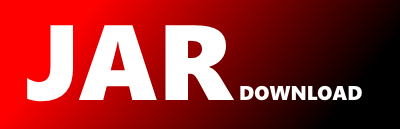
aws.sdk.kotlin.services.sagemaker.model.MonitoringClusterConfig.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.sagemaker.model
/**
* Configuration for the cluster used to run model monitoring jobs.
*/
class MonitoringClusterConfig private constructor(builder: Builder) {
/**
* The number of ML compute instances to use in the model monitoring job. For distributed
* processing jobs, specify a value greater than 1. The default value is 1.
*/
val instanceCount: kotlin.Int? = builder.instanceCount
/**
* The ML compute instance type for the processing job.
*/
val instanceType: aws.sdk.kotlin.services.sagemaker.model.ProcessingInstanceType? = builder.instanceType
/**
* The Amazon Web Services Key Management Service (Amazon Web Services KMS) key that Amazon SageMaker uses to encrypt data
* on the storage volume attached to the ML compute instance(s) that run the model monitoring
* job.
*/
val volumeKmsKeyId: kotlin.String? = builder.volumeKmsKeyId
/**
* The size of the ML storage volume, in gigabytes, that you want to provision. You must
* specify sufficient ML storage for your scenario.
*/
val volumeSizeInGb: kotlin.Int? = builder.volumeSizeInGb
companion object {
operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.sagemaker.model.MonitoringClusterConfig = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("MonitoringClusterConfig(")
append("instanceCount=$instanceCount,")
append("instanceType=$instanceType,")
append("volumeKmsKeyId=$volumeKmsKeyId,")
append("volumeSizeInGb=$volumeSizeInGb)")
}
override fun hashCode(): kotlin.Int {
var result = instanceCount ?: 0
result = 31 * result + (instanceType?.hashCode() ?: 0)
result = 31 * result + (volumeKmsKeyId?.hashCode() ?: 0)
result = 31 * result + (volumeSizeInGb ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as MonitoringClusterConfig
if (instanceCount != other.instanceCount) return false
if (instanceType != other.instanceType) return false
if (volumeKmsKeyId != other.volumeKmsKeyId) return false
if (volumeSizeInGb != other.volumeSizeInGb) return false
return true
}
inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.sagemaker.model.MonitoringClusterConfig = Builder(this).apply(block).build()
class Builder {
/**
* The number of ML compute instances to use in the model monitoring job. For distributed
* processing jobs, specify a value greater than 1. The default value is 1.
*/
var instanceCount: kotlin.Int? = null
/**
* The ML compute instance type for the processing job.
*/
var instanceType: aws.sdk.kotlin.services.sagemaker.model.ProcessingInstanceType? = null
/**
* The Amazon Web Services Key Management Service (Amazon Web Services KMS) key that Amazon SageMaker uses to encrypt data
* on the storage volume attached to the ML compute instance(s) that run the model monitoring
* job.
*/
var volumeKmsKeyId: kotlin.String? = null
/**
* The size of the ML storage volume, in gigabytes, that you want to provision. You must
* specify sufficient ML storage for your scenario.
*/
var volumeSizeInGb: kotlin.Int? = null
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.sagemaker.model.MonitoringClusterConfig) : this() {
this.instanceCount = x.instanceCount
this.instanceType = x.instanceType
this.volumeKmsKeyId = x.volumeKmsKeyId
this.volumeSizeInGb = x.volumeSizeInGb
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.sagemaker.model.MonitoringClusterConfig = MonitoringClusterConfig(this)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy