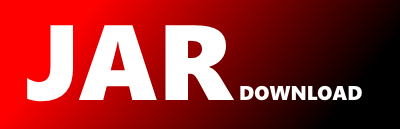
aws.sdk.kotlin.services.sagemaker.model.EndpointSummary.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.sagemaker.model
import aws.smithy.kotlin.runtime.time.Instant
/**
* Provides summary information for an endpoint.
*/
class EndpointSummary private constructor(builder: Builder) {
/**
* A timestamp that shows when the endpoint was created.
*/
val creationTime: aws.smithy.kotlin.runtime.time.Instant? = builder.creationTime
/**
* The Amazon Resource Name (ARN) of the endpoint.
*/
val endpointArn: kotlin.String? = builder.endpointArn
/**
* The name of the endpoint.
*/
val endpointName: kotlin.String? = builder.endpointName
/**
* The status of the endpoint.
* OutOfService: Endpoint is not available to take incoming
* requests.
* Creating: CreateEndpoint is executing.
* Updating: UpdateEndpoint or UpdateEndpointWeightsAndCapacities is executing.
* SystemUpdating: Endpoint is undergoing maintenance and cannot be
* updated or deleted or re-scaled until it has completed. This maintenance
* operation does not change any customer-specified values such as VPC config, KMS
* encryption, model, instance type, or instance count.
* RollingBack: Endpoint fails to scale up or down or change its
* variant weight and is in the process of rolling back to its previous
* configuration. Once the rollback completes, endpoint returns to an
* InService status. This transitional status only applies to an
* endpoint that has autoscaling enabled and is undergoing variant weight or
* capacity changes as part of an UpdateEndpointWeightsAndCapacities call or when the UpdateEndpointWeightsAndCapacities operation is called
* explicitly.
* InService: Endpoint is available to process incoming
* requests.
* Deleting: DeleteEndpoint is executing.
* Failed: Endpoint could not be created, updated, or re-scaled. Use
* DescribeEndpointOutput$FailureReason for information about
* the failure. DeleteEndpoint is the only operation that can be
* performed on a failed endpoint.
* To get a list of endpoints with a specified status, use the ListEndpointsInput$StatusEquals filter.
*/
val endpointStatus: aws.sdk.kotlin.services.sagemaker.model.EndpointStatus? = builder.endpointStatus
/**
* A timestamp that shows when the endpoint was last modified.
*/
val lastModifiedTime: aws.smithy.kotlin.runtime.time.Instant? = builder.lastModifiedTime
companion object {
operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.sagemaker.model.EndpointSummary = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("EndpointSummary(")
append("creationTime=$creationTime,")
append("endpointArn=$endpointArn,")
append("endpointName=$endpointName,")
append("endpointStatus=$endpointStatus,")
append("lastModifiedTime=$lastModifiedTime)")
}
override fun hashCode(): kotlin.Int {
var result = creationTime?.hashCode() ?: 0
result = 31 * result + (endpointArn?.hashCode() ?: 0)
result = 31 * result + (endpointName?.hashCode() ?: 0)
result = 31 * result + (endpointStatus?.hashCode() ?: 0)
result = 31 * result + (lastModifiedTime?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as EndpointSummary
if (creationTime != other.creationTime) return false
if (endpointArn != other.endpointArn) return false
if (endpointName != other.endpointName) return false
if (endpointStatus != other.endpointStatus) return false
if (lastModifiedTime != other.lastModifiedTime) return false
return true
}
inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.sagemaker.model.EndpointSummary = Builder(this).apply(block).build()
class Builder {
/**
* A timestamp that shows when the endpoint was created.
*/
var creationTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The Amazon Resource Name (ARN) of the endpoint.
*/
var endpointArn: kotlin.String? = null
/**
* The name of the endpoint.
*/
var endpointName: kotlin.String? = null
/**
* The status of the endpoint.
* OutOfService: Endpoint is not available to take incoming
* requests.
* Creating: CreateEndpoint is executing.
* Updating: UpdateEndpoint or UpdateEndpointWeightsAndCapacities is executing.
* SystemUpdating: Endpoint is undergoing maintenance and cannot be
* updated or deleted or re-scaled until it has completed. This maintenance
* operation does not change any customer-specified values such as VPC config, KMS
* encryption, model, instance type, or instance count.
* RollingBack: Endpoint fails to scale up or down or change its
* variant weight and is in the process of rolling back to its previous
* configuration. Once the rollback completes, endpoint returns to an
* InService status. This transitional status only applies to an
* endpoint that has autoscaling enabled and is undergoing variant weight or
* capacity changes as part of an UpdateEndpointWeightsAndCapacities call or when the UpdateEndpointWeightsAndCapacities operation is called
* explicitly.
* InService: Endpoint is available to process incoming
* requests.
* Deleting: DeleteEndpoint is executing.
* Failed: Endpoint could not be created, updated, or re-scaled. Use
* DescribeEndpointOutput$FailureReason for information about
* the failure. DeleteEndpoint is the only operation that can be
* performed on a failed endpoint.
* To get a list of endpoints with a specified status, use the ListEndpointsInput$StatusEquals filter.
*/
var endpointStatus: aws.sdk.kotlin.services.sagemaker.model.EndpointStatus? = null
/**
* A timestamp that shows when the endpoint was last modified.
*/
var lastModifiedTime: aws.smithy.kotlin.runtime.time.Instant? = null
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.sagemaker.model.EndpointSummary) : this() {
this.creationTime = x.creationTime
this.endpointArn = x.endpointArn
this.endpointName = x.endpointName
this.endpointStatus = x.endpointStatus
this.lastModifiedTime = x.lastModifiedTime
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.sagemaker.model.EndpointSummary = EndpointSummary(this)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy