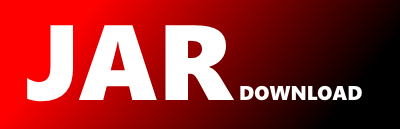
aws.sdk.kotlin.services.sagemaker.model.TrainingJob.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.sagemaker.model
import aws.smithy.kotlin.runtime.time.Instant
/**
* Contains information about a training job.
*/
class TrainingJob private constructor(builder: Builder) {
/**
* Information about the algorithm used for training, and algorithm metadata.
*/
val algorithmSpecification: aws.sdk.kotlin.services.sagemaker.model.AlgorithmSpecification? = builder.algorithmSpecification
/**
* The Amazon Resource Name (ARN) of the job.
*/
val autoMlJobArn: kotlin.String? = builder.autoMlJobArn
/**
* The billable time in seconds.
*/
val billableTimeInSeconds: kotlin.Int? = builder.billableTimeInSeconds
/**
* Contains information about the output location for managed spot training checkpoint
* data.
*/
val checkpointConfig: aws.sdk.kotlin.services.sagemaker.model.CheckpointConfig? = builder.checkpointConfig
/**
* A timestamp that indicates when the training job was created.
*/
val creationTime: aws.smithy.kotlin.runtime.time.Instant? = builder.creationTime
/**
* Configuration information for the Debugger hook parameters, metric and tensor collections, and
* storage paths. To learn more about
* how to configure the DebugHookConfig parameter,
* see Use the SageMaker and Debugger Configuration API Operations to Create, Update, and Debug Your Training Job.
*/
val debugHookConfig: aws.sdk.kotlin.services.sagemaker.model.DebugHookConfig? = builder.debugHookConfig
/**
* Information about the debug rule configuration.
*/
val debugRuleConfigurations: List? = builder.debugRuleConfigurations
/**
* Information about the evaluation status of the rules for the training job.
*/
val debugRuleEvaluationStatuses: List? = builder.debugRuleEvaluationStatuses
/**
* To encrypt all communications between ML compute instances in distributed training,
* choose True. Encryption provides greater security for distributed training,
* but training might take longer. How long it takes depends on the amount of communication
* between compute instances, especially if you use a deep learning algorithm in
* distributed training.
*/
val enableInterContainerTrafficEncryption: kotlin.Boolean = builder.enableInterContainerTrafficEncryption
/**
* When true, enables managed spot training using Amazon EC2 Spot instances to run
* training jobs instead of on-demand instances. For more information, see Managed Spot Training.
*/
val enableManagedSpotTraining: kotlin.Boolean = builder.enableManagedSpotTraining
/**
* If the TrainingJob was created with network isolation, the value is set
* to true. If network isolation is enabled, nodes can't communicate beyond
* the VPC they run in.
*/
val enableNetworkIsolation: kotlin.Boolean = builder.enableNetworkIsolation
/**
* The environment variables to set in the Docker container.
*/
val environment: Map? = builder.environment
/**
* Associates a SageMaker job as a trial component with an experiment and trial. Specified when
* you call the following APIs:
* CreateProcessingJob
* CreateTrainingJob
* CreateTransformJob
*/
val experimentConfig: aws.sdk.kotlin.services.sagemaker.model.ExperimentConfig? = builder.experimentConfig
/**
* If the training job failed, the reason it failed.
*/
val failureReason: kotlin.String? = builder.failureReason
/**
* A list of final metric values that are set when the training job completes. Used only
* if the training job was configured to use metrics.
*/
val finalMetricDataList: List? = builder.finalMetricDataList
/**
* Algorithm-specific parameters.
*/
val hyperParameters: Map? = builder.hyperParameters
/**
* An array of Channel objects that describes each data input
* channel.
*/
val inputDataConfig: List? = builder.inputDataConfig
/**
* The Amazon Resource Name (ARN) of the labeling job.
*/
val labelingJobArn: kotlin.String? = builder.labelingJobArn
/**
* A timestamp that indicates when the status of the training job was last
* modified.
*/
val lastModifiedTime: aws.smithy.kotlin.runtime.time.Instant? = builder.lastModifiedTime
/**
* Information about the Amazon S3 location that is configured for storing model
* artifacts.
*/
val modelArtifacts: aws.sdk.kotlin.services.sagemaker.model.ModelArtifacts? = builder.modelArtifacts
/**
* The S3 path where model artifacts that you configured when creating the job are
* stored. Amazon SageMaker creates subfolders for model artifacts.
*/
val outputDataConfig: aws.sdk.kotlin.services.sagemaker.model.OutputDataConfig? = builder.outputDataConfig
/**
* Resources, including ML compute instances and ML storage volumes, that are configured
* for model training.
*/
val resourceConfig: aws.sdk.kotlin.services.sagemaker.model.ResourceConfig? = builder.resourceConfig
/**
* The number of times to retry the job when the job fails due to an
* InternalServerError.
*/
val retryStrategy: aws.sdk.kotlin.services.sagemaker.model.RetryStrategy? = builder.retryStrategy
/**
* The Amazon Web Services Identity and Access Management (IAM) role configured for the training job.
*/
val roleArn: kotlin.String? = builder.roleArn
/**
* Provides detailed information about the state of the training job. For detailed
* information about the secondary status of the training job, see
* StatusMessage under SecondaryStatusTransition.
* Amazon SageMaker provides primary statuses and secondary statuses that apply to each of
* them:
* InProgress
* Starting
* - Starting the training job.
* Downloading - An optional stage for algorithms that
* support File training input mode. It indicates that
* data is being downloaded to the ML storage volumes.
* Training - Training is in progress.
* Uploading - Training is complete and the model
* artifacts are being uploaded to the S3 location.
* Completed
* Completed - The training job has completed.
* Failed
* Failed - The training job has failed. The reason for
* the failure is returned in the FailureReason field of
* DescribeTrainingJobResponse.
* Stopped
* MaxRuntimeExceeded - The job stopped because it
* exceeded the maximum allowed runtime.
* Stopped - The training job has stopped.
* Stopping
* Stopping - Stopping the training job.
* Valid values for SecondaryStatus are subject to change.
* We no longer support the following secondary statuses:
* LaunchingMLInstances
* PreparingTrainingStack
* DownloadingTrainingImage
*/
val secondaryStatus: aws.sdk.kotlin.services.sagemaker.model.SecondaryStatus? = builder.secondaryStatus
/**
* A history of all of the secondary statuses that the training job has transitioned
* through.
*/
val secondaryStatusTransitions: List? = builder.secondaryStatusTransitions
/**
* Specifies a limit to how long a model training job can run. It also specifies how long
* a managed Spot training job has to complete. When the job reaches the time limit, Amazon SageMaker
* ends the training job. Use this API to cap model training costs.
* To stop a job, Amazon SageMaker sends the algorithm the SIGTERM signal, which delays
* job termination for 120 seconds. Algorithms can use this 120-second window to save the
* model artifacts, so the results of training are not lost.
*/
val stoppingCondition: aws.sdk.kotlin.services.sagemaker.model.StoppingCondition? = builder.stoppingCondition
/**
* An array of key-value pairs. You can use tags to categorize your Amazon Web Services resources in
* different ways, for example, by purpose, owner, or environment. For more information,
* see Tagging Amazon Web Services
* Resources.
*/
val tags: List? = builder.tags
/**
* Configuration of storage locations for the Debugger TensorBoard output data.
*/
val tensorBoardOutputConfig: aws.sdk.kotlin.services.sagemaker.model.TensorBoardOutputConfig? = builder.tensorBoardOutputConfig
/**
* Indicates the time when the training job ends on training instances. You are billed
* for the time interval between the value of TrainingStartTime and this time.
* For successful jobs and stopped jobs, this is the time after model artifacts are
* uploaded. For failed jobs, this is the time when Amazon SageMaker detects a job failure.
*/
val trainingEndTime: aws.smithy.kotlin.runtime.time.Instant? = builder.trainingEndTime
/**
* The Amazon Resource Name (ARN) of the training job.
*/
val trainingJobArn: kotlin.String? = builder.trainingJobArn
/**
* The name of the training job.
*/
val trainingJobName: kotlin.String? = builder.trainingJobName
/**
* The status of the
* training
* job.
* Training job statuses are:
* InProgress - The training is in progress.
* Completed - The training job has completed.
* Failed - The training job has failed. To see the reason for the
* failure, see the FailureReason field in the response to a
* DescribeTrainingJobResponse call.
* Stopping - The training job is stopping.
* Stopped - The training job has stopped.
* For
* more detailed information, see SecondaryStatus.
*/
val trainingJobStatus: aws.sdk.kotlin.services.sagemaker.model.TrainingJobStatus? = builder.trainingJobStatus
/**
* Indicates the time when the training job starts on training instances. You are billed
* for the time interval between this time and the value of TrainingEndTime.
* The start time in CloudWatch Logs might be later than this time. The difference is due to the time
* it takes to download the training data and to the size of the training container.
*/
val trainingStartTime: aws.smithy.kotlin.runtime.time.Instant? = builder.trainingStartTime
/**
* The training time in seconds.
*/
val trainingTimeInSeconds: kotlin.Int? = builder.trainingTimeInSeconds
/**
* The Amazon Resource Name (ARN) of the associated hyperparameter tuning job if the
* training job was launched by a hyperparameter tuning job.
*/
val tuningJobArn: kotlin.String? = builder.tuningJobArn
/**
* A VpcConfig object that specifies the VPC that this training job has
* access to. For more information, see Protect Training Jobs by Using an Amazon
* Virtual Private Cloud.
*/
val vpcConfig: aws.sdk.kotlin.services.sagemaker.model.VpcConfig? = builder.vpcConfig
companion object {
operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.sagemaker.model.TrainingJob = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("TrainingJob(")
append("algorithmSpecification=$algorithmSpecification,")
append("autoMlJobArn=$autoMlJobArn,")
append("billableTimeInSeconds=$billableTimeInSeconds,")
append("checkpointConfig=$checkpointConfig,")
append("creationTime=$creationTime,")
append("debugHookConfig=$debugHookConfig,")
append("debugRuleConfigurations=$debugRuleConfigurations,")
append("debugRuleEvaluationStatuses=$debugRuleEvaluationStatuses,")
append("enableInterContainerTrafficEncryption=$enableInterContainerTrafficEncryption,")
append("enableManagedSpotTraining=$enableManagedSpotTraining,")
append("enableNetworkIsolation=$enableNetworkIsolation,")
append("environment=$environment,")
append("experimentConfig=$experimentConfig,")
append("failureReason=$failureReason,")
append("finalMetricDataList=$finalMetricDataList,")
append("hyperParameters=$hyperParameters,")
append("inputDataConfig=$inputDataConfig,")
append("labelingJobArn=$labelingJobArn,")
append("lastModifiedTime=$lastModifiedTime,")
append("modelArtifacts=$modelArtifacts,")
append("outputDataConfig=$outputDataConfig,")
append("resourceConfig=$resourceConfig,")
append("retryStrategy=$retryStrategy,")
append("roleArn=$roleArn,")
append("secondaryStatus=$secondaryStatus,")
append("secondaryStatusTransitions=$secondaryStatusTransitions,")
append("stoppingCondition=$stoppingCondition,")
append("tags=$tags,")
append("tensorBoardOutputConfig=$tensorBoardOutputConfig,")
append("trainingEndTime=$trainingEndTime,")
append("trainingJobArn=$trainingJobArn,")
append("trainingJobName=$trainingJobName,")
append("trainingJobStatus=$trainingJobStatus,")
append("trainingStartTime=$trainingStartTime,")
append("trainingTimeInSeconds=$trainingTimeInSeconds,")
append("tuningJobArn=$tuningJobArn,")
append("vpcConfig=$vpcConfig)")
}
override fun hashCode(): kotlin.Int {
var result = algorithmSpecification?.hashCode() ?: 0
result = 31 * result + (autoMlJobArn?.hashCode() ?: 0)
result = 31 * result + (billableTimeInSeconds ?: 0)
result = 31 * result + (checkpointConfig?.hashCode() ?: 0)
result = 31 * result + (creationTime?.hashCode() ?: 0)
result = 31 * result + (debugHookConfig?.hashCode() ?: 0)
result = 31 * result + (debugRuleConfigurations?.hashCode() ?: 0)
result = 31 * result + (debugRuleEvaluationStatuses?.hashCode() ?: 0)
result = 31 * result + (enableInterContainerTrafficEncryption.hashCode())
result = 31 * result + (enableManagedSpotTraining.hashCode())
result = 31 * result + (enableNetworkIsolation.hashCode())
result = 31 * result + (environment?.hashCode() ?: 0)
result = 31 * result + (experimentConfig?.hashCode() ?: 0)
result = 31 * result + (failureReason?.hashCode() ?: 0)
result = 31 * result + (finalMetricDataList?.hashCode() ?: 0)
result = 31 * result + (hyperParameters?.hashCode() ?: 0)
result = 31 * result + (inputDataConfig?.hashCode() ?: 0)
result = 31 * result + (labelingJobArn?.hashCode() ?: 0)
result = 31 * result + (lastModifiedTime?.hashCode() ?: 0)
result = 31 * result + (modelArtifacts?.hashCode() ?: 0)
result = 31 * result + (outputDataConfig?.hashCode() ?: 0)
result = 31 * result + (resourceConfig?.hashCode() ?: 0)
result = 31 * result + (retryStrategy?.hashCode() ?: 0)
result = 31 * result + (roleArn?.hashCode() ?: 0)
result = 31 * result + (secondaryStatus?.hashCode() ?: 0)
result = 31 * result + (secondaryStatusTransitions?.hashCode() ?: 0)
result = 31 * result + (stoppingCondition?.hashCode() ?: 0)
result = 31 * result + (tags?.hashCode() ?: 0)
result = 31 * result + (tensorBoardOutputConfig?.hashCode() ?: 0)
result = 31 * result + (trainingEndTime?.hashCode() ?: 0)
result = 31 * result + (trainingJobArn?.hashCode() ?: 0)
result = 31 * result + (trainingJobName?.hashCode() ?: 0)
result = 31 * result + (trainingJobStatus?.hashCode() ?: 0)
result = 31 * result + (trainingStartTime?.hashCode() ?: 0)
result = 31 * result + (trainingTimeInSeconds ?: 0)
result = 31 * result + (tuningJobArn?.hashCode() ?: 0)
result = 31 * result + (vpcConfig?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as TrainingJob
if (algorithmSpecification != other.algorithmSpecification) return false
if (autoMlJobArn != other.autoMlJobArn) return false
if (billableTimeInSeconds != other.billableTimeInSeconds) return false
if (checkpointConfig != other.checkpointConfig) return false
if (creationTime != other.creationTime) return false
if (debugHookConfig != other.debugHookConfig) return false
if (debugRuleConfigurations != other.debugRuleConfigurations) return false
if (debugRuleEvaluationStatuses != other.debugRuleEvaluationStatuses) return false
if (enableInterContainerTrafficEncryption != other.enableInterContainerTrafficEncryption) return false
if (enableManagedSpotTraining != other.enableManagedSpotTraining) return false
if (enableNetworkIsolation != other.enableNetworkIsolation) return false
if (environment != other.environment) return false
if (experimentConfig != other.experimentConfig) return false
if (failureReason != other.failureReason) return false
if (finalMetricDataList != other.finalMetricDataList) return false
if (hyperParameters != other.hyperParameters) return false
if (inputDataConfig != other.inputDataConfig) return false
if (labelingJobArn != other.labelingJobArn) return false
if (lastModifiedTime != other.lastModifiedTime) return false
if (modelArtifacts != other.modelArtifacts) return false
if (outputDataConfig != other.outputDataConfig) return false
if (resourceConfig != other.resourceConfig) return false
if (retryStrategy != other.retryStrategy) return false
if (roleArn != other.roleArn) return false
if (secondaryStatus != other.secondaryStatus) return false
if (secondaryStatusTransitions != other.secondaryStatusTransitions) return false
if (stoppingCondition != other.stoppingCondition) return false
if (tags != other.tags) return false
if (tensorBoardOutputConfig != other.tensorBoardOutputConfig) return false
if (trainingEndTime != other.trainingEndTime) return false
if (trainingJobArn != other.trainingJobArn) return false
if (trainingJobName != other.trainingJobName) return false
if (trainingJobStatus != other.trainingJobStatus) return false
if (trainingStartTime != other.trainingStartTime) return false
if (trainingTimeInSeconds != other.trainingTimeInSeconds) return false
if (tuningJobArn != other.tuningJobArn) return false
if (vpcConfig != other.vpcConfig) return false
return true
}
inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.sagemaker.model.TrainingJob = Builder(this).apply(block).build()
class Builder {
/**
* Information about the algorithm used for training, and algorithm metadata.
*/
var algorithmSpecification: aws.sdk.kotlin.services.sagemaker.model.AlgorithmSpecification? = null
/**
* The Amazon Resource Name (ARN) of the job.
*/
var autoMlJobArn: kotlin.String? = null
/**
* The billable time in seconds.
*/
var billableTimeInSeconds: kotlin.Int? = null
/**
* Contains information about the output location for managed spot training checkpoint
* data.
*/
var checkpointConfig: aws.sdk.kotlin.services.sagemaker.model.CheckpointConfig? = null
/**
* A timestamp that indicates when the training job was created.
*/
var creationTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* Configuration information for the Debugger hook parameters, metric and tensor collections, and
* storage paths. To learn more about
* how to configure the DebugHookConfig parameter,
* see Use the SageMaker and Debugger Configuration API Operations to Create, Update, and Debug Your Training Job.
*/
var debugHookConfig: aws.sdk.kotlin.services.sagemaker.model.DebugHookConfig? = null
/**
* Information about the debug rule configuration.
*/
var debugRuleConfigurations: List? = null
/**
* Information about the evaluation status of the rules for the training job.
*/
var debugRuleEvaluationStatuses: List? = null
/**
* To encrypt all communications between ML compute instances in distributed training,
* choose True. Encryption provides greater security for distributed training,
* but training might take longer. How long it takes depends on the amount of communication
* between compute instances, especially if you use a deep learning algorithm in
* distributed training.
*/
var enableInterContainerTrafficEncryption: kotlin.Boolean = false
/**
* When true, enables managed spot training using Amazon EC2 Spot instances to run
* training jobs instead of on-demand instances. For more information, see Managed Spot Training.
*/
var enableManagedSpotTraining: kotlin.Boolean = false
/**
* If the TrainingJob was created with network isolation, the value is set
* to true. If network isolation is enabled, nodes can't communicate beyond
* the VPC they run in.
*/
var enableNetworkIsolation: kotlin.Boolean = false
/**
* The environment variables to set in the Docker container.
*/
var environment: Map? = null
/**
* Associates a SageMaker job as a trial component with an experiment and trial. Specified when
* you call the following APIs:
* CreateProcessingJob
* CreateTrainingJob
* CreateTransformJob
*/
var experimentConfig: aws.sdk.kotlin.services.sagemaker.model.ExperimentConfig? = null
/**
* If the training job failed, the reason it failed.
*/
var failureReason: kotlin.String? = null
/**
* A list of final metric values that are set when the training job completes. Used only
* if the training job was configured to use metrics.
*/
var finalMetricDataList: List? = null
/**
* Algorithm-specific parameters.
*/
var hyperParameters: Map? = null
/**
* An array of Channel objects that describes each data input
* channel.
*/
var inputDataConfig: List? = null
/**
* The Amazon Resource Name (ARN) of the labeling job.
*/
var labelingJobArn: kotlin.String? = null
/**
* A timestamp that indicates when the status of the training job was last
* modified.
*/
var lastModifiedTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* Information about the Amazon S3 location that is configured for storing model
* artifacts.
*/
var modelArtifacts: aws.sdk.kotlin.services.sagemaker.model.ModelArtifacts? = null
/**
* The S3 path where model artifacts that you configured when creating the job are
* stored. Amazon SageMaker creates subfolders for model artifacts.
*/
var outputDataConfig: aws.sdk.kotlin.services.sagemaker.model.OutputDataConfig? = null
/**
* Resources, including ML compute instances and ML storage volumes, that are configured
* for model training.
*/
var resourceConfig: aws.sdk.kotlin.services.sagemaker.model.ResourceConfig? = null
/**
* The number of times to retry the job when the job fails due to an
* InternalServerError.
*/
var retryStrategy: aws.sdk.kotlin.services.sagemaker.model.RetryStrategy? = null
/**
* The Amazon Web Services Identity and Access Management (IAM) role configured for the training job.
*/
var roleArn: kotlin.String? = null
/**
* Provides detailed information about the state of the training job. For detailed
* information about the secondary status of the training job, see
* StatusMessage under SecondaryStatusTransition.
* Amazon SageMaker provides primary statuses and secondary statuses that apply to each of
* them:
* InProgress
* Starting
* - Starting the training job.
* Downloading - An optional stage for algorithms that
* support File training input mode. It indicates that
* data is being downloaded to the ML storage volumes.
* Training - Training is in progress.
* Uploading - Training is complete and the model
* artifacts are being uploaded to the S3 location.
* Completed
* Completed - The training job has completed.
* Failed
* Failed - The training job has failed. The reason for
* the failure is returned in the FailureReason field of
* DescribeTrainingJobResponse.
* Stopped
* MaxRuntimeExceeded - The job stopped because it
* exceeded the maximum allowed runtime.
* Stopped - The training job has stopped.
* Stopping
* Stopping - Stopping the training job.
* Valid values for SecondaryStatus are subject to change.
* We no longer support the following secondary statuses:
* LaunchingMLInstances
* PreparingTrainingStack
* DownloadingTrainingImage
*/
var secondaryStatus: aws.sdk.kotlin.services.sagemaker.model.SecondaryStatus? = null
/**
* A history of all of the secondary statuses that the training job has transitioned
* through.
*/
var secondaryStatusTransitions: List? = null
/**
* Specifies a limit to how long a model training job can run. It also specifies how long
* a managed Spot training job has to complete. When the job reaches the time limit, Amazon SageMaker
* ends the training job. Use this API to cap model training costs.
* To stop a job, Amazon SageMaker sends the algorithm the SIGTERM signal, which delays
* job termination for 120 seconds. Algorithms can use this 120-second window to save the
* model artifacts, so the results of training are not lost.
*/
var stoppingCondition: aws.sdk.kotlin.services.sagemaker.model.StoppingCondition? = null
/**
* An array of key-value pairs. You can use tags to categorize your Amazon Web Services resources in
* different ways, for example, by purpose, owner, or environment. For more information,
* see Tagging Amazon Web Services
* Resources.
*/
var tags: List? = null
/**
* Configuration of storage locations for the Debugger TensorBoard output data.
*/
var tensorBoardOutputConfig: aws.sdk.kotlin.services.sagemaker.model.TensorBoardOutputConfig? = null
/**
* Indicates the time when the training job ends on training instances. You are billed
* for the time interval between the value of TrainingStartTime and this time.
* For successful jobs and stopped jobs, this is the time after model artifacts are
* uploaded. For failed jobs, this is the time when Amazon SageMaker detects a job failure.
*/
var trainingEndTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The Amazon Resource Name (ARN) of the training job.
*/
var trainingJobArn: kotlin.String? = null
/**
* The name of the training job.
*/
var trainingJobName: kotlin.String? = null
/**
* The status of the
* training
* job.
* Training job statuses are:
* InProgress - The training is in progress.
* Completed - The training job has completed.
* Failed - The training job has failed. To see the reason for the
* failure, see the FailureReason field in the response to a
* DescribeTrainingJobResponse call.
* Stopping - The training job is stopping.
* Stopped - The training job has stopped.
* For
* more detailed information, see SecondaryStatus.
*/
var trainingJobStatus: aws.sdk.kotlin.services.sagemaker.model.TrainingJobStatus? = null
/**
* Indicates the time when the training job starts on training instances. You are billed
* for the time interval between this time and the value of TrainingEndTime.
* The start time in CloudWatch Logs might be later than this time. The difference is due to the time
* it takes to download the training data and to the size of the training container.
*/
var trainingStartTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The training time in seconds.
*/
var trainingTimeInSeconds: kotlin.Int? = null
/**
* The Amazon Resource Name (ARN) of the associated hyperparameter tuning job if the
* training job was launched by a hyperparameter tuning job.
*/
var tuningJobArn: kotlin.String? = null
/**
* A VpcConfig object that specifies the VPC that this training job has
* access to. For more information, see Protect Training Jobs by Using an Amazon
* Virtual Private Cloud.
*/
var vpcConfig: aws.sdk.kotlin.services.sagemaker.model.VpcConfig? = null
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.sagemaker.model.TrainingJob) : this() {
this.algorithmSpecification = x.algorithmSpecification
this.autoMlJobArn = x.autoMlJobArn
this.billableTimeInSeconds = x.billableTimeInSeconds
this.checkpointConfig = x.checkpointConfig
this.creationTime = x.creationTime
this.debugHookConfig = x.debugHookConfig
this.debugRuleConfigurations = x.debugRuleConfigurations
this.debugRuleEvaluationStatuses = x.debugRuleEvaluationStatuses
this.enableInterContainerTrafficEncryption = x.enableInterContainerTrafficEncryption
this.enableManagedSpotTraining = x.enableManagedSpotTraining
this.enableNetworkIsolation = x.enableNetworkIsolation
this.environment = x.environment
this.experimentConfig = x.experimentConfig
this.failureReason = x.failureReason
this.finalMetricDataList = x.finalMetricDataList
this.hyperParameters = x.hyperParameters
this.inputDataConfig = x.inputDataConfig
this.labelingJobArn = x.labelingJobArn
this.lastModifiedTime = x.lastModifiedTime
this.modelArtifacts = x.modelArtifacts
this.outputDataConfig = x.outputDataConfig
this.resourceConfig = x.resourceConfig
this.retryStrategy = x.retryStrategy
this.roleArn = x.roleArn
this.secondaryStatus = x.secondaryStatus
this.secondaryStatusTransitions = x.secondaryStatusTransitions
this.stoppingCondition = x.stoppingCondition
this.tags = x.tags
this.tensorBoardOutputConfig = x.tensorBoardOutputConfig
this.trainingEndTime = x.trainingEndTime
this.trainingJobArn = x.trainingJobArn
this.trainingJobName = x.trainingJobName
this.trainingJobStatus = x.trainingJobStatus
this.trainingStartTime = x.trainingStartTime
this.trainingTimeInSeconds = x.trainingTimeInSeconds
this.tuningJobArn = x.tuningJobArn
this.vpcConfig = x.vpcConfig
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.sagemaker.model.TrainingJob = TrainingJob(this)
/**
* construct an [aws.sdk.kotlin.services.sagemaker.model.AlgorithmSpecification] inside the given [block]
*/
fun algorithmSpecification(block: aws.sdk.kotlin.services.sagemaker.model.AlgorithmSpecification.Builder.() -> kotlin.Unit) {
this.algorithmSpecification = aws.sdk.kotlin.services.sagemaker.model.AlgorithmSpecification.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.sagemaker.model.CheckpointConfig] inside the given [block]
*/
fun checkpointConfig(block: aws.sdk.kotlin.services.sagemaker.model.CheckpointConfig.Builder.() -> kotlin.Unit) {
this.checkpointConfig = aws.sdk.kotlin.services.sagemaker.model.CheckpointConfig.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.sagemaker.model.DebugHookConfig] inside the given [block]
*/
fun debugHookConfig(block: aws.sdk.kotlin.services.sagemaker.model.DebugHookConfig.Builder.() -> kotlin.Unit) {
this.debugHookConfig = aws.sdk.kotlin.services.sagemaker.model.DebugHookConfig.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.sagemaker.model.ExperimentConfig] inside the given [block]
*/
fun experimentConfig(block: aws.sdk.kotlin.services.sagemaker.model.ExperimentConfig.Builder.() -> kotlin.Unit) {
this.experimentConfig = aws.sdk.kotlin.services.sagemaker.model.ExperimentConfig.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.sagemaker.model.ModelArtifacts] inside the given [block]
*/
fun modelArtifacts(block: aws.sdk.kotlin.services.sagemaker.model.ModelArtifacts.Builder.() -> kotlin.Unit) {
this.modelArtifacts = aws.sdk.kotlin.services.sagemaker.model.ModelArtifacts.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.sagemaker.model.OutputDataConfig] inside the given [block]
*/
fun outputDataConfig(block: aws.sdk.kotlin.services.sagemaker.model.OutputDataConfig.Builder.() -> kotlin.Unit) {
this.outputDataConfig = aws.sdk.kotlin.services.sagemaker.model.OutputDataConfig.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.sagemaker.model.ResourceConfig] inside the given [block]
*/
fun resourceConfig(block: aws.sdk.kotlin.services.sagemaker.model.ResourceConfig.Builder.() -> kotlin.Unit) {
this.resourceConfig = aws.sdk.kotlin.services.sagemaker.model.ResourceConfig.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.sagemaker.model.RetryStrategy] inside the given [block]
*/
fun retryStrategy(block: aws.sdk.kotlin.services.sagemaker.model.RetryStrategy.Builder.() -> kotlin.Unit) {
this.retryStrategy = aws.sdk.kotlin.services.sagemaker.model.RetryStrategy.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.sagemaker.model.StoppingCondition] inside the given [block]
*/
fun stoppingCondition(block: aws.sdk.kotlin.services.sagemaker.model.StoppingCondition.Builder.() -> kotlin.Unit) {
this.stoppingCondition = aws.sdk.kotlin.services.sagemaker.model.StoppingCondition.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.sagemaker.model.TensorBoardOutputConfig] inside the given [block]
*/
fun tensorBoardOutputConfig(block: aws.sdk.kotlin.services.sagemaker.model.TensorBoardOutputConfig.Builder.() -> kotlin.Unit) {
this.tensorBoardOutputConfig = aws.sdk.kotlin.services.sagemaker.model.TensorBoardOutputConfig.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.sagemaker.model.VpcConfig] inside the given [block]
*/
fun vpcConfig(block: aws.sdk.kotlin.services.sagemaker.model.VpcConfig.Builder.() -> kotlin.Unit) {
this.vpcConfig = aws.sdk.kotlin.services.sagemaker.model.VpcConfig.invoke(block)
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy