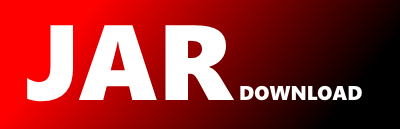
aws.sdk.kotlin.services.sagemaker.model.HyperParameterTuningJobSummary.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.sagemaker.model
import aws.smithy.kotlin.runtime.time.Instant
/**
* Provides summary information about a hyperparameter tuning job.
*/
class HyperParameterTuningJobSummary private constructor(builder: Builder) {
/**
* The date and time that the tuning job was created.
*/
val creationTime: aws.smithy.kotlin.runtime.time.Instant? = builder.creationTime
/**
* The date and time that the tuning job ended.
*/
val hyperParameterTuningEndTime: aws.smithy.kotlin.runtime.time.Instant? = builder.hyperParameterTuningEndTime
/**
* The
* Amazon
* Resource Name (ARN) of the tuning job.
*/
val hyperParameterTuningJobArn: kotlin.String? = builder.hyperParameterTuningJobArn
/**
* The name of the tuning job.
*/
val hyperParameterTuningJobName: kotlin.String? = builder.hyperParameterTuningJobName
/**
* The status of the
* tuning
* job.
*/
val hyperParameterTuningJobStatus: aws.sdk.kotlin.services.sagemaker.model.HyperParameterTuningJobStatus? = builder.hyperParameterTuningJobStatus
/**
* The date and time that the tuning job was
* modified.
*/
val lastModifiedTime: aws.smithy.kotlin.runtime.time.Instant? = builder.lastModifiedTime
/**
* The ObjectiveStatusCounters object that specifies the numbers of
* training jobs, categorized by objective metric status, that this tuning job
* launched.
*/
val objectiveStatusCounters: aws.sdk.kotlin.services.sagemaker.model.ObjectiveStatusCounters? = builder.objectiveStatusCounters
/**
* The ResourceLimits object that specifies the maximum number of
* training jobs and parallel training jobs allowed for this tuning job.
*/
val resourceLimits: aws.sdk.kotlin.services.sagemaker.model.ResourceLimits? = builder.resourceLimits
/**
* Specifies the search strategy hyperparameter tuning uses to choose which
* hyperparameters to
* use
* for each iteration. Currently, the only valid value is
* Bayesian.
*/
val strategy: aws.sdk.kotlin.services.sagemaker.model.HyperParameterTuningJobStrategyType? = builder.strategy
/**
* The TrainingJobStatusCounters object that specifies the numbers of
* training jobs, categorized by status, that this tuning job launched.
*/
val trainingJobStatusCounters: aws.sdk.kotlin.services.sagemaker.model.TrainingJobStatusCounters? = builder.trainingJobStatusCounters
companion object {
operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.sagemaker.model.HyperParameterTuningJobSummary = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("HyperParameterTuningJobSummary(")
append("creationTime=$creationTime,")
append("hyperParameterTuningEndTime=$hyperParameterTuningEndTime,")
append("hyperParameterTuningJobArn=$hyperParameterTuningJobArn,")
append("hyperParameterTuningJobName=$hyperParameterTuningJobName,")
append("hyperParameterTuningJobStatus=$hyperParameterTuningJobStatus,")
append("lastModifiedTime=$lastModifiedTime,")
append("objectiveStatusCounters=$objectiveStatusCounters,")
append("resourceLimits=$resourceLimits,")
append("strategy=$strategy,")
append("trainingJobStatusCounters=$trainingJobStatusCounters)")
}
override fun hashCode(): kotlin.Int {
var result = creationTime?.hashCode() ?: 0
result = 31 * result + (hyperParameterTuningEndTime?.hashCode() ?: 0)
result = 31 * result + (hyperParameterTuningJobArn?.hashCode() ?: 0)
result = 31 * result + (hyperParameterTuningJobName?.hashCode() ?: 0)
result = 31 * result + (hyperParameterTuningJobStatus?.hashCode() ?: 0)
result = 31 * result + (lastModifiedTime?.hashCode() ?: 0)
result = 31 * result + (objectiveStatusCounters?.hashCode() ?: 0)
result = 31 * result + (resourceLimits?.hashCode() ?: 0)
result = 31 * result + (strategy?.hashCode() ?: 0)
result = 31 * result + (trainingJobStatusCounters?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as HyperParameterTuningJobSummary
if (creationTime != other.creationTime) return false
if (hyperParameterTuningEndTime != other.hyperParameterTuningEndTime) return false
if (hyperParameterTuningJobArn != other.hyperParameterTuningJobArn) return false
if (hyperParameterTuningJobName != other.hyperParameterTuningJobName) return false
if (hyperParameterTuningJobStatus != other.hyperParameterTuningJobStatus) return false
if (lastModifiedTime != other.lastModifiedTime) return false
if (objectiveStatusCounters != other.objectiveStatusCounters) return false
if (resourceLimits != other.resourceLimits) return false
if (strategy != other.strategy) return false
if (trainingJobStatusCounters != other.trainingJobStatusCounters) return false
return true
}
inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.sagemaker.model.HyperParameterTuningJobSummary = Builder(this).apply(block).build()
class Builder {
/**
* The date and time that the tuning job was created.
*/
var creationTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The date and time that the tuning job ended.
*/
var hyperParameterTuningEndTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The
* Amazon
* Resource Name (ARN) of the tuning job.
*/
var hyperParameterTuningJobArn: kotlin.String? = null
/**
* The name of the tuning job.
*/
var hyperParameterTuningJobName: kotlin.String? = null
/**
* The status of the
* tuning
* job.
*/
var hyperParameterTuningJobStatus: aws.sdk.kotlin.services.sagemaker.model.HyperParameterTuningJobStatus? = null
/**
* The date and time that the tuning job was
* modified.
*/
var lastModifiedTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The ObjectiveStatusCounters object that specifies the numbers of
* training jobs, categorized by objective metric status, that this tuning job
* launched.
*/
var objectiveStatusCounters: aws.sdk.kotlin.services.sagemaker.model.ObjectiveStatusCounters? = null
/**
* The ResourceLimits object that specifies the maximum number of
* training jobs and parallel training jobs allowed for this tuning job.
*/
var resourceLimits: aws.sdk.kotlin.services.sagemaker.model.ResourceLimits? = null
/**
* Specifies the search strategy hyperparameter tuning uses to choose which
* hyperparameters to
* use
* for each iteration. Currently, the only valid value is
* Bayesian.
*/
var strategy: aws.sdk.kotlin.services.sagemaker.model.HyperParameterTuningJobStrategyType? = null
/**
* The TrainingJobStatusCounters object that specifies the numbers of
* training jobs, categorized by status, that this tuning job launched.
*/
var trainingJobStatusCounters: aws.sdk.kotlin.services.sagemaker.model.TrainingJobStatusCounters? = null
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.sagemaker.model.HyperParameterTuningJobSummary) : this() {
this.creationTime = x.creationTime
this.hyperParameterTuningEndTime = x.hyperParameterTuningEndTime
this.hyperParameterTuningJobArn = x.hyperParameterTuningJobArn
this.hyperParameterTuningJobName = x.hyperParameterTuningJobName
this.hyperParameterTuningJobStatus = x.hyperParameterTuningJobStatus
this.lastModifiedTime = x.lastModifiedTime
this.objectiveStatusCounters = x.objectiveStatusCounters
this.resourceLimits = x.resourceLimits
this.strategy = x.strategy
this.trainingJobStatusCounters = x.trainingJobStatusCounters
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.sagemaker.model.HyperParameterTuningJobSummary = HyperParameterTuningJobSummary(this)
/**
* construct an [aws.sdk.kotlin.services.sagemaker.model.ObjectiveStatusCounters] inside the given [block]
*/
fun objectiveStatusCounters(block: aws.sdk.kotlin.services.sagemaker.model.ObjectiveStatusCounters.Builder.() -> kotlin.Unit) {
this.objectiveStatusCounters = aws.sdk.kotlin.services.sagemaker.model.ObjectiveStatusCounters.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.sagemaker.model.ResourceLimits] inside the given [block]
*/
fun resourceLimits(block: aws.sdk.kotlin.services.sagemaker.model.ResourceLimits.Builder.() -> kotlin.Unit) {
this.resourceLimits = aws.sdk.kotlin.services.sagemaker.model.ResourceLimits.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.sagemaker.model.TrainingJobStatusCounters] inside the given [block]
*/
fun trainingJobStatusCounters(block: aws.sdk.kotlin.services.sagemaker.model.TrainingJobStatusCounters.Builder.() -> kotlin.Unit) {
this.trainingJobStatusCounters = aws.sdk.kotlin.services.sagemaker.model.TrainingJobStatusCounters.invoke(block)
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy