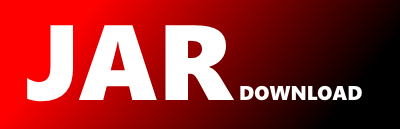
aws.sdk.kotlin.services.sagemaker.model.TransformS3DataSource.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.sagemaker.model
/**
* Describes the S3 data source.
*/
class TransformS3DataSource private constructor(builder: Builder) {
/**
* If you choose S3Prefix, S3Uri identifies a key name prefix.
* Amazon SageMaker uses all objects with the specified key name prefix for batch transform.
* If you choose ManifestFile, S3Uri identifies an object that
* is a manifest file containing a list of object keys that you want Amazon SageMaker to use for batch
* transform.
* The following values are compatible: ManifestFile,
* S3Prefix
* The following value is not compatible: AugmentedManifestFile
*/
val s3DataType: aws.sdk.kotlin.services.sagemaker.model.S3DataType? = builder.s3DataType
/**
* Depending on the value specified for the S3DataType, identifies either a
* key name prefix or a manifest. For example:
* A key name prefix might look like this:
* s3://bucketname/exampleprefix.
* A manifest might look like this:
* s3://bucketname/example.manifest
* The manifest is an S3 object which is a JSON file with the following format:
* [ {"prefix": "s3://customer_bucket/some/prefix/"},
* "relative/path/to/custdata-1",
* "relative/path/custdata-2",
* ...
* "relative/path/custdata-N"
* ]
* The preceding JSON matches the following S3Uris:
* s3://customer_bucket/some/prefix/relative/path/to/custdata-1
* s3://customer_bucket/some/prefix/relative/path/custdata-2
* ...
* s3://customer_bucket/some/prefix/relative/path/custdata-N
* The complete set of S3Uris in this manifest constitutes the
* input data for the channel for this datasource. The object that each
* S3Uris points to must be readable by the IAM role that Amazon SageMaker
* uses to perform tasks on your behalf.
*/
val s3Uri: kotlin.String? = builder.s3Uri
companion object {
operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.sagemaker.model.TransformS3DataSource = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("TransformS3DataSource(")
append("s3DataType=$s3DataType,")
append("s3Uri=$s3Uri)")
}
override fun hashCode(): kotlin.Int {
var result = s3DataType?.hashCode() ?: 0
result = 31 * result + (s3Uri?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as TransformS3DataSource
if (s3DataType != other.s3DataType) return false
if (s3Uri != other.s3Uri) return false
return true
}
inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.sagemaker.model.TransformS3DataSource = Builder(this).apply(block).build()
class Builder {
/**
* If you choose S3Prefix, S3Uri identifies a key name prefix.
* Amazon SageMaker uses all objects with the specified key name prefix for batch transform.
* If you choose ManifestFile, S3Uri identifies an object that
* is a manifest file containing a list of object keys that you want Amazon SageMaker to use for batch
* transform.
* The following values are compatible: ManifestFile,
* S3Prefix
* The following value is not compatible: AugmentedManifestFile
*/
var s3DataType: aws.sdk.kotlin.services.sagemaker.model.S3DataType? = null
/**
* Depending on the value specified for the S3DataType, identifies either a
* key name prefix or a manifest. For example:
* A key name prefix might look like this:
* s3://bucketname/exampleprefix.
* A manifest might look like this:
* s3://bucketname/example.manifest
* The manifest is an S3 object which is a JSON file with the following format:
* [ {"prefix": "s3://customer_bucket/some/prefix/"},
* "relative/path/to/custdata-1",
* "relative/path/custdata-2",
* ...
* "relative/path/custdata-N"
* ]
* The preceding JSON matches the following S3Uris:
* s3://customer_bucket/some/prefix/relative/path/to/custdata-1
* s3://customer_bucket/some/prefix/relative/path/custdata-2
* ...
* s3://customer_bucket/some/prefix/relative/path/custdata-N
* The complete set of S3Uris in this manifest constitutes the
* input data for the channel for this datasource. The object that each
* S3Uris points to must be readable by the IAM role that Amazon SageMaker
* uses to perform tasks on your behalf.
*/
var s3Uri: kotlin.String? = null
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.sagemaker.model.TransformS3DataSource) : this() {
this.s3DataType = x.s3DataType
this.s3Uri = x.s3Uri
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.sagemaker.model.TransformS3DataSource = TransformS3DataSource(this)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy