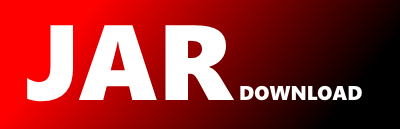
aws.sdk.kotlin.services.sagemaker.model.ProcessingS3Output.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.sagemaker.model
/**
* Configuration for uploading output data to Amazon S3 from the processing container.
*/
class ProcessingS3Output private constructor(builder: Builder) {
/**
* The local path of a directory where you want Amazon SageMaker to upload its contents to Amazon S3.
* LocalPath is an absolute path to a directory containing output files.
* This directory will be created by the platform and exist when your container's
* entrypoint is invoked.
*/
val localPath: kotlin.String? = builder.localPath
/**
* Whether to upload the results of the processing job continuously or after the job
* completes.
*/
val s3UploadMode: aws.sdk.kotlin.services.sagemaker.model.ProcessingS3UploadMode? = builder.s3UploadMode
/**
* A URI that identifies the Amazon S3 bucket where you want Amazon SageMaker to save the results of
* a processing job.
*/
val s3Uri: kotlin.String? = builder.s3Uri
companion object {
operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.sagemaker.model.ProcessingS3Output = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("ProcessingS3Output(")
append("localPath=$localPath,")
append("s3UploadMode=$s3UploadMode,")
append("s3Uri=$s3Uri)")
}
override fun hashCode(): kotlin.Int {
var result = localPath?.hashCode() ?: 0
result = 31 * result + (s3UploadMode?.hashCode() ?: 0)
result = 31 * result + (s3Uri?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as ProcessingS3Output
if (localPath != other.localPath) return false
if (s3UploadMode != other.s3UploadMode) return false
if (s3Uri != other.s3Uri) return false
return true
}
inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.sagemaker.model.ProcessingS3Output = Builder(this).apply(block).build()
class Builder {
/**
* The local path of a directory where you want Amazon SageMaker to upload its contents to Amazon S3.
* LocalPath is an absolute path to a directory containing output files.
* This directory will be created by the platform and exist when your container's
* entrypoint is invoked.
*/
var localPath: kotlin.String? = null
/**
* Whether to upload the results of the processing job continuously or after the job
* completes.
*/
var s3UploadMode: aws.sdk.kotlin.services.sagemaker.model.ProcessingS3UploadMode? = null
/**
* A URI that identifies the Amazon S3 bucket where you want Amazon SageMaker to save the results of
* a processing job.
*/
var s3Uri: kotlin.String? = null
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.sagemaker.model.ProcessingS3Output) : this() {
this.localPath = x.localPath
this.s3UploadMode = x.s3UploadMode
this.s3Uri = x.s3Uri
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.sagemaker.model.ProcessingS3Output = ProcessingS3Output(this)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy