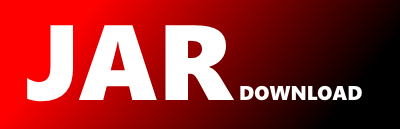
aws.sdk.kotlin.services.sagemaker.model.QualityCheckStepMetadata.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.sagemaker.model
/**
* Container for the metadata for a Quality check step. For more information, see
* the topic on QualityCheck step in the Amazon SageMaker Developer Guide.
*/
class QualityCheckStepMetadata private constructor(builder: Builder) {
/**
* The Amazon S3 URI of the baseline constraints file used for the drift check.
*/
val baselineUsedForDriftCheckConstraints: kotlin.String? = builder.baselineUsedForDriftCheckConstraints
/**
* The Amazon S3 URI of the baseline statistics file used for the drift check.
*/
val baselineUsedForDriftCheckStatistics: kotlin.String? = builder.baselineUsedForDriftCheckStatistics
/**
* The Amazon S3 URI of the newly calculated baseline constraints file.
*/
val calculatedBaselineConstraints: kotlin.String? = builder.calculatedBaselineConstraints
/**
* The Amazon S3 URI of the newly calculated baseline statistics file.
*/
val calculatedBaselineStatistics: kotlin.String? = builder.calculatedBaselineStatistics
/**
* The Amazon Resource Name (ARN) of the Quality check processing job that was run by this step execution.
*/
val checkJobArn: kotlin.String? = builder.checkJobArn
/**
* The type of the Quality check step.
*/
val checkType: kotlin.String? = builder.checkType
/**
* The model package group name.
*/
val modelPackageGroupName: kotlin.String? = builder.modelPackageGroupName
/**
* This flag indicates if a newly calculated baseline can be accessed through step properties
* BaselineUsedForDriftCheckConstraints and BaselineUsedForDriftCheckStatistics.
* If it is set to False, the previous baseline of the configured check type must also be available.
* These can be accessed through the BaselineUsedForDriftCheckConstraints and
* BaselineUsedForDriftCheckStatistics properties.
*/
val registerNewBaseline: kotlin.Boolean = builder.registerNewBaseline
/**
* This flag indicates if the drift check against the previous baseline will be skipped or not.
* If it is set to False, the previous baseline of the configured check type must be available.
*/
val skipCheck: kotlin.Boolean = builder.skipCheck
/**
* The Amazon S3 URI of violation report if violations are detected.
*/
val violationReport: kotlin.String? = builder.violationReport
companion object {
operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.sagemaker.model.QualityCheckStepMetadata = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("QualityCheckStepMetadata(")
append("baselineUsedForDriftCheckConstraints=$baselineUsedForDriftCheckConstraints,")
append("baselineUsedForDriftCheckStatistics=$baselineUsedForDriftCheckStatistics,")
append("calculatedBaselineConstraints=$calculatedBaselineConstraints,")
append("calculatedBaselineStatistics=$calculatedBaselineStatistics,")
append("checkJobArn=$checkJobArn,")
append("checkType=$checkType,")
append("modelPackageGroupName=$modelPackageGroupName,")
append("registerNewBaseline=$registerNewBaseline,")
append("skipCheck=$skipCheck,")
append("violationReport=$violationReport)")
}
override fun hashCode(): kotlin.Int {
var result = baselineUsedForDriftCheckConstraints?.hashCode() ?: 0
result = 31 * result + (baselineUsedForDriftCheckStatistics?.hashCode() ?: 0)
result = 31 * result + (calculatedBaselineConstraints?.hashCode() ?: 0)
result = 31 * result + (calculatedBaselineStatistics?.hashCode() ?: 0)
result = 31 * result + (checkJobArn?.hashCode() ?: 0)
result = 31 * result + (checkType?.hashCode() ?: 0)
result = 31 * result + (modelPackageGroupName?.hashCode() ?: 0)
result = 31 * result + (registerNewBaseline.hashCode())
result = 31 * result + (skipCheck.hashCode())
result = 31 * result + (violationReport?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as QualityCheckStepMetadata
if (baselineUsedForDriftCheckConstraints != other.baselineUsedForDriftCheckConstraints) return false
if (baselineUsedForDriftCheckStatistics != other.baselineUsedForDriftCheckStatistics) return false
if (calculatedBaselineConstraints != other.calculatedBaselineConstraints) return false
if (calculatedBaselineStatistics != other.calculatedBaselineStatistics) return false
if (checkJobArn != other.checkJobArn) return false
if (checkType != other.checkType) return false
if (modelPackageGroupName != other.modelPackageGroupName) return false
if (registerNewBaseline != other.registerNewBaseline) return false
if (skipCheck != other.skipCheck) return false
if (violationReport != other.violationReport) return false
return true
}
inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.sagemaker.model.QualityCheckStepMetadata = Builder(this).apply(block).build()
class Builder {
/**
* The Amazon S3 URI of the baseline constraints file used for the drift check.
*/
var baselineUsedForDriftCheckConstraints: kotlin.String? = null
/**
* The Amazon S3 URI of the baseline statistics file used for the drift check.
*/
var baselineUsedForDriftCheckStatistics: kotlin.String? = null
/**
* The Amazon S3 URI of the newly calculated baseline constraints file.
*/
var calculatedBaselineConstraints: kotlin.String? = null
/**
* The Amazon S3 URI of the newly calculated baseline statistics file.
*/
var calculatedBaselineStatistics: kotlin.String? = null
/**
* The Amazon Resource Name (ARN) of the Quality check processing job that was run by this step execution.
*/
var checkJobArn: kotlin.String? = null
/**
* The type of the Quality check step.
*/
var checkType: kotlin.String? = null
/**
* The model package group name.
*/
var modelPackageGroupName: kotlin.String? = null
/**
* This flag indicates if a newly calculated baseline can be accessed through step properties
* BaselineUsedForDriftCheckConstraints and BaselineUsedForDriftCheckStatistics.
* If it is set to False, the previous baseline of the configured check type must also be available.
* These can be accessed through the BaselineUsedForDriftCheckConstraints and
* BaselineUsedForDriftCheckStatistics properties.
*/
var registerNewBaseline: kotlin.Boolean = false
/**
* This flag indicates if the drift check against the previous baseline will be skipped or not.
* If it is set to False, the previous baseline of the configured check type must be available.
*/
var skipCheck: kotlin.Boolean = false
/**
* The Amazon S3 URI of violation report if violations are detected.
*/
var violationReport: kotlin.String? = null
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.sagemaker.model.QualityCheckStepMetadata) : this() {
this.baselineUsedForDriftCheckConstraints = x.baselineUsedForDriftCheckConstraints
this.baselineUsedForDriftCheckStatistics = x.baselineUsedForDriftCheckStatistics
this.calculatedBaselineConstraints = x.calculatedBaselineConstraints
this.calculatedBaselineStatistics = x.calculatedBaselineStatistics
this.checkJobArn = x.checkJobArn
this.checkType = x.checkType
this.modelPackageGroupName = x.modelPackageGroupName
this.registerNewBaseline = x.registerNewBaseline
this.skipCheck = x.skipCheck
this.violationReport = x.violationReport
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.sagemaker.model.QualityCheckStepMetadata = QualityCheckStepMetadata(this)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy