.
*/
val failureReason: kotlin.String? = builder.failureReason
/**
* The timestamp of the last health check.
*/
val lastHealthCheckTimestamp: aws.smithy.kotlin.runtime.time.Instant? = builder.lastHealthCheckTimestamp
/**
* The timestamp of the last user's activity. LastUserActivityTimestamp is also updated when SageMaker performs health checks without user activity. As a result, this value is set to the same value as LastHealthCheckTimestamp.
*/
val lastUserActivityTimestamp: aws.smithy.kotlin.runtime.time.Instant? = builder.lastUserActivityTimestamp
/**
* The instance type and the Amazon Resource Name (ARN) of the SageMaker image created on the instance.
*/
val resourceSpec: aws.sdk.kotlin.services.sagemaker.model.ResourceSpec? = builder.resourceSpec
/**
* The status.
*/
val status: aws.sdk.kotlin.services.sagemaker.model.AppStatus? = builder.status
/**
* The user profile name.
*/
val userProfileName: kotlin.String? = builder.userProfileName
companion object {
operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.sagemaker.model.DescribeAppResponse = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("DescribeAppResponse(")
append("appArn=$appArn,")
append("appName=$appName,")
append("appType=$appType,")
append("creationTime=$creationTime,")
append("domainId=$domainId,")
append("failureReason=$failureReason,")
append("lastHealthCheckTimestamp=$lastHealthCheckTimestamp,")
append("lastUserActivityTimestamp=$lastUserActivityTimestamp,")
append("resourceSpec=$resourceSpec,")
append("status=$status,")
append("userProfileName=$userProfileName)")
}
override fun hashCode(): kotlin.Int {
var result = appArn?.hashCode() ?: 0
result = 31 * result + (appName?.hashCode() ?: 0)
result = 31 * result + (appType?.hashCode() ?: 0)
result = 31 * result + (creationTime?.hashCode() ?: 0)
result = 31 * result + (domainId?.hashCode() ?: 0)
result = 31 * result + (failureReason?.hashCode() ?: 0)
result = 31 * result + (lastHealthCheckTimestamp?.hashCode() ?: 0)
result = 31 * result + (lastUserActivityTimestamp?.hashCode() ?: 0)
result = 31 * result + (resourceSpec?.hashCode() ?: 0)
result = 31 * result + (status?.hashCode() ?: 0)
result = 31 * result + (userProfileName?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as DescribeAppResponse
if (appArn != other.appArn) return false
if (appName != other.appName) return false
if (appType != other.appType) return false
if (creationTime != other.creationTime) return false
if (domainId != other.domainId) return false
if (failureReason != other.failureReason) return false
if (lastHealthCheckTimestamp != other.lastHealthCheckTimestamp) return false
if (lastUserActivityTimestamp != other.lastUserActivityTimestamp) return false
if (resourceSpec != other.resourceSpec) return false
if (status != other.status) return false
if (userProfileName != other.userProfileName) return false
return true
}
inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.sagemaker.model.DescribeAppResponse = Builder(this).apply(block).build()
class Builder {
/**
* The Amazon Resource Name (ARN) of the app.
*/
var appArn: kotlin.String? = null
/**
* The name of the app.
*/
var appName: kotlin.String? = null
/**
* The type of app.
*/
var appType: aws.sdk.kotlin.services.sagemaker.model.AppType? = null
/**
* The creation time.
*/
var creationTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The domain ID.
*/
var domainId: kotlin.String? = null
/**
* The failure reason.
*/
var failureReason: kotlin.String? = null
/**
* The timestamp of the last health check.
*/
var lastHealthCheckTimestamp: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The timestamp of the last user's activity. LastUserActivityTimestamp is also updated when SageMaker performs health checks without user activity. As a result, this value is set to the same value as LastHealthCheckTimestamp.
*/
var lastUserActivityTimestamp: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The instance type and the Amazon Resource Name (ARN) of the SageMaker image created on the instance.
*/
var resourceSpec: aws.sdk.kotlin.services.sagemaker.model.ResourceSpec? = null
/**
* The status.
*/
var status: aws.sdk.kotlin.services.sagemaker.model.AppStatus? = null
/**
* The user profile name.
*/
var userProfileName: kotlin.String? = null
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.sagemaker.model.DescribeAppResponse) : this() {
this.appArn = x.appArn
this.appName = x.appName
this.appType = x.appType
this.creationTime = x.creationTime
this.domainId = x.domainId
this.failureReason = x.failureReason
this.lastHealthCheckTimestamp = x.lastHealthCheckTimestamp
this.lastUserActivityTimestamp = x.lastUserActivityTimestamp
this.resourceSpec = x.resourceSpec
this.status = x.status
this.userProfileName = x.userProfileName
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.sagemaker.model.DescribeAppResponse = DescribeAppResponse(this)
/**
* construct an [aws.sdk.kotlin.services.sagemaker.model.ResourceSpec] inside the given [block]
*/
fun resourceSpec(block: aws.sdk.kotlin.services.sagemaker.model.ResourceSpec.Builder.() -> kotlin.Unit) {
this.resourceSpec = aws.sdk.kotlin.services.sagemaker.model.ResourceSpec.invoke(block)
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy