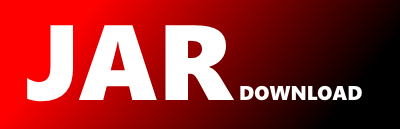
aws.sdk.kotlin.services.sagemaker.model.DescribeModelResponse.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.sagemaker.model
import aws.smithy.kotlin.runtime.time.Instant
class DescribeModelResponse private constructor(builder: Builder) {
/**
* The containers in the inference pipeline.
*/
val containers: List? = builder.containers
/**
* A timestamp that shows when the model was created.
*/
val creationTime: aws.smithy.kotlin.runtime.time.Instant? = builder.creationTime
/**
* If True, no inbound or outbound network calls can be made to or from the
* model container.
*/
val enableNetworkIsolation: kotlin.Boolean = builder.enableNetworkIsolation
/**
* The Amazon Resource Name (ARN) of the IAM role that you specified for the
* model.
*/
val executionRoleArn: kotlin.String? = builder.executionRoleArn
/**
* Specifies details of how containers in a multi-container endpoint are called.
*/
val inferenceExecutionConfig: aws.sdk.kotlin.services.sagemaker.model.InferenceExecutionConfig? = builder.inferenceExecutionConfig
/**
* The Amazon Resource Name (ARN) of the model.
*/
val modelArn: kotlin.String? = builder.modelArn
/**
* Name of the Amazon SageMaker model.
*/
val modelName: kotlin.String? = builder.modelName
/**
* The location of the primary inference code, associated artifacts, and custom
* environment map that the inference code uses when it is deployed in production.
*/
val primaryContainer: aws.sdk.kotlin.services.sagemaker.model.ContainerDefinition? = builder.primaryContainer
/**
* A VpcConfig object that specifies the VPC that this model has access
* to. For more information, see Protect Endpoints by Using an Amazon Virtual
* Private Cloud
*/
val vpcConfig: aws.sdk.kotlin.services.sagemaker.model.VpcConfig? = builder.vpcConfig
companion object {
operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.sagemaker.model.DescribeModelResponse = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("DescribeModelResponse(")
append("containers=$containers,")
append("creationTime=$creationTime,")
append("enableNetworkIsolation=$enableNetworkIsolation,")
append("executionRoleArn=$executionRoleArn,")
append("inferenceExecutionConfig=$inferenceExecutionConfig,")
append("modelArn=$modelArn,")
append("modelName=$modelName,")
append("primaryContainer=$primaryContainer,")
append("vpcConfig=$vpcConfig)")
}
override fun hashCode(): kotlin.Int {
var result = containers?.hashCode() ?: 0
result = 31 * result + (creationTime?.hashCode() ?: 0)
result = 31 * result + (enableNetworkIsolation.hashCode())
result = 31 * result + (executionRoleArn?.hashCode() ?: 0)
result = 31 * result + (inferenceExecutionConfig?.hashCode() ?: 0)
result = 31 * result + (modelArn?.hashCode() ?: 0)
result = 31 * result + (modelName?.hashCode() ?: 0)
result = 31 * result + (primaryContainer?.hashCode() ?: 0)
result = 31 * result + (vpcConfig?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as DescribeModelResponse
if (containers != other.containers) return false
if (creationTime != other.creationTime) return false
if (enableNetworkIsolation != other.enableNetworkIsolation) return false
if (executionRoleArn != other.executionRoleArn) return false
if (inferenceExecutionConfig != other.inferenceExecutionConfig) return false
if (modelArn != other.modelArn) return false
if (modelName != other.modelName) return false
if (primaryContainer != other.primaryContainer) return false
if (vpcConfig != other.vpcConfig) return false
return true
}
inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.sagemaker.model.DescribeModelResponse = Builder(this).apply(block).build()
class Builder {
/**
* The containers in the inference pipeline.
*/
var containers: List? = null
/**
* A timestamp that shows when the model was created.
*/
var creationTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* If True, no inbound or outbound network calls can be made to or from the
* model container.
*/
var enableNetworkIsolation: kotlin.Boolean = false
/**
* The Amazon Resource Name (ARN) of the IAM role that you specified for the
* model.
*/
var executionRoleArn: kotlin.String? = null
/**
* Specifies details of how containers in a multi-container endpoint are called.
*/
var inferenceExecutionConfig: aws.sdk.kotlin.services.sagemaker.model.InferenceExecutionConfig? = null
/**
* The Amazon Resource Name (ARN) of the model.
*/
var modelArn: kotlin.String? = null
/**
* Name of the Amazon SageMaker model.
*/
var modelName: kotlin.String? = null
/**
* The location of the primary inference code, associated artifacts, and custom
* environment map that the inference code uses when it is deployed in production.
*/
var primaryContainer: aws.sdk.kotlin.services.sagemaker.model.ContainerDefinition? = null
/**
* A VpcConfig object that specifies the VPC that this model has access
* to. For more information, see Protect Endpoints by Using an Amazon Virtual
* Private Cloud
*/
var vpcConfig: aws.sdk.kotlin.services.sagemaker.model.VpcConfig? = null
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.sagemaker.model.DescribeModelResponse) : this() {
this.containers = x.containers
this.creationTime = x.creationTime
this.enableNetworkIsolation = x.enableNetworkIsolation
this.executionRoleArn = x.executionRoleArn
this.inferenceExecutionConfig = x.inferenceExecutionConfig
this.modelArn = x.modelArn
this.modelName = x.modelName
this.primaryContainer = x.primaryContainer
this.vpcConfig = x.vpcConfig
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.sagemaker.model.DescribeModelResponse = DescribeModelResponse(this)
/**
* construct an [aws.sdk.kotlin.services.sagemaker.model.InferenceExecutionConfig] inside the given [block]
*/
fun inferenceExecutionConfig(block: aws.sdk.kotlin.services.sagemaker.model.InferenceExecutionConfig.Builder.() -> kotlin.Unit) {
this.inferenceExecutionConfig = aws.sdk.kotlin.services.sagemaker.model.InferenceExecutionConfig.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.sagemaker.model.ContainerDefinition] inside the given [block]
*/
fun primaryContainer(block: aws.sdk.kotlin.services.sagemaker.model.ContainerDefinition.Builder.() -> kotlin.Unit) {
this.primaryContainer = aws.sdk.kotlin.services.sagemaker.model.ContainerDefinition.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.sagemaker.model.VpcConfig] inside the given [block]
*/
fun vpcConfig(block: aws.sdk.kotlin.services.sagemaker.model.VpcConfig.Builder.() -> kotlin.Unit) {
this.vpcConfig = aws.sdk.kotlin.services.sagemaker.model.VpcConfig.invoke(block)
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy