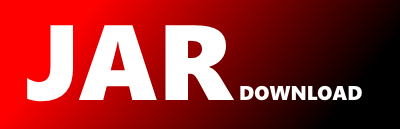
aws.sdk.kotlin.services.sagemaker.model.ResourceConfig.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.sagemaker.model
/**
* Describes the resources, including ML compute instances and ML storage volumes, to
* use for model training.
*/
class ResourceConfig private constructor(builder: Builder) {
/**
* The number of ML compute instances to use. For distributed training, provide a
* value greater than 1.
*/
val instanceCount: kotlin.Int = builder.instanceCount
/**
* The ML compute instance type.
*/
val instanceType: aws.sdk.kotlin.services.sagemaker.model.TrainingInstanceType? = builder.instanceType
/**
* The Amazon Web Services KMS key that Amazon SageMaker uses to encrypt data on the storage volume attached to the ML
* compute instance(s) that run the training job.
* Certain Nitro-based instances include local storage, dependent on the instance
* type. Local storage volumes are encrypted using a hardware module on the instance.
* You can't request a VolumeKmsKeyId when using an instance type with
* local storage.
* For a list of instance types that support local instance storage, see Instance Store Volumes.
* For more information about local instance storage encryption, see SSD
* Instance Store Volumes.
* The VolumeKmsKeyId can be in any of the following formats:
* // KMS Key ID
* "1234abcd-12ab-34cd-56ef-1234567890ab"
* // Amazon Resource Name (ARN) of a KMS Key
* "arn:aws:kms:us-west-2:111122223333:key/1234abcd-12ab-34cd-56ef-1234567890ab"
*/
val volumeKmsKeyId: kotlin.String? = builder.volumeKmsKeyId
/**
* The size of the ML storage volume that you want to provision.
* ML storage volumes store model artifacts and incremental states. Training
* algorithms might also use the ML storage volume for scratch space. If you want to store
* the training data in the ML storage volume, choose File as the
* TrainingInputMode in the algorithm specification.
* You must specify sufficient ML storage for your scenario.
* Amazon SageMaker supports only the General Purpose SSD (gp2) ML storage volume type.
* Certain Nitro-based instances include local storage with a fixed total size,
* dependent on the instance type. When using these instances for training, Amazon SageMaker mounts
* the local instance storage instead of Amazon EBS gp2 storage. You can't request a
* VolumeSizeInGB greater than the total size of the local instance
* storage.
* For a list of instance types that support local instance storage, including the
* total size per instance type, see Instance Store Volumes.
*/
val volumeSizeInGb: kotlin.Int = builder.volumeSizeInGb
companion object {
operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.sagemaker.model.ResourceConfig = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("ResourceConfig(")
append("instanceCount=$instanceCount,")
append("instanceType=$instanceType,")
append("volumeKmsKeyId=$volumeKmsKeyId,")
append("volumeSizeInGb=$volumeSizeInGb)")
}
override fun hashCode(): kotlin.Int {
var result = instanceCount
result = 31 * result + (instanceType?.hashCode() ?: 0)
result = 31 * result + (volumeKmsKeyId?.hashCode() ?: 0)
result = 31 * result + (volumeSizeInGb)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as ResourceConfig
if (instanceCount != other.instanceCount) return false
if (instanceType != other.instanceType) return false
if (volumeKmsKeyId != other.volumeKmsKeyId) return false
if (volumeSizeInGb != other.volumeSizeInGb) return false
return true
}
inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.sagemaker.model.ResourceConfig = Builder(this).apply(block).build()
class Builder {
/**
* The number of ML compute instances to use. For distributed training, provide a
* value greater than 1.
*/
var instanceCount: kotlin.Int = 0
/**
* The ML compute instance type.
*/
var instanceType: aws.sdk.kotlin.services.sagemaker.model.TrainingInstanceType? = null
/**
* The Amazon Web Services KMS key that Amazon SageMaker uses to encrypt data on the storage volume attached to the ML
* compute instance(s) that run the training job.
* Certain Nitro-based instances include local storage, dependent on the instance
* type. Local storage volumes are encrypted using a hardware module on the instance.
* You can't request a VolumeKmsKeyId when using an instance type with
* local storage.
* For a list of instance types that support local instance storage, see Instance Store Volumes.
* For more information about local instance storage encryption, see SSD
* Instance Store Volumes.
* The VolumeKmsKeyId can be in any of the following formats:
* // KMS Key ID
* "1234abcd-12ab-34cd-56ef-1234567890ab"
* // Amazon Resource Name (ARN) of a KMS Key
* "arn:aws:kms:us-west-2:111122223333:key/1234abcd-12ab-34cd-56ef-1234567890ab"
*/
var volumeKmsKeyId: kotlin.String? = null
/**
* The size of the ML storage volume that you want to provision.
* ML storage volumes store model artifacts and incremental states. Training
* algorithms might also use the ML storage volume for scratch space. If you want to store
* the training data in the ML storage volume, choose File as the
* TrainingInputMode in the algorithm specification.
* You must specify sufficient ML storage for your scenario.
* Amazon SageMaker supports only the General Purpose SSD (gp2) ML storage volume type.
* Certain Nitro-based instances include local storage with a fixed total size,
* dependent on the instance type. When using these instances for training, Amazon SageMaker mounts
* the local instance storage instead of Amazon EBS gp2 storage. You can't request a
* VolumeSizeInGB greater than the total size of the local instance
* storage.
* For a list of instance types that support local instance storage, including the
* total size per instance type, see Instance Store Volumes.
*/
var volumeSizeInGb: kotlin.Int = 0
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.sagemaker.model.ResourceConfig) : this() {
this.instanceCount = x.instanceCount
this.instanceType = x.instanceType
this.volumeKmsKeyId = x.volumeKmsKeyId
this.volumeSizeInGb = x.volumeSizeInGb
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.sagemaker.model.ResourceConfig = ResourceConfig(this)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy