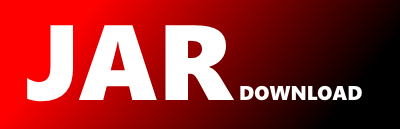
aws.sdk.kotlin.services.sagemaker.model.PipelineExecution.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.sagemaker.model
import aws.smithy.kotlin.runtime.time.Instant
/**
* An execution of a pipeline.
*/
class PipelineExecution private constructor(builder: Builder) {
/**
* Information about the user who created or modified an experiment, trial, trial
* component, lineage group, or project.
*/
val createdBy: aws.sdk.kotlin.services.sagemaker.model.UserContext? = builder.createdBy
/**
* The creation time of the pipeline execution.
*/
val creationTime: aws.smithy.kotlin.runtime.time.Instant? = builder.creationTime
/**
* If the execution failed, a message describing why.
*/
val failureReason: kotlin.String? = builder.failureReason
/**
* Information about the user who created or modified an experiment, trial, trial
* component, lineage group, or project.
*/
val lastModifiedBy: aws.sdk.kotlin.services.sagemaker.model.UserContext? = builder.lastModifiedBy
/**
* The time that the pipeline execution was last modified.
*/
val lastModifiedTime: aws.smithy.kotlin.runtime.time.Instant? = builder.lastModifiedTime
/**
* The parallelism configuration applied to the pipeline execution.
*/
val parallelismConfiguration: aws.sdk.kotlin.services.sagemaker.model.ParallelismConfiguration? = builder.parallelismConfiguration
/**
* The Amazon Resource Name (ARN) of the pipeline that was executed.
*/
val pipelineArn: kotlin.String? = builder.pipelineArn
/**
* The Amazon Resource Name (ARN) of the pipeline execution.
*/
val pipelineExecutionArn: kotlin.String? = builder.pipelineExecutionArn
/**
* The description of the pipeline execution.
*/
val pipelineExecutionDescription: kotlin.String? = builder.pipelineExecutionDescription
/**
* The display name of the pipeline execution.
*/
val pipelineExecutionDisplayName: kotlin.String? = builder.pipelineExecutionDisplayName
/**
* The status of the pipeline status.
*/
val pipelineExecutionStatus: aws.sdk.kotlin.services.sagemaker.model.PipelineExecutionStatus? = builder.pipelineExecutionStatus
/**
* Specifies the names of the experiment and trial created by a pipeline.
*/
val pipelineExperimentConfig: aws.sdk.kotlin.services.sagemaker.model.PipelineExperimentConfig? = builder.pipelineExperimentConfig
/**
* Contains a list of pipeline parameters. This list can be empty.
*/
val pipelineParameters: List? = builder.pipelineParameters
companion object {
operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.sagemaker.model.PipelineExecution = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("PipelineExecution(")
append("createdBy=$createdBy,")
append("creationTime=$creationTime,")
append("failureReason=$failureReason,")
append("lastModifiedBy=$lastModifiedBy,")
append("lastModifiedTime=$lastModifiedTime,")
append("parallelismConfiguration=$parallelismConfiguration,")
append("pipelineArn=$pipelineArn,")
append("pipelineExecutionArn=$pipelineExecutionArn,")
append("pipelineExecutionDescription=$pipelineExecutionDescription,")
append("pipelineExecutionDisplayName=$pipelineExecutionDisplayName,")
append("pipelineExecutionStatus=$pipelineExecutionStatus,")
append("pipelineExperimentConfig=$pipelineExperimentConfig,")
append("pipelineParameters=$pipelineParameters)")
}
override fun hashCode(): kotlin.Int {
var result = createdBy?.hashCode() ?: 0
result = 31 * result + (creationTime?.hashCode() ?: 0)
result = 31 * result + (failureReason?.hashCode() ?: 0)
result = 31 * result + (lastModifiedBy?.hashCode() ?: 0)
result = 31 * result + (lastModifiedTime?.hashCode() ?: 0)
result = 31 * result + (parallelismConfiguration?.hashCode() ?: 0)
result = 31 * result + (pipelineArn?.hashCode() ?: 0)
result = 31 * result + (pipelineExecutionArn?.hashCode() ?: 0)
result = 31 * result + (pipelineExecutionDescription?.hashCode() ?: 0)
result = 31 * result + (pipelineExecutionDisplayName?.hashCode() ?: 0)
result = 31 * result + (pipelineExecutionStatus?.hashCode() ?: 0)
result = 31 * result + (pipelineExperimentConfig?.hashCode() ?: 0)
result = 31 * result + (pipelineParameters?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as PipelineExecution
if (createdBy != other.createdBy) return false
if (creationTime != other.creationTime) return false
if (failureReason != other.failureReason) return false
if (lastModifiedBy != other.lastModifiedBy) return false
if (lastModifiedTime != other.lastModifiedTime) return false
if (parallelismConfiguration != other.parallelismConfiguration) return false
if (pipelineArn != other.pipelineArn) return false
if (pipelineExecutionArn != other.pipelineExecutionArn) return false
if (pipelineExecutionDescription != other.pipelineExecutionDescription) return false
if (pipelineExecutionDisplayName != other.pipelineExecutionDisplayName) return false
if (pipelineExecutionStatus != other.pipelineExecutionStatus) return false
if (pipelineExperimentConfig != other.pipelineExperimentConfig) return false
if (pipelineParameters != other.pipelineParameters) return false
return true
}
inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.sagemaker.model.PipelineExecution = Builder(this).apply(block).build()
class Builder {
/**
* Information about the user who created or modified an experiment, trial, trial
* component, lineage group, or project.
*/
var createdBy: aws.sdk.kotlin.services.sagemaker.model.UserContext? = null
/**
* The creation time of the pipeline execution.
*/
var creationTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* If the execution failed, a message describing why.
*/
var failureReason: kotlin.String? = null
/**
* Information about the user who created or modified an experiment, trial, trial
* component, lineage group, or project.
*/
var lastModifiedBy: aws.sdk.kotlin.services.sagemaker.model.UserContext? = null
/**
* The time that the pipeline execution was last modified.
*/
var lastModifiedTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The parallelism configuration applied to the pipeline execution.
*/
var parallelismConfiguration: aws.sdk.kotlin.services.sagemaker.model.ParallelismConfiguration? = null
/**
* The Amazon Resource Name (ARN) of the pipeline that was executed.
*/
var pipelineArn: kotlin.String? = null
/**
* The Amazon Resource Name (ARN) of the pipeline execution.
*/
var pipelineExecutionArn: kotlin.String? = null
/**
* The description of the pipeline execution.
*/
var pipelineExecutionDescription: kotlin.String? = null
/**
* The display name of the pipeline execution.
*/
var pipelineExecutionDisplayName: kotlin.String? = null
/**
* The status of the pipeline status.
*/
var pipelineExecutionStatus: aws.sdk.kotlin.services.sagemaker.model.PipelineExecutionStatus? = null
/**
* Specifies the names of the experiment and trial created by a pipeline.
*/
var pipelineExperimentConfig: aws.sdk.kotlin.services.sagemaker.model.PipelineExperimentConfig? = null
/**
* Contains a list of pipeline parameters. This list can be empty.
*/
var pipelineParameters: List? = null
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.sagemaker.model.PipelineExecution) : this() {
this.createdBy = x.createdBy
this.creationTime = x.creationTime
this.failureReason = x.failureReason
this.lastModifiedBy = x.lastModifiedBy
this.lastModifiedTime = x.lastModifiedTime
this.parallelismConfiguration = x.parallelismConfiguration
this.pipelineArn = x.pipelineArn
this.pipelineExecutionArn = x.pipelineExecutionArn
this.pipelineExecutionDescription = x.pipelineExecutionDescription
this.pipelineExecutionDisplayName = x.pipelineExecutionDisplayName
this.pipelineExecutionStatus = x.pipelineExecutionStatus
this.pipelineExperimentConfig = x.pipelineExperimentConfig
this.pipelineParameters = x.pipelineParameters
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.sagemaker.model.PipelineExecution = PipelineExecution(this)
/**
* construct an [aws.sdk.kotlin.services.sagemaker.model.UserContext] inside the given [block]
*/
fun createdBy(block: aws.sdk.kotlin.services.sagemaker.model.UserContext.Builder.() -> kotlin.Unit) {
this.createdBy = aws.sdk.kotlin.services.sagemaker.model.UserContext.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.sagemaker.model.UserContext] inside the given [block]
*/
fun lastModifiedBy(block: aws.sdk.kotlin.services.sagemaker.model.UserContext.Builder.() -> kotlin.Unit) {
this.lastModifiedBy = aws.sdk.kotlin.services.sagemaker.model.UserContext.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.sagemaker.model.ParallelismConfiguration] inside the given [block]
*/
fun parallelismConfiguration(block: aws.sdk.kotlin.services.sagemaker.model.ParallelismConfiguration.Builder.() -> kotlin.Unit) {
this.parallelismConfiguration = aws.sdk.kotlin.services.sagemaker.model.ParallelismConfiguration.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.sagemaker.model.PipelineExperimentConfig] inside the given [block]
*/
fun pipelineExperimentConfig(block: aws.sdk.kotlin.services.sagemaker.model.PipelineExperimentConfig.Builder.() -> kotlin.Unit) {
this.pipelineExperimentConfig = aws.sdk.kotlin.services.sagemaker.model.PipelineExperimentConfig.invoke(block)
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy