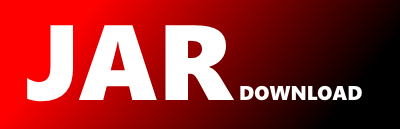
aws.sdk.kotlin.services.sagemaker.model.FileSystemDataSource.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.sagemaker.model
/**
* Specifies a file system data source for a channel.
*/
class FileSystemDataSource private constructor(builder: Builder) {
/**
* The full path to the directory to associate with the channel.
*/
val directoryPath: kotlin.String? = builder.directoryPath
/**
* The access mode of the mount of the directory associated with the channel. A directory
* can be mounted either in ro (read-only) or rw (read-write)
* mode.
*/
val fileSystemAccessMode: aws.sdk.kotlin.services.sagemaker.model.FileSystemAccessMode? = builder.fileSystemAccessMode
/**
* The file system id.
*/
val fileSystemId: kotlin.String? = builder.fileSystemId
/**
* The file system type.
*/
val fileSystemType: aws.sdk.kotlin.services.sagemaker.model.FileSystemType? = builder.fileSystemType
companion object {
operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.sagemaker.model.FileSystemDataSource = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("FileSystemDataSource(")
append("directoryPath=$directoryPath,")
append("fileSystemAccessMode=$fileSystemAccessMode,")
append("fileSystemId=$fileSystemId,")
append("fileSystemType=$fileSystemType)")
}
override fun hashCode(): kotlin.Int {
var result = directoryPath?.hashCode() ?: 0
result = 31 * result + (fileSystemAccessMode?.hashCode() ?: 0)
result = 31 * result + (fileSystemId?.hashCode() ?: 0)
result = 31 * result + (fileSystemType?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as FileSystemDataSource
if (directoryPath != other.directoryPath) return false
if (fileSystemAccessMode != other.fileSystemAccessMode) return false
if (fileSystemId != other.fileSystemId) return false
if (fileSystemType != other.fileSystemType) return false
return true
}
inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.sagemaker.model.FileSystemDataSource = Builder(this).apply(block).build()
class Builder {
/**
* The full path to the directory to associate with the channel.
*/
var directoryPath: kotlin.String? = null
/**
* The access mode of the mount of the directory associated with the channel. A directory
* can be mounted either in ro (read-only) or rw (read-write)
* mode.
*/
var fileSystemAccessMode: aws.sdk.kotlin.services.sagemaker.model.FileSystemAccessMode? = null
/**
* The file system id.
*/
var fileSystemId: kotlin.String? = null
/**
* The file system type.
*/
var fileSystemType: aws.sdk.kotlin.services.sagemaker.model.FileSystemType? = null
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.sagemaker.model.FileSystemDataSource) : this() {
this.directoryPath = x.directoryPath
this.fileSystemAccessMode = x.fileSystemAccessMode
this.fileSystemId = x.fileSystemId
this.fileSystemType = x.fileSystemType
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.sagemaker.model.FileSystemDataSource = FileSystemDataSource(this)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy